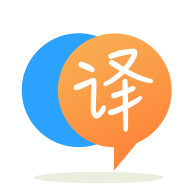
[英]How to download the file using ASP.NET MVC Rest API call and jQuery Ajax
[英]How to call API from view using jQuery and AJAX in an ASP.NET Core MVC project?
我創建了一個 ASP.NET MVC 項目來從列表執行 CRUD 操作,而不是使用數據庫,使用HomeController
和 API 控制器。 現在我想編輯該項目,以便直接從我的 HTML 頁面視圖中調用 API 函數。
控件從視圖傳遞到主控制器,然后傳遞到 API 控制器。 我想要使用 jQuery 和 AJAX 在視圖和 API 之間建立直接連接。
我應該在問題中指定哪些進一步的細節?
<script>
$(document).ready(function () {
$('#login').click(function () {
var x = $('#email1').val()
var y = $('#pswd').val()
$.ajax({
type: "GET",
url: "https://localhost:44347/api/Values/Login/" + x+y,
contentType: "application/json; charset=utf-8",
dataType: "json",
success: function (data) {
window.location.replace("Studentdataproject\Studentdataproject\Views\Home\Details.cshtml");
},
error: function (data) {
alert(data.responseText);
}
});
}); }
這是我在登錄視圖中調用 API 控制器中的登錄的腳本。 它沒有調用 API 控制器。
登錄API:
[HttpGet("Login/{email}/{password}")]
public async System.Threading.Tasks.Task<IActionResult> LoginAsync(string email, string password)
{
obj1 = obj2.Login(email, password);
if (obj1 == null)
{
return Ok(null);
}
else
{
var claims = new List<Claim>()
{
new Claim(ClaimTypes.Name,obj1.email),
new Claim(ClaimTypes.Role,obj1.roles)
};
var identity = new ClaimsIdentity(claims, CookieAuthenticationDefaults.AuthenticationScheme);
var princilple = new ClaimsPrincipal(identity);
await HttpContext.SignInAsync(CookieAuthenticationDefaults.AuthenticationScheme, princilple);
return Ok();
}
}
您需要像這樣在 AJAX 中更改您的 URL:
url: "https://localhost:44347/api/Values/Login/" + x + "/" + y,
使用 AJAX 調用 Web API,類似於調用控制器方法。
下面是使用 AJAX 調用 API 的People
類的 CRUD 操作。 你可以參考一下。
人物類:
public class People
{
[Key]
public int id { get; set; }
public string name { get; set; }
}
接口:
[Route("api/[controller]")]
[ApiController]
public class APICRUDController : ControllerBase
{
private readonly MydbContext _context;
public APICRUDController(MydbContext context)
{
_context = context;
}
[HttpGet("GetPerson")]
public IActionResult GetPerson(int? id)
{
var person = _context.People.ToList();
if (id != null)
{
person = person.Where(x => x.id == id).ToList();
}
return Ok(person);
}
[HttpPost("AddPerson")]
public IActionResult AddPerson([FromBody]People obj)
{
if (!ModelState.IsValid)
{
return Ok("Please enter correct fields!");
}
_context.People.Add(obj);
_context.SaveChanges();
return Ok("Person added successfully!");
}
[HttpPost("UpdatePerson")]
public IActionResult UpdatePerson([FromBody]People obj)
{
People people = (from c in _context.People
where c.id == obj.id
select c).FirstOrDefault();
people.name = obj.name;
_context.SaveChanges();
return Ok();
}
[HttpPost("DeletePerson")]
public void DeletePerson(int Id)
{
People people = (from c in _context.People
where c.id == Id
select c).FirstOrDefault();
_context.People.Remove(people);
_context.SaveChanges();
}
}
查看代碼:
@model WebApplication_core.Models.People @{ ViewData["Title"] = "Index"; Layout = "~/Views/Shared/_Layout.cshtml"; } @section Scripts{ <script> $(function () { loadData(); }) function loadData() { $.ajax({ url: 'https://localhost:44361/api/APICRUD/GetPerson', type: "GET", dataType: "json", success: function (result) { var html = ''; $.each(result, function (key, item) { html += '<tr>'; html += '<td>' + item.id + '</td>'; html += '<td>' + item.name + '</td>'; html += '<td><a href="#" onclick="return Edit(' + item.id + ')">Edit</a> | <a href="#" onclick="Delete(' + item.id + ')">Delete</a></td>'; html += '</tr>'; }); $('.tbody').html(html); }, error: function (errormessage) { alert(errormessage.responseText); } }); } function Add() { var obj = { name: $('#name').val(), }; $.ajax({ type: "POST", url: 'https://localhost:44361/api/APICRUD/AddPerson', contentType: "application/json;charset=utf-8", data: JSON.stringify(obj), success: function (result) { if (result.indexOf("successfully") > -1) { loadData(); $('#myModal').modal('hide'); $('#name').val(""); } alert(result); }, error: function (errormessage) { alert(errormessage.responseText); } }); } function Edit(Id) { $("#myModalLabel").text("Edit Person"); $("#id").parent().show(); $('#name').css('border-color', 'lightgrey'); $.ajax({ url: 'https://localhost:44361/api/APICRUD/GetPerson?id=' + Id, typr: "GET", contentType: "application/json;charset=UTF-8", dataType: "json", success: function (result) { if (result.length > 0) { $('#id').val(result[0].id); $('#name').val(result[0].name); $('#myModal').modal('show'); $('#btnUpdate').show(); $('#btnAdd').hide(); } }, error: function (errormessage) { alert(errormessage.responseText); } }); return false; } function Update() { var obj = { id: parseInt($('#id').val()), name: $('#name').val(), }; $.ajax({ url: 'https://localhost:44361/api/APICRUD/UpdatePerson', data: JSON.stringify(obj), type: "POST", contentType: "application/json;charset=utf-8", success: function () { loadData(); $('#myModal').modal('hide'); $('#id').val(""); $('#name').val(""); }, error: function (errormessage) { alert(errormessage.responseText); } }); } function Delete(Id) { if (confirm("Are you sure you want to delete this Record?")) { $.ajax({ url: 'https://localhost:44361/api/APICRUD/DeletePerson?Id=' + Id, type: "POST", contentType: "application/json;charset=UTF-8", success: function () { alert("delete successfully!"); loadData(); }, error: function (errormessage) { alert(errormessage.responseText); } }); } } function HideKey() { $("#myModalLabel").text("Add Person"); $("#id").parent().hide(); } </script> } <div class="container"> <h2>People Record</h2> <button type="button" class="btn btn-primary" data-toggle="modal" data-target="#myModal" onclick="HideKey()">Add New User</button><br /><br /> <table class="table table-bordered table-hover"> <thead> <tr> <th> ID </th> <th> Name </th> <th> Action </th> </tr> </thead> <tbody class="tbody"></tbody> </table> </div> <div class="modal fade" id="myModal" tabindex="-1" role="dialog" aria-labelledby="myModalLabel" aria-hidden="true"> <div class="modal-dialog"> <div class="modal-content"> <div class="modal-header"> <button type="button" class="close" data-dismiss="modal">×</button> <h4 class="modal-title" id="myModalLabel"></h4> </div> <div class="modal-body"> <form> <div class="form-group"> <label asp-for="id"></label> <input asp-for="id" class="form-control" disabled /> </div> <div class="form-group"> <label asp-for="name"></label> <input asp-for="name" class="form-control" /> <span class="field-validation-valid text-danger" asp-validation-for="name"></span> </div> </form> </div> <div class="modal-footer"> <button type="button" class="btn btn-primary" id="btnAdd" onclick="return Add();">Add</button> <button type="button" class="btn btn-primary" id="btnUpdate" style="display:none;" onclick="Update();">Update</button> <button type="button" class="btn btn-default" data-dismiss="modal">Close</button> </div> </div> </div> </div>
這是這個演示的結果:
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.