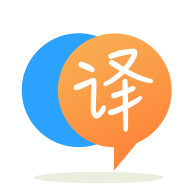
[英]NetworkStream callback called multiple times on server when client closes connection
[英]SignalR server closes connection immediately when the client connects
我想在項目中使用 SignalR 向設備發送重啟消息,但我不能。 所以我決定開始一個新的解決方案,僅用於測試目的,結果相同:
SocketException (0x80004005): 現有連接被遠程主機強行關閉
我正在使用帶有 .NET 4.7.2 的 Visual Studio Professional 2017,這些是我遵循的步驟:
SignalRServer
ASP.NET Web App 項目創建了一個新解決方案,使用SignalRServer
進行身份驗證。SignalR
新文件夾並添加新項目 > SignalR Hub Class (v2)。 我將它命名為SignalRHub
:public class SignalRHub : Hub
{
public void Reboot()
{
Clients.All.reboot();
}
public void Hello()
{
Clients.All.hello();
}
public override Task OnConnected()
{
Debug.WriteLine($"[SERVER] {this.Context.ConnectionId} connected");
return base.OnConnected();
}
public override Task OnReconnected()
{
Debug.WriteLine($"[SERVER] {this.Context.ConnectionId} reconnected");
return base.OnReconnected();
}
public override Task OnDisconnected(bool stopCalled)
{
Debug.WriteLine($"[SERVER] {this.Context.ConnectionId} disconnected");
return base.OnDisconnected(stopCalled);
}
}
public partial class Startup
{
public void Configuration(IAppBuilder app)
{
app.MapSignalR();
ConfigureAuth(app);
}
}
服務器實現完成。 之后,客戶端:
SignalRClient
的新 WPF 項目。Microsoft.AspNet.SignalR.Client
包。MainWindow.xaml
:<Window x:Class="SignalRClient.MainWindow"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:d="http://schemas.microsoft.com/expression/blend/2008"
xmlns:mc="http://schemas.openxmlformats.org/markup-compatibility/2006"
xmlns:local="clr-namespace:SignalRClient"
mc:Ignorable="d"
Title="MainWindow" Height="300" Width="400" Loaded="Window_Loaded">
<Grid>
<Button VerticalAlignment="Center" HorizontalAlignment="Center" Padding="5" Content="Send message!" Click="Button_Click"/>
</Grid>
</Window>
MainWindow.xaml.cs
:public partial class MainWindow : Window
{
private IHubProxy signalRHubProxy;
public MainWindow()
{
InitializeComponent();
}
private async void Window_Loaded(object sender, RoutedEventArgs e)
{
try
{
using (var hubConnection = new HubConnection("http://localhost:54475/"))
{
this.signalRHubProxy = hubConnection.CreateHubProxy("SignalRHub");
this.signalRHubProxy.On("Hello", this.HelloMessageReceived);
this.signalRHubProxy.On("Reboot", this.RebootMessageReceived);
ServicePointManager.DefaultConnectionLimit = 10;
await hubConnection.Start();
Debug.WriteLine($"[CLIENT] Connected to hub");
}
}
catch (Exception exception)
{
Debug.WriteLine($"[CLIENT] {exception.Message}");
}
}
private void HelloMessageReceived()
{
Console.WriteLine("[CLIENT] Hello message received");
}
private void RebootMessageReceived()
{
Console.WriteLine("[CLIENT] Reboot message received");
}
private async void Button_Click(object sender, RoutedEventArgs e)
{
await this.signalRHubProxy.Invoke("Hello", new { });
}
}
客戶端也完成了。 現在我首先運行 Web 應用程序,當加載主頁時,我運行 WPF 應用程序(調試 > 啟動新實例)。 這是輸出:
[SERVER] b3f11c77-6bfc-416a-914b-f2573f0cc42c connected
[CLIENT] Connected to hub
[SERVER] b3f11c77-6bfc-416a-914b-f2573f0cc42c disconnected
SignalRClient.exe Error: 0 : Error while closing the websocket: System.Net.WebSockets.WebSocketException (0x80004005): An internal WebSocket error occurred. Please see the innerException, if present, for more details. ---> System.Net.Sockets.SocketException (0x80004005): An existing connection was forcibly closed by the remote host
at System.Net.WebSockets.WebSocketConnectionStream.WebSocketConnection.WriteAsync(Byte[] buffer, Int32 offset, Int32 count, CancellationToken cancellationToken)
at System.Net.DelegatedStream.WriteAsync(Byte[] buffer, Int32 offset, Int32 count, CancellationToken cancellationToken)
at System.Net.WebSockets.WebSocketConnectionStream.<>n__1(Byte[] buffer, Int32 offset, Int32 count, CancellationToken cancellationToken)
at System.Net.WebSockets.WebSocketConnectionStream.<WriteAsync>d__22.MoveNext()
--- End of stack trace from previous location where exception was thrown ---
at System.Runtime.CompilerServices.TaskAwaiter.ThrowForNonSuccess(Task task)
at System.Runtime.CompilerServices.TaskAwaiter.HandleNonSuccessAndDebuggerNotification(Task task)
at System.Net.WebSockets.WebSocketBase.<SendFrameAsync>d__48.MoveNext()
--- End of stack trace from previous location where exception was thrown ---
at System.Runtime.CompilerServices.TaskAwaiter.ThrowForNonSuccess(Task task)
at System.Runtime.CompilerServices.TaskAwaiter.HandleNonSuccessAndDebuggerNotification(Task task)
at System.Runtime.CompilerServices.TaskAwaiter.ValidateEnd(Task task)
at System.Net.WebSockets.WebSocketBase.WebSocketOperation.<Process>d__19.MoveNext()
--- End of stack trace from previous location where exception was thrown ---
at System.Runtime.CompilerServices.TaskAwaiter.ThrowForNonSuccess(Task task)
at System.Runtime.CompilerServices.TaskAwaiter.HandleNonSuccessAndDebuggerNotification(Task task)
at System.Net.WebSockets.WebSocketBase.<CloseOutputAsyncCore>d__51.MoveNext()
at System.Net.WebSockets.WebSocketBase.ThrowIfConvertibleException(String methodName, Exception exception, CancellationToken cancellationToken, Boolean aborted)
at System.Net.WebSockets.WebSocketBase.<CloseOutputAsyncCore>d__51.MoveNext()
--- End of stack trace from previous location where exception was thrown ---
at System.Runtime.CompilerServices.TaskAwaiter.ThrowForNonSuccess(Task task)
at System.Runtime.CompilerServices.TaskAwaiter.HandleNonSuccessAndDebuggerNotification(Task task)
at Microsoft.AspNet.SignalR.WebSockets.WebSocketHandler.<>c.<<CloseAsync>b__13_0>d.MoveNext() in /_/src/Microsoft.AspNet.SignalR.Core/Owin/WebSockets/WebSocketHandler.cs:line 114
The thread 0x4b44 has exited with code 0 (0x0).
The thread 0x5560 has exited with code 0 (0x0).
我可以看到客戶端連接到服務器,但連接立即關閉。 我不知道我是不是忘記了一步。 我以相同的結果停止了防火牆。 你知道我該如何解決這個問題嗎?
似乎using
語句是原因,因為它在try
塊的末尾處理創建的HubConnection
對象,這導致 websocket 關閉。
您可以考慮刪除using
語句並將hubConnection
保存為字段或屬性,以便稍后調用hubConnection.Stop()
以斷開連接。
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.