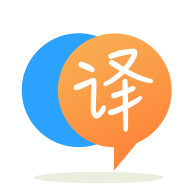
[英]How do you replace the elements of an array with elements from another array?
[英]How do i 'replace' an array by filling an empty array's elements using pop method from another array?
我正在嘗試在 python 中實現一個堆棧,並且我正在嘗試使用list
數據結構。 如果我想使用 pop 方法通過使用現有數組中的元素來“填充”一個空數組,我該怎么辦?
# Implementing Stacks in Python through the given list structure
practiceStack = []
practiceStack.append(['HyunSoo', 'Shah'])
practiceStack.append('Jack')
practiceStack.append('Queen')
practiceStack.append(('Aces'))
# printing every element in the list/array
for i in practiceStack:
print(i)
# since stacks are LIFO (last in first out) or FILO (first in last out), the pop method will remove the first thing we did
emptyArrayPop = []
這就是我嘗試過的(通過使用 for 循環)並不斷收到use integers not list
錯誤
for i in practiceStack:
emptyArrayPop[i].append(practiceStack.pop)
print(emptyArrayPop)
pop
的 function 是 function — 不是一個值。 換句話說, practiceStack.pop
是一個指向function 的指針(在您花更多時間編寫代碼之前,您幾乎可以忽略它); 你可能想要這個:
practiceStack.pop()
您還需要 append 到列表中; 使用append
添加內容時,列表將自動添加到末尾; 您不需要提供索引。
進一步說明: List.append 方法將采用您傳遞給它的值並將其添加到列表的末尾。 例如:
A = [1, 2, 3]
A.append(4)
A
現在是[1, 2, 3, 4]
。 如果您嘗試運行以下命令:
A[2].append(4)
...那么您實際上是在說,“將 4 附加到A
中位置 2 的值的末尾”,(在上面的示例中,`A[2] 設置為 3;請記住 python 列表從 0 開始計數,或者是“0-index”。)這就像說“將 4 附加到 3”。 這沒有任何意義; 它對 append 和 integer 對另一個 integer 沒有任何意義。
相反,您想將 append 放到 LIST 本身; 您不需要指定 position。
不要將此與為列表中的 position 分配值混淆; 如果您在列表的現有 position 上設置值,則可以使用=
運算符:
>>> B = [1, 2, 3]
>>> B[2]
3
>>> B[2] = 4
>>> print(B)
[1, 2, 4]
>>> B.append(8)
>>> print(B)
[1, 2, 4, 8]
因此,要回答您的原始問題,您想要的行如下:
emptyArrayPop.append(practiceStack.pop())
(注意[i]
已被刪除)
[編輯]正如@selcuk 指出的那樣,這不是唯一的問題。
您還需要修復在practiceStack
列表中訪問數據的方式,因為在迭代列表時無法編輯列表(調用pop
會就地修改列表)。
您需要遍歷列表的 integer 索引才能訪問practiceStack
的元素:
for i in range(len(practiceStack)):
emptyArrayPop.append(practiceStack.pop())
practiceStack = []
practiceStack.append(['HyunSoo', 'Shah'])
practiceStack.append('Jack')
practiceStack.append('Queen')
practiceStack.append(('Aces'))
# printing every element in the list/array
for i in practiceStack:
print(i)
# since stacks are LIFO (last in first out) or FILO (first in last out), the pop method will remove the first thing we did
emptyArrayPop = []
for i in range(len(practiceStack)):
emptyArrayPop.append(practiceStack[i])
print(emptyArrayPop)
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.