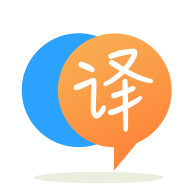
[英]Problem with my for loop, finding the shortest path length of a subpath using networkx
[英]What is wrong with my function? Finding the shortest path using numpy and networkx
所以我正在創建一個代碼來使用 networkx 計算最短路徑。 我使用 numpy 創建了一個 3D 數組,然后像這樣計算最短路徑:
import numpy as np
import networkx as nx
arr = np.random.randint(1, 100, size = (2, 5, 5)) #3D array
for i in arr:
graph = nx.from_numpy_array(i, create_using = nx.DiGraph)
path = nx.shortest_path(graph, 0, 3, weight = 'weight')
print(path)
因為我使用了兩個矩陣,所以我得到了下一個輸出:
[0, 1, 3] #path1
[0, 3] #path2
之后,我決定創建一個函數來做完全相同的事情,如下所示:
import numpy as np
import networkx as nx
arr = np.random.randint(1, 100, size = (2, 5, 5)) #3D array
def shortest(prices):
for i in arr:
graph = nx.from_numpy_array(i, create_using = nx.DiGraph)
path = nx.shortest_path(graph, 0, 3, weight = 'weight')
return path
print(shortest(arr))
我得到了下一個輸出:
[0, 1, 3] #same as path 1
如果我像這樣改變“返回路徑”的位置:
def shortest(precios):
for i in arr:
graph = nx.from_numpy_array(i, create_using = nx.DiGraph)
path = nx.shortest_path(graph, 0, 3, weight = 'weight')
return path
print(shortest(arr))
我得到了下一個輸出:
[0, 3] #same as path 2
我無法使用我的函數在同一輸出中獲得兩條路徑。 知道這里發生了什么嗎? 我正在練習在 python 中使用函數,因為我對這個主題有點陌生,所以我希望你能幫我看看有什么問題? 謝謝!
這與networkx
或numpy
無關; 這是簡單的控制流程。 您的“最短路徑”循環遍歷提供的圖形並為每個圖形找到最短路徑。
非函數版本在找到時打印每個路徑——仍然一次只處理一個。 由於您沒有在函數中設置聚合找到的解決方案,因此一次仍然只能獲得一個……這對於函數來說意味着您只能獲得一個。
此代碼在找到第一個解決方案后立即返回:
for i in arr:
graph = nx.from_numpy_array(i, create_using = nx.DiGraph)
path = nx.shortest_path(graph, 0, 3, weight = 'weight')
return path # The function ends as soon as it gets here: you get only the first solution.
這段代碼找到了所有的解決方案,但你一找到下一個就扔掉每一個:
for i in arr:
graph = nx.from_numpy_array(i, create_using = nx.DiGraph)
path = nx.shortest_path(graph, 0, 3, weight = 'weight')
# You just deleted the previous solution, and replaced it with another.
return path
這僅返回最后一個解決方案,因為這是您唯一沒有覆蓋的解決方案。 您必須以某種方式累積解決方案並將它們一起返回,或者更改您的函數用法。 例如:
all_path = []
for i in arr:
graph = nx.from_numpy_array(i, create_using = nx.DiGraph)
all_path.append( nx.shortest_path(graph, 0, 3, weight = 'weight') )
return all_path
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.