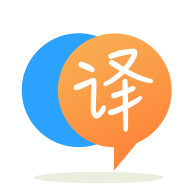
[英]How Read a Text File and Display it onto a TextBlock in Visual Studio (C#)
[英]How to read from a text file that is constantly updating and write to a TextBlock in Visual Studio? [on hold]
我正在做一個項目,需要報告5個超聲波傳感器的距離,該傳感器每200ms更新一次距離。 我想在GUI中更新這些距離。 我目前已將距離寫入文本文件。 我將如何從該文本文件讀取並獲得所有五個距離來寫入TextBlocks,並每200ms更新一次?
您好,歡迎@ mjbll123,
您可以通過一種小方法強制程序“等待”直到文件可用:
public string WaitForFile(string file)
{
try
{
//using variable to make sure the return doesn't get executed
string tmp = File.ReadAllText(file);
return tmp;
}
catch(Exception)
{
return WaitForFile(file);
}
}
這實際上是在嘗試訪問文件並立即讀取它。 如果失敗( IOException
),則它將再次嘗試(在catch
塊中)。
您可以使用System.IO.FileSystemWatcher
類。 更改文件時將引發一個事件。 您可以在事件中讀取文件並更新GUI。 示例方法如下:
private void watch()
{
FileSystemWatcher watcher = new FileSystemWatcher();
watcher.Path = yourFilePath;
watcher.NotifyFilter = NotifyFilters.LastWrite;
watcher.Filter = "*.*";
watcher.Changed += new FileSystemEventHandler(OnChanged);
watcher.EnableRaisingEvents = true;
}
在EventHandler中,請確保使用下面的命令讀取文件,以確保在打開事件以供其他程序編寫時讀取事件。
private void OnChanged(object source, FileSystemEventArgs e)
{
using (var fs = new FileStream(path, FileMode.Open, FileAccess.Read, FileShare.ReadWrite))
using (var sr = new StreamReader(fs, Encoding.Default)) {
// read the stream
var fileText = sr.ReadToEnd();
}
}
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.