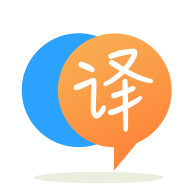
[英]'includes()' is for string, not for array in Javascript?
[英]How to know if a string includes at least one element of array in Javascript
let theString = 'include all the information someone would need to answer your question'
let theString2 = 'include some the information someone would need to answer your question'
let theString3 = 'the information someone would need to answer your question'
let theArray = ['all', 'some', 'x', 'y', 'etc' ]
theString 為真,因為有 'all',theString2 為真,因為有 'some',theString3 為假,因為沒有 'all' 或 'some'
const theStringIsGood = theString.split(' ').some(word => theArray.includes(word))
此代碼將句子拆分為每個單詞,並檢查是否有任何單詞與匹配集 theArray 匹配。
您可以使用 string.includes() 方法。 但在這種情況下, theString3 仍然會返回 true,因為 some 在某事中。 您可以創建一個輔助函數來查看字符串是否在數組中:
function findString(arr, str) {
let flag = false;
let strArr = str.split(' ');
arr.forEach(function(s) {
strArr.forEach(function(s2) {
if(s === s2) {
flag = true;
}
});
});
return flag;
};
現在您可以將數組和字符串之一傳遞給函數並對其進行測試。
你可以用正則表達式簡單地做到這一點:
function ArrayInText(str, words) {
let regex = new RegExp("\\b(" + words.join('|') + ")\\b", "g");
return regex.test(str);
}
在正則表達式中 \\b 是單詞邊界
請注意,如果您自己拆分所有字符串並逐個檢查,則會占用大量內存,因為這是一種貪婪的解決方案。 我提供使用 javascript 本機函數的方法。
片段:
let theString = 'include all the information someone would need to answer your question' let theString2 = 'include some the information someone would need to answer your question' let theString3 = 'the information someone would need to answer your question' let theArray = ['all', 'some', 'x', 'y', 'etc'] function ArrayInText(str, words) { let regex = new RegExp("\\\\b(" + words.join('|') + ")\\\\b", "g"); return regex.test(str); } console.log(ArrayInText(theString, theArray)); console.log(ArrayInText(theString2, theArray)); console.log(ArrayInText(theString3, theArray));
祝你好運 :)
1) isIncludeWord
-- 使用split
和includes
方法查找精確的單詞匹配。
2) isInclude
- 使用some
和includes
查找匹配包含。
let theString = "include all the information someone would need to answer your question"; let theString2 = "include some the information someone would need to answer your question"; let theString3 = "the information someone would need to answer your question"; let theArray = ["all", "some", "x", "y", "etc"]; const isIncludeWord = (str, arr) => str.split(" ").reduce((include, word) => include || arr.includes(word), false); const isInclude = (str, arr) => arr.some(item => str.includes(item)); console.log(isIncludeWord(theString, theArray)); console.log(isIncludeWord(theString2, theArray)); console.log(isIncludeWord(theString3, theArray)); console.log(isInclude(theString, theArray)); console.log(isInclude(theString2, theArray)); console.log(isInclude(theString3, theArray));
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.