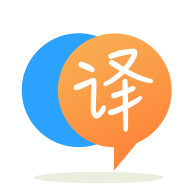
[英]Angular 7 - forEach iteration inside of a promise is executing after the promise resolves. Why?
[英]Go to the next forEach iteration after promise resolves
我將通過一些數據數組,在每次迭代中我都會處理 promise。
我想要的是 go 到下一次forEach
迭代,只有當當前迭代上的 promise 得到解決時。
我搜索了不同的解決方案,發現使用for(... of...)
而不是forEach
可以解決問題。 但還是想不通。
const data = ['apple', 'orange', 'banana'];
data.forEach((row, rowIndex) => {
let params = {
fruitIndex: rowIndex,
};
axios.post('/fruits', { ...params })
.then(response => {
// go to the next iteration of forEach only after this promise resolves
})
.catch(error => console.log(error))
});
遞歸有助於:
const data = ['apple', 'orange', 'banana'];
function request_fruit(n) {
let params = {
fruitIndex: n,
};
axios.post('/fruits', { ...params })
.then(response => {
// Work with recived...
// ....
// Request next
if(n+1<fruits.length) request_fruit(n+1)
})
.catch(error => console.log(error))
};
request_fruit(0)
如果可以的話,我會推薦一個易於閱讀和理解的帶有等待/異步的 for of 循環。 請注意,頂級 await 是最近添加的,因此您應該將其包裝在一些異步 function 中(無論如何您都可能這樣做)。
如果你不能使用 async/await,你可以使用reduce
和初始解析的 promise 來鏈接后續調用。
請注意,我使用了 function sleep
,它在一段時間后解決了 promise。 sleep
調用應該可以與 axios 調用互換。
const sleep = (ms) => new Promise(resolve => setTimeout(resolve, ms)); const data = [1000, 2000, 3000]; function withReduce() { console.log('Sequential Promises with Reduce:'); return data.reduce((previousPromise, ms) => { return previousPromise.then(() => { console.log(`sleeping for ${ms}ms`); return sleep(ms); }); }, Promise.resolve()); } async function withAsync() { console.log('Sequential Promises with for of and await:'); for (let ms of data) { console.log(`sleeping for ${ms}ms`); await sleep(ms); } } withReduce().then(withAsync);
看起來還沒有人發布特定的 async/await,所以這就是你如何將它與 for...of 一起使用:
const data = ['apple', 'orange', 'banana'];
for (const [rowIndex, row] of data.entries()) {
let params = {
fruitIndex: rowIndex,
};
let response;
try {
response = await axios.post('/fruits', { ...params });
} catch (error) {
console.log(error);
continue;
}
// …
}
這一切都放在異步 function 中。 (另外, {...params }
有點奇怪。直接使用params
有什么問題?)
只需在 api 調用上使用 await 即可。
function postFruitData(){
const data = ['apple', 'orange', 'banana'];
data.forEach(async (row, rowIndex) => {
let params = {
fruitIndex: rowIndex,
};
const response = await axios.post('/fruits', { ...params })
});
}
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.