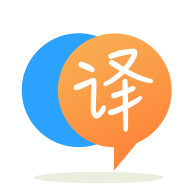
[英]LostFocus event handler is triggering when a control within the selected row is clicked
[英]Passing a dynamic value to an event handler when a row is clicked
請參見下面的代碼:
<?xml version="1.0" encoding="utf-8" ?>
<ContentPage xmlns="http://xamarin.com/schemas/2014/forms"
xmlns:x="http://schemas.microsoft.com/winfx/2009/xaml"
xmlns:local="clr-namespace:App1"
x:Class="App1.MainPage">
<ContentPage.BindingContext>
<local:PersonViewModel />
</ContentPage.BindingContext>
<StackLayout>
<ListView x:Name="listView" ItemsSource="{Binding People}">
<ListView.Header>
<Grid>
<Grid.BindingContext>
<local:PersonViewModel />
</Grid.BindingContext>
<Grid.ColumnDefinitions>
<ColumnDefinition Width="*" />
<ColumnDefinition Width="*" />
<ColumnDefinition Width="*" />
<ColumnDefinition Width="*" />
</Grid.ColumnDefinitions>
<Grid.RowDefinitions>
<RowDefinition Height="Auto" />
</Grid.RowDefinitions>
<Label Grid.Column="1" Text="Id" />
<Label Grid.Column="2" Text="First Name" />
<Label Grid.Column="3" Text="Surname" />
<Label Grid.Column="4" Text="Date Of Birth" />
</Grid>
</ListView.Header>
<ListView.ItemTemplate>
<DataTemplate>
<ViewCell>
<Grid>
<Grid.ColumnDefinitions>
<ColumnDefinition Width="*" />
<ColumnDefinition Width="*" />
<ColumnDefinition Width="*" />
<ColumnDefinition Width="*" />
</Grid.ColumnDefinitions>
<Grid.RowDefinitions>
<RowDefinition Height="Auto" />
</Grid.RowDefinitions>
<Label Grid.Column="1" Text="{Binding Id}" />
<Label Grid.Column="2" Text="{Binding FirstName}" />
<Label Grid.Column="3" Text="{Binding Surname}" />
<Label Grid.Column="4" Text="{Binding Age}" />
</Grid>
</ViewCell>
</DataTemplate>
</ListView.ItemTemplate>
</ListView>
</StackLayout>
</ContentPage>
和下面的視圖模型:
public class PersonViewModel
{
private ObservableCollection<Person> _people = new ObservableCollection<Person>();
public ObservableCollection<Person> People
{
get { return _people; }
}
public PersonViewModel()
{
_people.Add(new Person { Id = Guid.NewGuid().ToString(), Age = 36, FirstName = "Andrew", Surname = "Smith" });
_people.Add(new Person { Id = Guid.NewGuid().ToString(), Age = 65, FirstName = "David", Surname = "White" });
_people.Add(new Person { Id = Guid.NewGuid().ToString(), Age = 39, FirstName = "Bert", Surname = "Edwards" });
}
}
它按預期工作,即將網格添加到頁面。 如果用戶單擊其中一行; 我希望將該行的ID傳遞給事件處理程序。 我該怎么做呢。 我花了最后三個小時來研究這個問題,但是沒有找到答案。 我找到的最接近的是: https : //forums.xamarin.com/discussion/23002/how-do-you-detect-a-grid-click-tap 。 但是,在這種情況下,傳遞的是靜態值,而不是動態值。
我假設您要的是對象的ID,而不是行的ID(因為那不是相關的恕我直言)
因此,Listview有一個ItemTapped事件:
<ListView x:Name="listView"
ItemTapped="TappedListView"
ItemsSource="{Binding People}">
並且,在您的Page.xaml.cs中
private void TappedListView(object sender, ItemTappedEventArgs e)
{
var yourItem = e.Item as Person;
var id = yourItem.Id;
}
您可以在官方文檔中閱讀有關Listview Interactivity的更多信息。
如Jason所說,ListView中有selecteditem屬性,類型是Person模型,您可以在PersonViewmodel中創建Person模型,綁定到ListView SelectedItem並實現INotifyPropertyChanged,以在單擊ListView Item時進行更新。
<ListView
x:Name="listView"
ItemsSource="{Binding peoples}"
SelectedItem="{Binding selecteditem}">
<ListView.Header>
<Grid>
<Grid.ColumnDefinitions>
<ColumnDefinition Width="*" />
<ColumnDefinition Width="*" />
<ColumnDefinition Width="*" />
<ColumnDefinition Width="*" />
</Grid.ColumnDefinitions>
<Grid.RowDefinitions>
<RowDefinition Height="Auto" />
</Grid.RowDefinitions>
<Label Grid.Column="0" Text="Id" />
<Label Grid.Column="1" Text="First Name" />
<Label Grid.Column="2" Text="Surname" />
<Label Grid.Column="3" Text="Date Of Birth" />
</Grid>
</ListView.Header>
<ListView.ItemTemplate>
<DataTemplate>
<ViewCell>
<Grid>
<Grid.ColumnDefinitions>
<ColumnDefinition Width="*" />
<ColumnDefinition Width="*" />
<ColumnDefinition Width="*" />
<ColumnDefinition Width="*" />
</Grid.ColumnDefinitions>
<Grid.RowDefinitions>
<RowDefinition Height="Auto" />
</Grid.RowDefinitions>
<Label Grid.Column="0" Text="{Binding Id}" />
<Label Grid.Column="1" Text="{Binding FirstName}" />
<Label Grid.Column="2" Text="{Binding Surname}" />
<Label Grid.Column="3" Text="{Binding Age}" />
</Grid>
</ViewCell>
</DataTemplate>
</ListView.ItemTemplate>
</ListView>
public class PersonViewModel:ViewModelBase
{
public ObservableCollection<personmodel> peoples { get; set; }
private personmodel _selecteditem;
public personmodel selecteditem
{
get { return _selecteditem; }
set
{
_selecteditem = value;
RaisePropertyChanged("selecteditem");
}
}
public PersonViewModel()
{
peoples = new ObservableCollection<personmodel>();
peoples.Add(new personmodel { Id = Guid.NewGuid().ToString(), Age = 36, FirstName = "Andrew", Surname = "Smith" });
peoples.Add(new personmodel { Id = Guid.NewGuid().ToString(), Age = 65, FirstName = "David", Surname = "White" });
peoples.Add(new personmodel { Id = Guid.NewGuid().ToString(), Age = 39, FirstName = "Bert", Surname = "Edwards" });
selecteditem = peoples[0];
}
}
public class ViewModelBase: INotifyPropertyChanged
{
public event PropertyChangedEventHandler PropertyChanged;
public void RaisePropertyChanged(string propertyName)
{
PropertyChangedEventHandler handler = PropertyChanged;
if (handler != null)
{
handler(this, new PropertyChangedEventArgs(propertyName));
}
}
}
因此,如果您要將點擊的行ID傳遞給事件處理程序,則只需傳遞
selecteditem.Id
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.