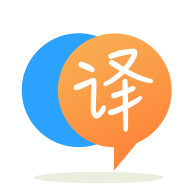
[英]Java guess game. How do I input data validation(range checking) and how do I input guesses?
[英]Java guess game. How do I use data validation to check if a number is within a certain range?
我需要幫助編寫一組數據驗證語句來檢查用戶條目是否在 0 到 100 的范圍內,並且用戶鍵入的任何內容都應顯示錯誤消息,即 ISNT 為 1 到 100 之間的非十進制 integer。 此外,我需要一種方法來編寫如何獲得“再見”output 的代碼,以便僅在用戶輸入“n”而不是“n”和“y”時顯示。 N 表示否,y 表示是。
這是我的代碼。
import java.util.Scanner;
public class GuessingGameCalc {
private static void displayWelcomeMessage(int max) {
System.out.println("Welome to the Java Guessing Game!");
System.out.println(" ");
System.out.println("I'm thinking of a number between 1 and" + " " + max + " " + "let's see if you guess what it is!");
System.out.println(" ");
}
public static int calculateRandomValue(int max) {
double value = (int) (Math.random() * max + 1);
int number = (int) value;
number++;
return number;
}
public static void validateTheData(int count) {
if( count < 3) {
System.out.println("Good job!");
} else if (count < 7) {
System.out.println("Need more practice.");
} else{
System.out.println("Need way more practice.");
}
}
public static void main(String[] args) {
final int max = 100;
String prompt = "y";
displayWelcomeMessage(max);
int unit = calculateRandomValue(max);
Scanner sc = new Scanner(System.in);
int counter = 1;
while (prompt.equalsIgnoreCase("y")) {
while (true) {
System.out.println("Please enter a number.");
int userEntry = sc.nextInt();
if (userEntry < 1 || userEntry > max) {
System.out.println("Invalid guess! Guess again!");
continue;
}
if (userEntry < unit) {
if ( (unit - userEntry) > 10 ) {
System.out.println("Way Too low! Guess higher!");
} else {
System.out.println("Too low! Guess higher!");
}
} else if (userEntry > unit) {
if( (userEntry - unit) > 10 ){
System.out.println("Way Too high! Guess lower!");
} else {
System.out.println("Too high! Guess lower!");
}
} else {
System.out.println("Congratulations! You guessed it in" + " " + counter + " " + "tries!\n");
validateTheData(counter);
break;
}
counter++;
}
System.out.println("Would you like to try again? Yes or No?");
prompt = sc.next();
System.out.println("Goodbye!");
}
}
}
而不是使用.nextInt()
而是使用.nextLine()
,它返回一個String
,然后將其解析為一個int
並捕獲NumberFormatException
所以基本上你會有這樣的結構:
try {
int userEntry = Integer.parseInt(sc.nextLine());
...
} catch (NumberFormatException nfe) {
System.out.println("Please enter a valid number.");
}
哦,只是對您代碼的 rest 的評論。 你真的不需要兩個while
循環,一個就足夠了。
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.