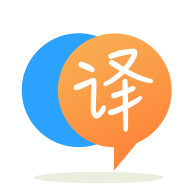
[英]Xamarin.Forms: Custom renderer for displaying disclosure indicator on UITableViewCell
[英]Adding ViewCell Disclosure Indicator Accessory to Xamarin.Forms Android and Iphone
我發現很多移動應用程序(Android&IOS)的列表視圖的最右側帶有圖標,例如公開,選中標記等。這就是viewcell渲染器。 我知道如何制作到iPhone,但不知道如何制作到Android? 贊賞有人可以與我分享如何將其應用於android。 提前致謝。 您可能會在下面看到我上傳的圖片:
我在iOS中添加一個類文件,代碼如下:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using Xamarin.Forms.Platform.iOS;
using Xamarin.Forms;
using Foundation;
using UIKit;
using CustomRenderer.iOS;
[assembly: ExportRenderer(typeof(ViewCell),
typeof(StandardViewCellRenderer))]
namespace CustomRenderer.iOS
{
class StandardViewCellRenderer : ViewCellRenderer
{
public override UIKit.UITableViewCell GetCell(Cell item,
UIKit.UITableViewCell reusableCell, UIKit.UITableView tv)
{
var cell = base.GetCell(item, reusableCell, tv);
switch (item.StyleId)
{
case "none":
cell.Accessory = UIKit.UITableViewCellAccessory.None;
break;
case "checkmark":
cell.Accessory =
UIKit.UITableViewCellAccessory.Checkmark;
break;
case "detail-button":
cell.Accessory =
UIKit.UITableViewCellAccessory.DetailButton;
break;
case "detail-disclosure-button":
cell.Accessory =
UIKit.UITableViewCellAccessory.DetailDisclosureButton;
break;
case "disclosure":
default:
cell.Accessory =
UIKit.UITableViewCellAccessory.DisclosureIndicator;
break;
}
return cell;
}
}
}
Then, in my main XAML, code as below:
<ListView x:Name="MyListView">
<ListView.ItemTemplate>
<DataTemplate>
<ViewCell StyleId="detail-disclosure-button">
<StackLayout>
<Label Text="{Binding AnimalName}"/>
</StackLayout>
</ViewCell>
</DataTemplate>
</ListView.ItemTemplate>
</ListView>
對於Android,您將需要下載圖片(沒有真正的本機方式,因為它是iOS設計功能)。
首先下載相應的圖標(例如從此處下載 )
然后,使用自定義渲染器
使用Android.Graphics; 使用Android.Graphics.Drawables; 使用Android.Views; 使用Testapp.Droid; 使用Xamarin.Forms; 使用Xamarin.Forms.Platform.Android;
[assembly: ExportRenderer(typeof(ViewCell), typeof(CustomViewCellRenderer))]
namespace Testapp.Droid
{
public class CustomViewCellRenderer : ViewCellRenderer
{
protected override Android.Views.View GetCellCore(Cell item, Android.Views.View convertView, Android.Views.ViewGroup parent, Android.Content.Context context)
{
var cell = base.GetCellCore(item, convertView, parent, context);
//switch (item.StyleId)
//{
// case "disclosure":
// break;
//}
var bmp = BitmapFactory.DecodeResource(cell.Resources, Resource.Drawable.arrow);
var bitmapDrawable = new BitmapDrawable(cell.Resources, Bitmap.CreateScaledBitmap(bmp, 50, 50, true));
bitmapDrawable.Gravity = GravityFlags.Right | GravityFlags.CenterVertical;
cell.SetBackground(bitmapDrawable);
return cell;
}
}
}
然后,此XAML:
<ListView>
<ListView.ItemsSource>
<x:Array Type="{x:Type x:String}">
<x:String>mono</x:String>
<x:String>monodroid</x:String>
<x:String>monotouch</x:String>
<x:String>monorail</x:String>
<x:String>monodevelop</x:String>
<x:String>monotone</x:String>
<x:String>monopoly</x:String>
<x:String>monomodal</x:String>
<x:String>mononucleosis</x:String>
</x:Array>
</ListView.ItemsSource>
<ListView.ItemTemplate>
<DataTemplate>
<ViewCell>
<StackLayout>
<Label Text="{Binding .}" TextColor="#f35e20" />
</StackLayout>
</ViewCell>
</DataTemplate>
</ListView.ItemTemplate>
</ListView>
結果是:
如果要在Android中執行此操作,請創建示例,可以從GitHub的ViewCell_Custom_Renderer文件夾下載以供參考。
https://github.com/WendyZang/Test.git
對於此示例,您需要首先創建一個自定義單元。
public class NativeCell : ViewCell
{
public static readonly BindableProperty NameProperty =
BindableProperty.Create("Name", typeof(string), typeof(NativeCell), "");
public string Name
{
get { return (string)GetValue(NameProperty); }
set { SetValue(NameProperty, value); }
}
public static readonly BindableProperty CategoryProperty =
BindableProperty.Create("Category", typeof(string), typeof(NativeCell), "");
public string Category
{
get { return (string)GetValue(CategoryProperty); }
set { SetValue(CategoryProperty, value); }
}
public static readonly BindableProperty ImageFilenameProperty =
BindableProperty.Create("ImageFilename", typeof(string), typeof(NativeCell), "");
public string ImageFilename
{
get { return (string)GetValue(ImageFilenameProperty); }
set { SetValue(ImageFilenameProperty, value); }
}
}
然后在Android上創建自定義渲染器。
[assembly: ExportRenderer(typeof(NativeCell), typeof(NativeAndroidCellRenderer))]
namespace CustomRenderer.Droid
{
public class NativeAndroidCellRenderer : ViewCellRenderer
{
NativeAndroidCell cell;
protected override Android.Views.View GetCellCore(Cell item, Android.Views.View convertView, ViewGroup parent, Context context)
{
var nativeCell = (NativeCell)item;
Console.WriteLine("\t\t" + nativeCell.Name);
cell = convertView as NativeAndroidCell;
if (cell == null)
{
cell = new NativeAndroidCell(context, nativeCell);
}
else
{
cell.NativeCell.PropertyChanged -= OnNativeCellPropertyChanged;
}
nativeCell.PropertyChanged += OnNativeCellPropertyChanged;
cell.UpdateCell(nativeCell);
return cell;
}
void OnNativeCellPropertyChanged(object sender, PropertyChangedEventArgs e)
{
var nativeCell = (NativeCell)sender;
if (e.PropertyName == NativeCell.NameProperty.PropertyName)
{
cell.HeadingTextView.Text = nativeCell.Name;
}
else if (e.PropertyName == NativeCell.CategoryProperty.PropertyName)
{
cell.SubheadingTextView.Text = nativeCell.Category;
}
else if (e.PropertyName == NativeCell.ImageFilenameProperty.PropertyName)
{
cell.SetImage(nativeCell.ImageFilename);
}
}
}
}
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.