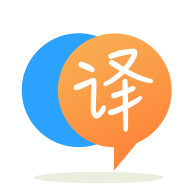
[英]Reading text file line by line in Python and storing into struct as coordinates for graphing
[英]graphing a line of data from a text file
這是我第一次在 python 上創建圖形。 我有一個文本文件,其中包含“每周天然氣平均值”的數據。 其中有 52 個(這是一年的數據)。 我了解如何讀取數據並將其制成列表,我想,如果我自己提出要點,我可以做制作圖表的基礎知識。 但是我不知道如何將兩者連接起來,因為將文件中的數據轉換為我的X軸,然后制作我自己的Y軸(1-52)。 我的代碼是我慢慢組合起來的一堆想法。 任何幫助或指導將是驚人的。
import matplotlib.pyplot as plt
def main():
print("Welcome to my program. This program will read data
off a file"\
+" called 1994_Weekly_Gas_Averages.txt. It will plot the"\
+" data on a line graph.")
print()
gasFile = open("1994_Weekly_Gas_Averages.txt", 'r')
gasList= []
gasAveragesPerWeek = gasFile.readline()
while gasAveragesPerWeek != "":
gasAveragePerWeek = float(gasAveragesPerWeek)
gasList.append(gasAveragesPerWeek)
gasAveragesPerWeek = gasFile.readline()
index = 0
while index<len(gasList):
gasList[index] = gasList[index].rstrip('\n')
index += 1
print(gasList)
#create x and y coordinates with data
x_coords = [gasList]
y_coords = [1,53]
#build line graph
plt.plot(x_coords, y_coords)
#add title
plt.title('1994 Weekly Gas Averages')
#add labels
plt.xlabel('Gas Averages')
plt.ylabel('Week')
#display graph
plt.show()
main()
在閱讀您的代碼時,我可以發現兩個錯誤:
gasList
已經是一個列表,因此當您編寫x_coords = [gasList]
您正在創建一個列表列表,這將不起作用y_coords=[1,53]
創建了一個只有 2 個值的列表:1 和 53。繪圖時,您需要有與 x 值一樣多的 y 值,因此該列表中應該有 52 個值. 您不必全部手動編寫它們,您可以使用函數range(start, stop)
為您完成此操作話雖如此,通過使用已經為您編寫的函數,您可能會收獲很多。 例如,如果您使用模塊numpy
( import numpy as np
),那么您可以使用np.loadtxt()
讀取文件的內容並在一行中創建一個數組。 嘗試自己解析文件會更快,並且更不容易出錯。
最終代碼:
import matplotlib.pyplot as plt
import numpy as np
def main():
print(
"Welcome to my program. This program will read data off a file called 1994_Weekly_Gas_Averages.txt. It will "
"plot the data on a line graph.")
print()
gasFile = "1994_Weekly_Gas_Averages.txt"
gasList = np.loadtxt(gasFile)
y_coords = range(1, len(gasList) + 1) # better not hardcode the length of y_coords,
# in case there fewer values that expected
# build line graph
plt.plot(gasList, y_coords)
# add title
plt.title('1994 Weekly Gas Averages')
# add labels
plt.xlabel('Gas Averages')
plt.ylabel('Week')
# display graph
plt.show()
if __name__ == "__main__":
main()
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.