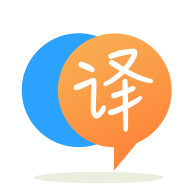
[英]Permutations of 2 characters in Python into fixed length string with equal numbers of each character
[英]Python random string/text/code generator with fixed length and some characters
所以,幾年前,我在麥當勞的禮品卡系統中發現了一個漏洞。 基本點是通過組合大約 15-20 張卡片和它們的代碼,我得到了一個點,即第 3 個和第 7 個字符是相同的,而其他字符是完全隨機的。
我可能在我所在地區(土耳其)舉辦的新可樂節中發現了同樣的事情。 我只是想問,我怎樣才能開發一個帶有隨機模塊的代碼,它會通過遍歷特定算法來隨機創建代碼,例如讓我們再次使用相同的第 3 個和第 7 個字符。
通過保留它們,隨機生成 8 個編號/字母代碼。 另外我認為使用 while True 會更好地生成無限量的它們。 我最終會將它們添加到列表中。 但是,我需要中間部分的幫助。
import random
import string
def randomStringDigits(stringLength=6):
lettersAndDigits = string.ascii_letters + string.digits
return ''.join(random.choice(lettersAndDigits) for i in range(stringLength))
print ("Generating a Random String including letters and digits")
while True:
print ("First Random String is ", randomStringDigits(8))
不確定這樣做的合法性,但問題很簡單。
import random
import string
value_of_seven = 7
value_of_three = 3
def randomString(stringLength=10):
"""Generate a random string of fixed length """
letters = string.ascii_lowercase
_string = ''.join(random.choice(letters) for i in range(stringLength))
print ("Random String is ", randomString() )
return _string
x = 0
string_set = set()
while x <= 10:
x += 1
rand_str = randomString()
if rand_str[-1, 3] is value_of_seven and rand_str[1, 3] is value_of_three:
string_set.add(rand_str)
但我們真的需要知道,只是字母小寫? 大寫?
此外,如果您嘗試在這些地方使用相同的東西生成它們,您仍然會在同一點切片並在末尾添加字符串。
好的,這是符合您要求的工作版本
import random
import string
value_of_seven = '7'
value_of_three = '3'
def _random(stringLength=5):
"""Generate a string of Ch/digits """
lett_dig = string.ascii_letters + string.digits
return ''.join(random.choice(lett_dig) for i in range(stringLength))
if __name__ == '__main__':
s = _random()
s = s[:2] + value_of_three + s[2:]
s = s[:6] + value_of_seven + s[6:]
print(s)
您快到了。 我只是稍微調整了一下 - 你可以按原樣調整它:
import random
numcount = 5
fstring = ""
length_of_code_you_want = 12
position_to_keep_constant_1 = 2 # first position to keep constant is position 3, index 2
position_to_keep_constant_2 = 6 # 2nd position to keep constant is position 7, index 6
constant_1 = "J" # the value of the constant at position 3
constant_2 = "L" # the value of the constant at position 7
for num in range(length_of_code_you_want): #strings are 19 characters long
if random.randint(0, 1) == 1:
x = random.randint(1, 8)
x += 96
fstring += (chr(x).upper())
elif not numcount == 0:
x = random.randint(0, 9)
fstring += str(x)
numcount -= 1
list_out = list(fstring)
list_out[position_to_keep_constant_1] = str(constant_1)
list_out[position_to_keep_constant_2] = str(constant_2)
string_out = "".join(list_out)
print(string_out)
我不確定這樣做是否真的很優雅,但我只是將隨機字母保留在列表中,在各自的位置插入值,然后將列表元素加入字符串中。
import random
import string
first = '3' # the first value to place in the 3rd position
second = '7' # the second value to place in the 7th position of the string
def random_string_digits(string_length):
values = string.ascii_lowercase+string.digits
output = [random.choice(values) for i in range(string_length)]
# the final string length will be equal to string_length+2
# now that we created the random list of characters, it's time to insert the letter in it
output.insert(2, first) # insert the first value in the 3rd position
output.insert(6, second) # insert the second value in the 7th position
return ''.join(output)
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.