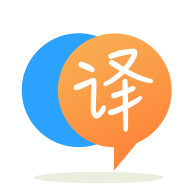
[英]How do I compare user integer input to values in dictionary? (Python)
[英]How do I sort list in python with user input and values between 1 and 100 and no repeating values?
我想制作一個程序,以升序對數字列表進行排序,值在 1 到 100 之間。這就是我所做的,如果您運行代碼,列表會打印多次並且沒有正確指出錯誤:
# This is the user input
a = int(input("Enter the first number: "))
b = int(input("Enter the second number: "))
c = int(input("Enter the third number: "))
d = int(input("Enter the fourth number: "))
e = int(input("Enter the fifth number: "))
f = int(input("Enter the sixth number: "))
# This the list to sort
array = [a, b, c, d, e, f]
#This will count how many time each value is repeated
aa = int(array.count(a))
bb = int(array.count(b))
cc = int(array.count(c))
dd = int(array.count(d))
ee = int(array.count(e))
ff = int(array.count(f))
# This will loop through the list
for i in array:
# This is checking if any values are repeated or not between 1 and 100
if i > 100 or i < 1 or aa and bb and cc and dd and ee and ff > 1:
print("Number should be smaller than 100 and larger than 1 and numbers can't be repeated")
# This is to print the sorted list if you get everything right
else:
array.sort()
print(array)
我認為您想用if...aa and bb and cc and dd and ee and ff > 1:
的or
語句替換您的and
語句。 否則,僅當所有數字都重復時,條件才會為True
。
if i > 100 or i < 1 or aa or bb or cc or dd or ee or ff > 1:
注意:這將打印Number should be smaller than 100 and larger than 1 and numbers can't be repeated
6 次,這是您真正想要的嗎?
否則,有一種更短的方法可以做您(可能)想做的事情:
array
轉換為一個set
(只保留唯一值)並比較它們的長度嘗試這個 :
# This is the user input
a = int(input("Enter the first number: "))
b = int(input("Enter the second number: "))
c = int(input("Enter the third number: "))
d = int(input("Enter the fourth number: "))
e = int(input("Enter the fifth number: "))
f = int(input("Enter the sixth number: "))
# This the list to sort
array = [a, b, c, d, e, f]
if max(array)>100 or min(array)<1 or len(set(array)) != len(array):
print("Number should be smaller than 100 and larger than 1 and numbers can't be repeated")
else:
array.sort()
print(array)
替代方法:使用集合來收集您正在接受的號碼,並使用它來拒絕已經存在的號碼
我知道 StackOverflow 的本意不是給別人寫代碼,但是我想推薦給你的很多東西,我只能用代碼來表達。 在這里,我認為你可以通過分析來學習一些東西。
(它需要 Python 3.8 或更高版本):
accepted_numbers = set() # Begin with an empty bag
## Main Job ##
def main():
for i in range(1, 6+1):
while not satisfy_conditions(number := get_ith_number(i)):
notify_error()
accepted_numbers.add(number)
report()
## END Main Job ##
## Support ##
def satisfy_conditions(number):
# Returns True if the number is not in the bag and is between 1 and 100
return number not in accepted_numbers and (1 <= number <= 100)
def get_ith_number(i):
word_number = {1:'first', 2:'second', 3:'third', 4:'forth', 5:'five', 6:'sixth'}
return int(input(f'Enter the {word_number[i]} number: '))
def notify_error():
print("Number should be smaller than 100 and larger than 1 and numbers can't be repeated")
def report():
print(sorted(accepted_numbers))
## END Support ##
if __name__ == '__main__':
main()
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.