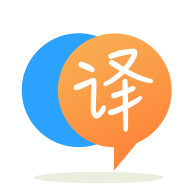
[英]How do I write an iterative function that computes the sum of the first n odd numbers it returns 1 + 3 + … + (2n - 1), using a for loop?
[英]How do I write a function function(n) that takes in an integer, and returns the sum of the first n even numbers using a while loop?
如何編寫一個函數 function(n) 接受一個整數,並使用 while 循環返回前 n 個偶數的總和? 示例:function(3) 返回 12,因為前三個偶數是 2、4、6。
我的代碼:
def function(n):
result = 0
while i < 2*n+1:
if not i%2==1:
result += i
return result
但是我得到的結果是“i”沒有定義。 我感謝幫助和感謝!
你忘了聲明 i 並在每個循環中增加它
def func(n):
result = 0
i = 0
while i < 2*n+1:
if i%2 == 0:
result += i
i += 1
return result
你沒有定義變量i
並且沒有在你的 for 循環中增加i
,這就是為什么你有一個無限循環。
代碼應如下所示:
def function(n):
i = 0
result = 0
while i < 2 * n + 1:
if not i % 2:
result += i
i += 1
return result
但是,下面給出了這樣做的干凈方法:
# Time complexity of O(n)
def fun(n):
result = 0
for i in range(1, n + 1):
result += 2*i
return result
# Efficient as having time complexity of O(1)
def fun2(n):
return n * (n + 1)
print(fun2(3))
print(fun(3))
您可以使用fun2
因為它的時間復雜度為O(1)
。 請參閱此內容以了解更多信息。
@TreysenZobell 的回答解釋了這個問題。 另一種單行解決方案是使用內置sum()
def function(n):
return sum([i for i in range(2 * n + 1) if i % 2 == 0])
不如這些花哨的單襯褲優雅,但想補充一點while True
答案:
def fn(num):
vals, counter = 0, 0
even_nums = []
while True:
if vals < num:
counter += 2
even_nums.append(counter)
vals += 1
else:
return sum(even_nums)
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.