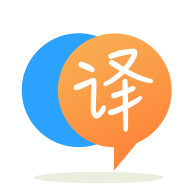
[英]How to fix thid error ArrayIndexOutOfBoundsException: Index 1 out of bounds for length 1?
[英]How to fix “Index 5 out of bounds for length 5” error in Java
我正在研究 CCC 2018 Robo Thieves 問題的解決方案,它是原始問題的簡化版本。 我的問題是,當我執行我的代碼時,它會給我這個“索引 5 超出長度 5 的范圍”,我不確定它為什么會發生。 我的程序執行了一半,然后發生此錯誤。
import java.util.*;
public class RoboThieves {
public static void main(String args[]) {
Scanner sc = new Scanner(System.in);
ArrayList<Integer> rowPos = new ArrayList<Integer>();
ArrayList<Integer> colPos = new ArrayList<Integer>();
int sRow = -1; // robot pos
int sCol = -1;
int dotCounter = 0;
int stepCounter = 0;
int rowSize = sc.nextInt();
int colSize = sc.nextInt();
char[][] factoryGrid = new char[rowSize][colSize];
for (int i = 0; i < rowSize; i++) {
String rowChars = sc.next().toUpperCase();
for (int j = 0; j < colSize; j++) {
factoryGrid[i][j] = rowChars.charAt(j);
}
}
// check to see if the grid was inputted properly (with square brackets)
/*
* for (char [] row: factoryGrid) { System.out.println(Arrays.toString(row)); }
*/
// check to see if the grid was inputted properly (as inputted)
for (int i = 0; i < rowSize; i++) {
for (int j = 0; j < colSize; j++) {
System.out.print(factoryGrid[i][j]);
}
System.out.println();
}
// locate dots and store their row and col in arraylists
for (int i = 0; i < rowSize; i++) {
for (int j = 0; j < colSize; j++) {
if (factoryGrid[i][j] == '.') {
rowPos.add(i);
colPos.add(j);
dotCounter++;
}
}
}
// print dot location to check
for (int i = 0; i < rowPos.size(); i++) {
System.out.println("Dot Position = " + "(" + rowPos.get(i) + "," + colPos.get(i) + ")");
}
// locate robot position
for (int i = 0; i < rowSize; i++) {
for (int j = 0; j < colSize; j++) {
if (factoryGrid[i][j] == 'S')
sRow = i;
sCol = j;
}
}
// print camera location to check
System.out.println("Camera Position = " + "(" + sRow + "," + sCol + ")");
//System.out.println(dotCounter); // test to see if counter works
char above = getAbove(factoryGrid, sRow, sCol);
char right = getRight(factoryGrid, sRow, sCol);
char below = getBelow(factoryGrid, sRow, sCol);
char left = getLeft(factoryGrid, sRow, sCol);
if (above == '.') {
boolean canMove = check360(factoryGrid, sRow, sCol);
// check if camera is around dot
if (canMove == true) {
// set robot position to dot position and old position to W
sRow = sRow - 1;
// sCol = sCol;
factoryGrid[sRow][sCol] = 'W';
dotCounter--;
stepCounter++;
} else {
// this is if there is a camera in the 360 radius of the open space
System.out.println("You cannot move to the space beside because there is a camera in your sightline!");
}
} else if (right == '.') {
boolean canMove = check360(factoryGrid, sRow, sCol);
// check if camera is around dot
if (canMove == true) {
// set robot position to dot position and old position to W
// sRow = sRow;
sCol = sCol + 1;
factoryGrid[sRow][sCol] = 'W';
dotCounter--;
stepCounter++;
} else {
// this is if there is a camera in the 360 radius of the open space
System.out.println("You cannot move to the space beside because there is a camera in your sightline!");
}
} else if (below == '.') {
boolean canMove = check360(factoryGrid, sRow, sCol);
// check if camera is around dot
if (canMove == true) {
// set robot position to dot position and old position to W
sRow = sRow + 1;
// sCol = sCol;
factoryGrid[sRow][sCol] = 'W';
dotCounter--;
stepCounter++;
} else {
// this is if there is a camera in the 360 radius of the open space
System.out.println("You cannot move to the space beside because there is a camera in your sightline!");
}
} else if (left == '.') {
boolean canMove = check360(factoryGrid, sRow, sCol);
// check if camera is around dot
if (canMove == true) {
// set robot position to dot position and old position to W
// sRow = sRow;
sCol = sCol - 1;
factoryGrid[sRow][sCol] = 'W';
dotCounter--;
stepCounter++;
} else {
// this is if there is a camera in the 360 radius of the open space
System.out.println("You cannot move to the space beside because there is a camera in your sightline!");
}
} else {
System.out.println(
"The robot cannot move to any spaces try inputting a factory layout that can produce an answer.");
} // end if above dot (yes)
System.out.println(stepCounter);
for (int i = 0; i < rowSize; i++) {
for (int j = 0; j < colSize; j++) {
System.out.print(factoryGrid[i][j]);
}
System.out.println();
}
} // end main method
public static char getLeft(char[][] factGrid, int cRow, int cCol) {
return factGrid[cRow][(cCol - 1)];
}
public static char getAbove(char[][] factGrid, int cRow, int cCol) {
return factGrid[(cRow - 1)][(cCol)];
}
public static char getBelow(char[][] factGrid, int cRow, int cCol) {
return factGrid[cRow + 1][cCol];
}
public static char getRight(char[][] factGrid, int cRow, int cCol) {
return factGrid[cRow][(cCol + 1)];
}
public static boolean check360(char[][] factGrid, int cRow, int cCol) {
boolean canMove = true;
char left = getLeft(factGrid, cRow, cCol);
char above = getAbove(factGrid, cRow, cCol);
char right = getRight(factGrid, cRow, cCol);
char below = getBelow(factGrid, cRow, cCol);
if (left == 'C' || above == 'C' || right == 'C' || below == 'C') {
canMove = false;
}
return canMove;
}
} // end main program
乍一看,我認為問題可能在於您的 getLeft()、getAbove()、getRight() 和 getBelow() 方法。
在這些方法中,你給它一個 cRow 和 cCol 的值並加或減 1。但是,你需要確保你查詢的索引不超過雙數組factGrid的大小,或者 go 小於 0。
例如,在您的 getRight() 方法中,您可以嘗試:
public static char getRight(char[][] factGrid, int cRow, int cCol) {
if(factGrid[0].length > (cCol + 1)) {
return factGrid[cRow][(cCol + 1)];
} else {
return '';
}
}
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.