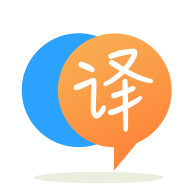
[英]Getting Error When Posting data into Azure Cosmos DB using Azure functions C#?
[英]Cosmos DB query using SQL works when running in Data explorer but not when executed with the C# DocumentClient
我有一個查詢,旨在計算特定類型的新鮮和陳舊文檔的數量。
鑒於我嘗試在一個查詢中獲取多個類型的計數,因此很有可能應該重寫查詢,並且新版本在使用 C# 時按預期工作。
如果我使用“新 SQL 查詢”在 Cosmos DB 數據資源管理器中運行該查詢,但是當使用Microsoft.Azure.DocumentDB.Core
中 DocumentClient 的2.2.2
和2.8.1
(升級到最新版本)版本運行相同的查詢時。 Microsoft.Azure.DocumentDB.Core
nuget package 它沒有。
鑒於以下文件:
[{
"PartitionKey": "partition",
"SomeKey": "foo",
"Type": "DocumentType",
"Value": "1",
"StaleAfterThis": 101
},
{
"PartitionKey": "partition",
"SomeKey": "bar",
"Type": "DocumentType",
"Value": "2",
"StaleAfterThis": 90
},
{
"PartitionKey": "partition",
"SomeKey": "bar",
"Type": "DocumentType",
"Value": "3",
"StaleAfterThis": 500
},
{
"PartitionKey": "partition",
"SomeKey": "foo",
"Type": "DocumentType",
"Value": "4",
"StaleAfterThis": 500
}]
以及以下查詢:
SELECT
SUM((SELECT VALUE Count(1) WHERE c.SomeKey = 'foo' AND c.PartitionKey = 'partition' AND c.StaleAfterThis < 100)) as StaleFooCount,
SUM((SELECT VALUE Count(1) WHERE c.SomeKey = 'foo' AND c.PartitionKey = 'partition' AND c.StaleAfterThis >= 100)) as FreshFooCount,
SUM((SELECT VALUE Count(1) WHERE c.SomeKey = 'bar' AND c.PartitionKey = 'partition' AND c.StaleAfterThis < 100)) as StaleBarCount,
SUM((SELECT VALUE Count(1) WHERE c.SomeKey = 'bar' AND c.PartitionKey = 'partition' AND c.StaleAfterThis >= 100)) as FreshBarCount
FROM c
WHERE
c.Type = 'DocumentType' AND
c.PartitionKey = 'partition'
在數據資源管理器中基於 web 的查詢編輯器中運行此查詢時,結果符合預期。
[
{
"StaleFooCount": 0,
"FreshFooCount": 1,
"StaleBarCount": 1,
"FreshBarCount": 1
}
]
如果我使用 C# 嘗試相同的查詢,則 DocumentClient 的所有值都是 0。返回動態結果和鍵入結果時都是這種情況。
var queryOptions = new FeedOptions { MaxItemCount = 1 };
var queryText =
@"SELECT
SUM((SELECT VALUE Count(1) WHERE c.SomeKey = '@foo' AND c.PartitionKey = '@partitionKey' AND c.StaleAfterThis < @staleAfterThis)) as StaleFooCount,
SUM((SELECT VALUE Count(1) WHERE c.SomeKey = '@foo' AND c.PartitionKey = '@partitionKey' AND c.StaleAfterThis >= @staleAfterThis)) as FreshFooCount,
SUM((SELECT VALUE Count(1) WHERE c.SomeKey = '@bar' AND c.PartitionKey = '@partitionKey' AND c.StaleAfterThis < @staleAfterThis)) as StaleBarCount,
SUM((SELECT VALUE Count(1) WHERE c.SomeKey = '@bar' AND c.PartitionKey = '@partitionKey' AND c.StaleAfterThis >= @staleAfterThis)) as FreshBarCount
FROM c
WHERE
c.Type = 'DocumentType' AND
c.PartitionKey = '@partitionKey'";
var queryParameters = new SqlParameterCollection
{
new SqlParameter("@partitionKey", "partition"),
new SqlParameter("@foo", FooBarEnum.Foo.ToString()),
new SqlParameter("@bar", FooBarEnum.Bar.ToString()),
new SqlParameter("@staleAfterThis", 100)
};
var sqlQuerySpec = new SqlQuerySpec(queryText, queryParameters);
var query = _client.CreateDocumentQuery<TempResult>(_collectionLink, sqlQuerySpec, queryOptions).AsDocumentQuery();
var totalStaleDocuments = new TempResult();
while (query.HasMoreResults)
{
foreach (var result in await query.ExecuteNextAsync<TempResult>())
{
totalStaleDocuments.FreshFooCount += result.FreshFooCount;
totalStaleDocuments.StaleFooCount += result.StaleFooCount;
totalStaleDocuments.FreshBarCount += result.FreshBarCount;
totalStaleDocuments.StaleBarCount += result.StaleBarCount;
}
}
public class TempResult
{
public int StaleFooCount { get; set; }
public int FreshFooCount { get; set; }
public int StaleBarCount { get; set; }
public int FreshBarCount { get; set; }
}
[
{
"StaleFooCount": 0,
"FreshFooCount": 1,
"StaleBarCount": 1,
"FreshBarCount": 1
}
]
[
{
"StaleFooCount": 0,
"FreshFooCount": 0,
"StaleBarCount": 0,
"FreshBarCount": 0
}
]
您的 C# 查詢不一樣。 在您的門戶查詢中,您正在執行c.SomeKey = 'foo'
而在 C# 中,您正在執行c.Type = '@foo'
最后,從查詢參數中刪除'
,如下所示:
var queryText =
@"SELECT
SUM((SELECT VALUE Count(1) WHERE c.SomeKey = @foo AND c.PartitionKey = @partitionKey AND c.StaleAfterThis < @staleAfterThis)) as StaleFooCount,
SUM((SELECT VALUE Count(1) WHERE c.SomeKey = @foo AND c.PartitionKey = @partitionKey AND c.StaleAfterThis >= @staleAfterThis)) as FreshFooCount,
SUM((SELECT VALUE Count(1) WHERE c.SomeKey = @bar AND c.PartitionKey = @partitionKey AND c.StaleAfterThis < @staleAfterThis)) as StaleBarCount,
SUM((SELECT VALUE Count(1) WHERE c.SomeKey = @bar AND c.PartitionKey = @partitionKey AND c.StaleAfterThis >= @staleAfterThis)) as FreshBarCount
FROM c
WHERE
c.Type = 'DocumentType' AND
c.PartitionKey = @partitionKey";
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.