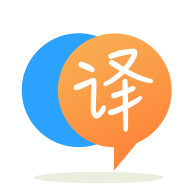
[英]How to create a native C++ DLL that I can run with rundll32.exe without specifying an entry point
[英]How can I turn my program into a .dll file and run it in cmd using rundll32.exe?
我有一個程序可以創建多個線程並在循環中打印一些字符串。 我的任務是將這個程序變成 a.dll 並使用 rundll32.exe 運行它,但我不知道如何將 a.dll 作為可執行文件運行。
#define _CRT_SECURE_NO_WARNINGS
#include<windows.h>
#include <stdlib.h>
#include<process.h>
#include<stdio.h>
#include<string>
#include<ctime>
#include<vector>
#include<iostream>
typedef struct {
std::string info;
unsigned int m_number;
int m_stop_thread;
int m_priority_thread;
unsigned m_cycles;
unsigned m_currentThread;
}data;
HANDLE tmp;
unsigned int __stdcall Func(void* d) {
data* real = (data*)d;
std::cout << "\nCurrent thread ID: " << GetCurrentThreadId() << std::endl;
if (real->m_currentThread == real->m_priority_thread)
SetThreadPriority(tmp, 2);
std::cout << "Thread priority: " << GetThreadPriority(tmp) << std::endl;
for (int j = (real->m_currentThread - 1) * real->m_cycles / real->m_number;j < real->m_currentThread * real->m_cycles / real->m_number;j++) {
for (int i = 0;i < real->info.size();++i)
std::cout << real->info[i];
std::cout << std::endl;
}
return 0;
}
int main(int argc, char* argv[]) {
int threadsNumber, priority, stop;
std::string str;
std::cout << "Enter the info about a student:\n";
std::getline(std::cin, str);
std::cout << "Enter the number of threads:\n";
std::cin >> threadsNumber;
int cycles;
std::cout << "Enter the number of cycles:\n";
std::cin >> cycles;
std::cout << "Which thread priority do you want to change? ";
std::cin >> priority;
std::cout << "Which thread do you want to stop? ";
std::cin >> stop;
std::vector<HANDLE> threads;
data* args = new data;
args->info = str;
args->m_number = threadsNumber;
args->m_cycles = cycles;
args->m_priority_thread = priority;
args->m_stop_thread = stop;
clock_t time = clock();
for (int i = 1;i <= threadsNumber;++i) {
args->m_currentThread = i;
tmp = (HANDLE)_beginthreadex(0, 0, &Func, args, 0, 0);
threads.push_back(tmp);
}
WaitForMultipleObjects(threads.size(), &threads.front(), TRUE, INFINITE);
time = clock() - time;
std::cout << "time: " << (double)time / CLOCKS_PER_SEC << "s" << std::endl << std::endl;
getchar();
return 0;
}
有誰知道如何將此代碼放入 dll 並使用命令行運行它?
當你編譯你的程序時,你在 Windows 中創建了一個叫做Portable Executable [PE]的東西。 共享該家族的文件包括.exe
、 .dll
、 .scr
,您可以通過在文本編輯器(例如記事本)中打開它們並查看文件是否以MZ
開頭(這是 Mark Zbikowski 的簽名)來識別它們。
簡而言之, *.dll
或*.exe
沒有太大區別,除了一些取決於版本的小塊。 所以簡而言之,當你編譯它時,你正在制作一個“dll”。 但是,如果您希望將程序編譯為dll
,這取決於您的編譯器:
但我會小心部署此類文件,因為@Richard 很好地指出RunDll32已被棄用,但它仍用於某些編程語言庫的齒輪中。 因此,如果您正在構建用於自測目的的東西,我會根據您的編譯器推薦這 4 個選項。
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.