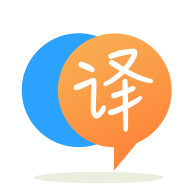
[英]VB.NET call ADODB.Command.Execute - not returning Recordset
[英]ADODB RecordSet to String() in VB.NET
我發現了這篇文章對我的項目有幫助:
我想使其適應VB.NET而不是VBA,但是它不起作用。
我的目標是使用SQL創建String()。 為了讓您了解硬編碼后的字符串的樣子,這是我們的處理方法:
Public Sub New()
InitializeComponent()
strValue = New String() {"10051", "65658", "25689" etc... }
End Sub
**'My actual code is as follows:**
Private Sub Form1_Load(ByVal sender As Object, ByVal e As EventArgs)
Handles MyBase.Load
Dim Connection1 As New ADODB.Connection
Dim Connection1 As New ADODB.Connection
Dim RecordSet1 As New ADODB.Recordset
Dim SqlQuery1 As String, ConnectionString1 As String
Dim strValue As String()
SqlQuery1 = "Select ItemCode From OITM"
ConnectionString1 = "Driver=SQL Server;Server=Myserver; Database=MyDbase;
User Id = sa; Password= 12345"
Connection1.Open(ConnectionString1)
RecordSet1 = New ADODB.Recordset
RecordSet1.Open(SqlQuery1, Connection1)
strValue = TryCast(RecordSet1.Fields("ItemCode").Value, String())
Do While RecordSet1.EOF = False
strValue = TryCast(RecordSet1.Fields("ItemCode").Value, String())
MsgBox(strValue)
RecordSet1.MoveNext()
Loop
我希望MsbBox在每次循環時都顯示ItemCode,並建立整個(很長)字符串。 但它顯示為空白。
我讀到EOF可能在VB .NET中不起作用。
我必須做什么?
我看到一些問題:
首先,您將從記錄集中獲得的值轉換為字符串數組。 為什么? 您不應該這樣做,嘗試像這樣直接設置值:
strValue = RecordSet1.Fields("ItemCode").Value
其次,您不連接字符串。 使用“ +”或“&”連接您從每一行中讀取的內容,如下所示:
Do While RecordSet1.EOF = False
strValue = strValue + RecordSet1.Fields("ItemCode").Value
MsgBox(strValue)
RecordSet1.MoveNext()
Loop
但是您需要一個滿足您需要的數組。 因此,您可以使用列表,然后使用toArray方法:
Dim strValues As String()
Dim strList As List(Of String) = New List(Of String)()
Do While RecordSet1.EOF = False
strList.Add(RecordSet1.Fields("ItemCode").Value)
RecordSet1.MoveNext()
Loop
strValues = strList.ToArray()
現在strValues是從SQL數據庫讀取的字符串數組。
我希望MsbBox在每個循環中顯示ItemCode,並建立整個(很長)字符串
那不是它的工作原理。 每次循環,您只有一項 。 代碼中沒有任何內容會累積每次迭代的值。 嘗試將其強制轉換為數組,即使它可以工作(不可行),也只會在每次迭代中為您提供一個只有該一項的新數組實例 。 再說一次,即使如此,也不會發生。 您不能只將單個項目轉換為數組。
不僅如此,.Net還使用實際的數組,而不是您現在在其他語言中看到的類似數組的集合。 .Net也有那些集合,但是它沒有試圖假裝它們是數組。 實際數組的關鍵屬性之一是固定大小 。 首次分配數組時,必須知道該數組中的元素數。 甚至ReDim Preserve
依賴於此,它可以有效地分配一個全新的數組並在每次使用它時復制所有元素。 這意味着期望將項目強制轉換為數組變量會串聯到數組真的很奇怪。 聽起來您可能想要一個List(Of String)
而不是一個數組。
如果程序的其他部分需要數組,則需要更改它們以使用List(Of String)
代替。 .Net中不再使用基本數組了,基本數組已被通用List(Of T)
類型取代。 並且盡可能使您的方法參數要求IEnumerable(Of T)
而不是T()
或List(Of T)
。 當您使用IEnumerable(Of T)
,數組和列表都可以作為參數傳遞。
另一個問題是使用帶有MsgBox()
的數組。 該消息框不夠聰明,不足以知道如何處理數組。 您最終回到了System.Object.ToString()
重載,該重載僅返回類型名稱。 因此,即使其他所有方法都起作用了(也沒有用),您最好希望看到的就是文本System.Array
。
最后, 不要在VB.Net中使用RecordSet
當向前移植代碼時,僅存在舊的ADO庫是為了向后兼容。 您不應該在新開發中使用它。 改用較新的ADO.Net API。
嘗試這個:
'At the very top of the file:
Imports System.Data.SqlClient
Imports System.Collections.Generic
Imports System.Text
Private Sub Form1_Load(ByVal sender As Object, ByVal e As EventArgs)
Handles MyBase.Load
'I know this is just a sample, but DON'T USE THE SA ACCOUNT!
Dim ConnectionString As String = "Server=Myserver;Database=MyDbase;User Id=sa;Password=12345"
Dim Sql As String = "Select ItemCode From OITM"
Dim result As New List(Of String) 'The "array" (really an array-like collection)
Dim message As New StringBuilder() 'For building up the string
Using connection As New SqlConnection(ConnectionString), _
command As New SqlCommand(sql, connection)
connection.Open()
Using reader As SqlDataReader = command.ExecuteReader()
Dim delimiter As String = ""
While reader.Read()
Dim itemCode As String = reader("ItemCode").ToString()
result.Add(itemCode)
message.Append(delimiter).Append(itemCode)
MessageBox.Show(message.ToString())
delimiter = ","
End While
End Using
End Using
End Sub
另一種選擇是創建一個數據表:
Private Sub Form1_Load(ByVal sender As Object, ByVal e As EventArgs)
Handles MyBase.Load
'I know this is just a sample, but DON'T USE THE SA ACCOUNT!
Dim ConnectionString As String = "Server=Myserver;Database=MyDbase;User Id=sa;Password=12345"
Dim Sql As String = "Select ItemCode From OITM"
Dim result As New DataSet()
Using connection As New SqlConnection(ConnectionString), _
command As New SqlCommand(sql, connection), _
adapter As New SqlDataAdapter(command)
adapter.Fill(result)
End Using
End Sub
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.