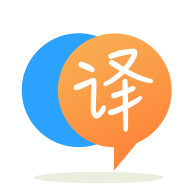
[英]undefined reference to `readVector(std::__cxx11::basic_string<char, std::char_traits<char>, std::allocator<char> >)'
[英]Getting unknown Segmentation Fault "(address) in std::__cxx11::basic_string<char, std::char_traits<char>,..., std::allocator<char> > const&) const ()
我正在用 C++ 編寫一個程序,它代表一個具有這些類和結構的論壇:
struct Date{ /* struct used to get a random day for each comment */
int d,m,y,h,min;
Date();
void GetDate() const;
};
class Post{
private:
std::string Title;
std::string Creator;
std::string text;
Date PostDate;
int id;
public:
Post();
~Post();
void postprint() const;
int idprint();
std::string get_creator() const; //returns the creator of the post
};
class Thread{
private:
std::string Subject;
std::string Creator;
Date ThreadDate;
int post_nums = rand()%10+1; // this will be the number of posts that will be made in the thread, used in threadprint()
Post *posts = new Post[post_nums]; //so the randomizer doesn't return 0 array cells, max 10 posts for each thread
public:
Thread();
~Thread();
std::string subjprint() const;
void threadprint() const;
int getnums() const; //returns the number of posts in the posts array
Post *getposts() const; //returns to a pointer the array of posts
};
class Forum{
private:
std::string Title;
Thread *threads = new Thread[3];
//void printt(int) const;
public:
Forum(const std::string);
~Forum();
void Print_threads() const;
void Print_specified_thread(const std::string);
Thread *Find_thread_subject(const std::string);
void Print_specified_post(const int);
Post *Find_specified_post(const int);
};
struct Post_list{
Post *post;
Post_list *next;
Post_list *prev;
};
struct Tree_node{
std::string Creator;
Post_list *list_ptr;
Tree_node *left;
Tree_node *right;
};
class Thread_tree{
private:
Tree_node *node_root;
void Tree_create(Tree_node *);
int Tree_empty(Tree_node *);
void Tree_sort(const Thread *, Tree_node *);
void Tree_insert(const std::string, Tree_node *);
public:
Thread_tree(const Thread *);
};
現在我試圖將每個線程的評論的創建者放入一個 BST 中,將它們整理出來,其中每個節點struct Tree_node
都有一個創建者字符串和他在列表中的評論。 class Thread_tree
構造class Thread_tree
是這樣的
Thread_tree::Thread_tree(const Thread *Thread1){ //constructor only to be called once
cout << "Creating tree of post creators from thread " << Thread1->subjprint() << endl;
Tree_create(this->node_root); //points root to NULL
Tree_sort(Thread1, this->node_root);
}
而Tree_sort()
是
void Thread_tree::Tree_sort(const Thread *Thread1, Tree_node *root){
int k = Thread1->getnums(); //k is assigned to the number of the posts that the thread has
Post *post_ptr = Thread1->getposts(); //ptr is assigned to the posts array of the thread
for (int i=0; i<k; i++){
cout << "\ni is " << i << endl;
Tree_insert((post_ptr+i)->get_creator(), root);
}
}
和Tree_insert()
void Thread_tree::Tree_insert(const string creator, Tree_node *root){
if (Tree_empty(root)){
root = new Tree_node;
root->Creator = creator;
root->list_ptr = NULL;
root->left = NULL;
root->right = NULL;
}
else if (creator.compare(root->Creator) < 0){ //creator in string1 is smaller than the root->Creator
Tree_insert(creator, (root->left)); // in ascending order
}
if (creator.compare(root->Creator) > 0) {//creator in string1 is bigger than the root->Creator
Tree_insert(creator, (root->right)); //in ascending order
}
}
在 main() 中,當我制作一棵樹Thread_tree Tree1(thread);
由於“線程”是指向現有線程的指針,因此出現分段錯誤。 我運行 gdb 調試器,並在使用bt
后收到此消息:
Program received signal SIGSEGV, Segmentation fault.
0x00007ffff7b7625a in std::__cxx11::basic_string<char, std::char_traits<char>, std::allocator<char> >::compare(std::__cxx11::basic_string<char, std::char_traits<char>, std::allocator<char> > const&) const ()
from /usr/lib/x86_64-linux-gnu/libstdc++.so.6
(gdb) bt
#0 0x00007ffff7b7625a in std::__cxx11::basic_string<char, std::char_traits<char>, std::allocator<char> >::compare(std::__cxx11::basic_string<char, std::char_traits<char>, std::allocator<char> > const&) const ()
from /usr/lib/x86_64-linux-gnu/libstdc++.so.6
#1 0x00000000004025f1 in Thread_tree::Tree_insert (this=0x7fffffffe7c0, creator="user1234", root=0x1002) at classes.cpp:211
#2 0x00000000004026be in Thread_tree::Tree_insert (this=0x7fffffffe7c0, creator="user1234", root=0x604110) at classes.cpp:218
#3 0x00000000004024e4 in Thread_tree::Tree_sort (this=0x7fffffffe7c0, Thread1=0x616d98, root=0x604110) at classes.cpp:197
#4 0x00000000004023b7 in Thread_tree::Thread_tree (this=0x7fffffffe7c0, Thread1=0x616d98) at classes.cpp:181
#5 0x0000000000402f03 in main () at main.cpp:19
我不明白問題是什么,從我從消息中了解到的,程序在Tree_insert()
的第二次調用時Tree_insert()
你的問題是Tree_create(this->node_root);
Tree_create
創建傳遞給它的指針的副本並將NULL
分配給該副本,而this->node_root
保持不變並且在Tree_sort
時未初始化。
最簡單的解決方案是將NULL
(或nullptr
,在c++11
更可取)直接分配給this->node_root
這同樣適用於您的其他方法。 當您按值傳遞指針時,對它的任何賦值都是在復制時完成的。 這里的解決方案是通過引用傳遞指針(例如Tree_create(Tree_node*& root)
)
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.