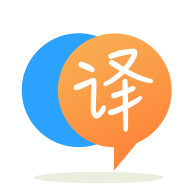
[英]Async CanExecute method using DelegateCommand in MVVM
[英]How do I get DelegateCommand CanExecute to update WPF UI using MVVM?
解決這個問題,我試圖用兩個按鈕制作一個 UI。 按下一個按鈕會禁用自身並啟用另一個按鈕。 我正在使用 MVVM 模式和 DelegateCommand:
主窗口 XAML
<Window x:Class="WpfApp1.MainWindow"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:d="http://schemas.microsoft.com/expression/blend/2008"
xmlns:mc="http://schemas.openxmlformats.org/markup-compatibility/2006"
xmlns:local="clr-namespace:WpfApp1"
mc:Ignorable="d"
Title="MainWindow" Height="450" Width="800">
<Window.DataContext>
<local:ViewModel />
</Window.DataContext>
<StackPanel>
<Rectangle Height="100"></Rectangle>
<Button Command="{Binding StartServer}" >Start Server</Button>
<Button Command="{Binding StopServer}" >Stop Server</Button>
</StackPanel>
</Window>
視圖模型
public class ViewModel : INotifyPropertyChanged
{
public ViewModel()
{
PropertyChanged += PropertyChangedHandler;
}
private void PropertyChangedHandler(object sender, PropertyChangedEventArgs e)
{
switch (e.PropertyName)
{
case nameof(ServerIsRunning):
StartServer.RaiseCanExecuteChanged();
StopServer.RaiseCanExecuteChanged();
break;
}
}
private bool m_serverIsRunning;
public bool ServerIsRunning
{
get => m_serverIsRunning;
set
{
m_serverIsRunning = value;
RaisePropertyChanged(nameof(ServerIsRunning));
}
}
public DelegateCommand<object> StartServer => new DelegateCommand<object>(
context =>
{
ServerIsRunning = true;
}, e => !ServerIsRunning);
public DelegateCommand<object> StopServer => new DelegateCommand<object>(
context =>
{
ServerIsRunning = false;
}, e => ServerIsRunning);
public event PropertyChangedEventHandler PropertyChanged;
public void RaisePropertyChanged(string property)
{
PropertyChanged?.Invoke(this, new PropertyChangedEventArgs(property));
}
}
委托命令
public class DelegateCommand<T> : ICommand
{
private readonly Action<object> ExecuteAction;
private readonly Func<object, bool> CanExecuteFunc;
public DelegateCommand(Action<object> executeAction, Func<object, bool> canExecuteFunc)
{
ExecuteAction = executeAction;
CanExecuteFunc = canExecuteFunc;
}
public bool CanExecute(object parameter)
{
return CanExecuteFunc == null || CanExecuteFunc(parameter);
}
public void Execute(object parameter)
{
ExecuteAction(parameter);
}
public event EventHandler CanExecuteChanged;
public void RaiseCanExecuteChanged()
{
CanExecuteChanged?.Invoke(this, EventArgs.Empty);
}
}
問題是,當我單擊Start Server
按鈕時,按鈕的 IsEnabled state 在 UI 中沒有改變。 我是否不正確地使用了 DelegateCommand?
您的代碼的問題在於您的命令屬性使用表達式主體,每次有人獲取屬性時都會執行它們,這意味着每次您請求StartServer
命令時,它都會為您提供一個新實例。 您可以通過在 getter 上放置一個斷點來自己檢查這一點,您會看到它會被多次命中,並且總是會創建一個新的委托命令實例。
為了使您的代碼工作,您必須使用每個命令的一個實例,您可以通過使用支持字段或只讀屬性並將其設置在構造函數中來實現這一點:
public MainWindowViewModel()
{
PropertyChanged += PropertyChangedHandler;
StartServer = new DelegateCommand<object>(
context =>
{
ServerIsRunning = true;
}, e => !ServerIsRunning);
StopServer = new DelegateCommand<object>(
context => {
ServerIsRunning = false;
}, e => ServerIsRunning);
}
public DelegateCommand<object> StartServer
{ get; }
public DelegateCommand<object> StopServer
{ get; }
UI 只會監聽它所綁定的命令實例引發的CanExecuteChanged
事件(通過Command={Binding...}
。
您的代碼也可以簡化,因為您可以直接調用RaiseCanExecuteChanged
而不是引發PropertyChangedEvent
並自己聽:
public bool ServerIsRunning
{
get => m_serverIsRunning;
set
{
m_serverIsRunning = value;
StartServer.RaiseCanExecuteChanged();
StopServer.RaiseCanExecuteChanged();
}
}
像這樣的東西?
public class ViewModel : INotifyPropertyChanged
{
bool _isServerStarted;
public ViewModel()
{
StartServer = new DelegateCommand(OnStartServerExecute, OnStartServerCanExecute);
StopServer = new DelegateCommand(OnStopServerExecute, OnStopServerCanExecute);
}
void OnStartServerExecute()
{
IsServerStarted = true;
}
bool OnStartServerCanExecute()
{
return !IsServerStarted;
}
void OnStopServerExecute()
{
IsServerStarted = false;
}
bool OnStopServerCanExecute()
{
return IsServerStarted;
}
void RaiseCanExecuteChanged()
{
StartServer.RaiseCanExecuteChanged();
StopServer.RaiseCanExecuteChanged();
}
public bool IsServerStarted
{
get => _isServerStarted;
set
{
_isServerStarted = value;
PropertyChanged?.Invoke(this, new PropertyChangedEventArgs(nameof(IsServerStarted)));
RaiseCanExecuteChanged();
}
}
public DelegateCommand StartServer { get; }
public DelegateCommand StopServer { get; }
public event PropertyChangedEventHandler PropertyChanged;
}
您只需使用 CommandManager.InvalidateRequerySuggested() 來更新按鈕 state。
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.