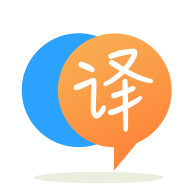
[英]How to reference derived objects in a vector of pointers to base class objects?
[英]Making a vector of base class pointers and pass Derived class objects to it (Polymorphism)
我正在嘗試為我的 Shape 程序實現一個菜單。 我已經實現了所有的形狀類。 兩個直接派生自抽象類“Shape”,另外兩個派生自一個名為“Polygon”的類,該類派生自“Shape”,如下所示:
Shape -> Polygon -> Rectangle, Triangle
`-> Circle, Arrow
在我的菜單類中,我想創建某種類型的數組,該數組可以包含指向對象的指針和基類“Shape”的類型。 但我不確定如何正確地以一種適用於我所有形狀的方式來做,因為我的 2 個課程不是直接從“形狀”派生的。
這是我的菜單類:
class Menu
{
protected:
//array of derived objects
public:
Menu();
~Menu();
// more functions..
void addShape(Shape& shape);
void deleteAllShapes();
void deleteShape(Shape& shape);
void printDetails(Shape& shape);
private:
Canvas _canvas; //Ignore, I use this program to eventually draw this objects to a cool GUI
};
在函數“addShape(Shape& shape);”中,我想用它來將每個給定的形狀添加到我的數組中。 如何實現向其添加新對象? 而且,我如何檢查給定的對象是否來自“多邊形”? 因為如果是這樣,那么據我所知,我需要以不同的方式調用成員函數。
我看到你在菜單中有一個數組,讓我們說:
Shape* myshapes[10];
形狀可以是矩形、三角形、圓形等。您想要的是能夠像這樣使用菜單的 printDetails() 方法:
void printDetails()
{
for(int i = 0; i < size; i++)
{
cout << "Index " << i << " has " << myshapes[i]->getShapeName() << endl;
}
}
getShapeName() 將返回一個字符串,例如“矩形”,如果它是矩形。 您將能夠在純虛函數的幫助下做到這一點。 純虛函數必須在抽象類 Shape 中,它具有:
virtual string getShapeName() = 0; //pure virtual
這意味着我們期望在派生類中定義此函數。 通過這種方式,您將能夠使用形狀數組中的形狀指針使用 getShapeName() 方法,該方法將告訴您形狀是矩形、三角形還是圓形等。
class Shape
{
public:
virtual string getShapeName() = 0;
};
class Circle : public Shape
{
private:
int radius;
public:
Circle(int r) { radius = r; cout << "Circle created!\n"; }
string getShapeName() { return "Circle"; }
};
class Arrow : public Shape
{
private:
int length;
public:
Arrow(int l) { length = l; cout << "Arrow created!\n"; }
string getShapeName() { return "Arrow"; }
};
class Polygon : public Shape
{
public:
virtual string getShapeName() = 0;
};
class Triangle : public Polygon
{
private:
int x, y, z;
public:
Triangle(int a, int b, int c) { x = a; y = b; z = c; cout << "Triangle created!\n"; }
string getShapeName() { return "Triangle"; }
};
class Rectangle : public Polygon
{
private:
int length;
int width;
public:
Rectangle(int l, int w){ length = l; width = w; cout << "Rectangle created!\n"; }
string getShapeName() { return "Rectangle"; }
};
要實現 addShape() 方法,您可以這樣做:
void addShape(Shape &shape)
{
myshapes[count] = &shape;
count++;
}
另外,請記住在 addShape() 方法中通過引用或使用指針傳遞 Shape。 我希望這會有所幫助...祝你好運:-)
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.