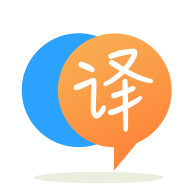
[英]I'm trying to make a function that converts integer to string which is not returning the string. How can I solve this?
[英]How can I store a digit of an Integer I'm trying to separating?
我遇到的問題是,我想取一個整數並將其分開。 例如:用戶輸入:23432。控制台應該打印“2 3 4 3 2。我遇到的問題是存儲這些數字。例如,
User Input : 2020
assign input to num.
digit = 2020 % 10 = 0 <--- 1st Digit
num = num / 10 = 202
digit2 = num % 10 = 2 <--- 2nd Digit
num = num / 100 = 20.2
temp = round(num) = 20
digit3 = num % 10 = 0 <--- 3rd Digit
digit4 = num / 10 = 2 <---- 4th Digit
這種方法的問題在於它依賴於用戶輸入,我使用的范圍是 1-32767,所以我不知道要創建多少個數字變量。 使用我創建的結構,有人可以幫助它以某種方式運行,無論數字是什么,數字都以我描述的方式保存和打印嗎?
int Rem(int num);
int Div(int num);
int main() {
int num;
printf("Enter an integer between 1 and 32767: ");
scanf("%d", &num);
Rem(num);
Div(num);
printf("%d","The digits in the number are: ");
}
int Rem(int num) {
int rem = num % 10;
return rem;
}
int Div(int num){
int div = num / 10;
return div;
}
這種方法的問題在於它依賴於用戶輸入,我使用的范圍是 1-32767,所以我不知道要創建多少個數字變量。
所以計算一下。 您可以通過每次將變量增加 10 倍,直到再增加一次使其大於輸入數字來做到這一點:
int num;
printf("Enter an integer between 1 and 32767: ");
scanf("%d", &num);
int div = 1;
while(div * 10 <= num)
div *= 10;
然后你可以重復將你的數字除以這個除數來得到每個數字,每次將除數除以 10 一次移動一個位置:
printf("The digits in the number are: ");
while(div > 0)
{
printf("%d ", (num / div) % 10);
div /= 10;
}
這是一個非常復雜的方法。 為什么不讀取字符串,並像這樣解析字符串:
int main(void) {
char buf[256]; // should be big enough, right? 10^256-1
memset(buf, 0, 256];
puts("enter something : ");
if( NULL == fgets(STDIN, 255, buf)) {
perror("Egad! Something went wrong!");
exit(1);
}
// at this point, you already have all the input in the buf variable
for(int i=0; buf[i]; i++) {
putchar( buf[i] ); // put out the number
putchar( ' ' ); // put out a space
}
putchar( '\n' ); // make a nice newline
}
如上所述,它允許任何字符,而不僅僅是數字。 如果您想限制為數字,您可以過濾輸入,或在 for 循環中進行檢查……這取決於您要完成的任務。
C 允許您非常優雅地處理問題的一種方法是通過遞歸。
考慮一個例程,它只知道如何打印數字的最后一位,如果需要,前面有一個空格。
void printWithSpaces(int neblod)
{
// Take everything except the last digit.
int mene = neblod / 10;
// Now extract the last digit
int fhtagn = neblod % 10;
// Check if there are leading digits
if (mene != 0)
{
// There are, so do some magic to deal with the leading digits
// And print the intervening space.
putchar(' ');
}
putchar(fhtagn + '0');
}
好的。 所以這很好,除了我們可以用什么來“做一些魔術來處理前導數字”?
難道我們不想將它們打印為數字序列,並帶有合適的中間空格嗎?
這不正是void printWithSpaces(int neblod)
所做的嗎?
所以我們做一個改變:
void printWithSpaces(int neblod)
{
// Take everything except the last digit.
int mene = neblod / 10;
// Now extract the last digit
int fhtagn = neblod % 10;
// Check if there are leading digits
if (mene != 0)
{
// There are, so print them out
printWithSpaces(mene);
// And print the intervening space.
putchar(' ');
}
putchar(fhtagn + '0');
}
你已經完成了。
對於好奇的人,以下關於 C 遞歸的文章可能會提供一個有趣的閱讀,以及對我稍微不尋常的變量名稱選擇的一些見解。 ;) http://www.bobhobbs.com/files/kr_lovecraft.html
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.