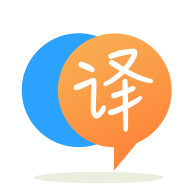
[英]How to use a custom comparer for EqualityComparer<T>.Default?
[英]What comparer should I use for a .NET Dictionary to use the EqualityComparer<T> methods of the Key?
我的Position
class 派生自EqualityComparer<T>
class。 我希望字典使用覆蓋的方法,但它使用的是Object.Equals
和Object.GetHashCode
中的方法。 我應該為Dictionary
使用什么比較器來使用Position
class 的EqualityComparer<T>
方法?
using System.Collections.Generic;
using System.Diagnostics;
namespace ConsoleApp1
{
class Position : EqualityComparer<Position>
{
public int X { get; set; }
public int Y { get; set; }
public override bool Equals(Position left, Position right)
{
if (left == null || right == null)
return false;
return left.X == right.X && left.Y == right.Y;
}
public override int GetHashCode(Position cell)
{
if (cell == null)
return 0;
return cell.X * 31 + cell.Y;
}
}
class Program
{
static void Main(string[] args)
{
var dictionary = new Dictionary<Position, int>();
var position = new Position();
position.X = 1;
position.Y = 1;
dictionary[position] = 1;
position = new Position();
position.X = 1;
position.Y = 1;
var found = dictionary.TryGetValue(position, out var result);
Debug.Assert(found);
Debug.Assert(result == 1);
}
}
}
雖然在 object 本身上實現比較器非常
尷尬
,但您只需將比較器的實例傳遞給Dictionary
構造函數,如EqualityComparer示例所示。
var dictionary = new Dictionary<Position, int>(new Position());
更常見的方法是
Equals
和GetHashCode
實現IEquatable<T>
(如果在您的情況下存在自然平等)。 這比object.Equals
方法更可取,因為Equals(object)
強制對值類型進行裝箱。object.Equals
和object.GetHashCode
,如約翰的答案所示 - 如果您的類型具有自然相等並且它是引用類型( class
),這是最直接的方法。 替代答案:您可以覆蓋Position
的GetHashCode
和Equals
方法:
class Position
{
public int X { get; set; }
public int Y { get; set; }
public override bool Equals(object other)
{
if (other == null || !(other is Position))
{
return false;
}
var otherPosition = (Position)other;
return otherPosition.X == this.X && otherPosition.Y == this.Y;
}
public override int GetHashCode()
{
return this.X * 31 + this.Y;
}
}
這樣你就可以像這樣簡單地聲明你的字典:
var dict = new Dictionary<Position, string>();
它會按您的預期工作。
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.