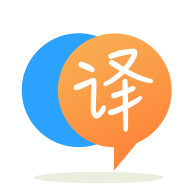
[英]C++: How can I avoid “invalid covariant return type” in inherited classes without casting?
[英]C++ - How to return list of covariant classes?
我正在處理 Qt + C++ (x11)。
我有一個基類和幾個返回指向該子類(協變)的新指針的子類。 我也需要返回這些子類的容器(QList)。 一個例子:
class A
{
public:
int id;
}
class B : public A
{
int Age;
};
class WorkerA
{
public:
virtual A *newOne() {return new A()};
virtual QList<A*> *newOnes {
QList<A*> list = new QList<A*>;
//Perform some data search and insert it in list, this is only simple example. In real world it will call a virtual method to fill member data overriden in each subclass.
A* a = this.newOne();
a.id = 0;
list.append(this.newOne());
return list;
};
};
class WorkerB
{
public:
virtual B *newOne() override {return new B()}; //This compiles OK (covariant)
virtual QList<B*> *newOnes override { //This fails (QList<B*> is not covariant of QList<A*>)
(...)
};
};
這將無法編譯,因為 QList 是與 QList 完全不同的類型。 但類似的東西會很好。 在現實世界中,B 的數據成員將比 A 多,並且會有 C、D...,因此需要“協變”列表的返回。 我會更好:
WorkerB wb;
//some calls to wb...
QList<B*> *bList = wb.newOnes();
B* b = bList.at(0); //please excuse the absence of list size checking
info(b.id);
info(b.age);
比
WorkerB wb;
//some calls to wb...
QList<A*> *bList = wb.newOnes();
B* b = static_cast<B*>(bList.at(0)); //please excuse the absence of list size checking
info(b.id);
info(b.age);
有沒有辦法實現這一目標?
我希望從下面提到的代碼中你會得到一些關於這個問題的提示。
這是 main.cpp:
#include <QCoreApplication>
#include <QDebug>
#include "myclass.h"
int main(int argc, char *argv[])
{
QCoreApplication a(argc, argv);
MyClass mClass;
mClass.name = "Debussy";
// put a class into QVariant
QVariant v = QVariant::fromValue(mClass);
// What's the type?
// It's MyClass, and it's been registered
// by adding macro in "myclass.h"
MyClass vClass = v.value<MyClass>();
qDebug() << vClass.name;
return a.exec();
}
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.