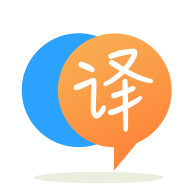
[英]Configuring multiple 1 to 0..1 relationships between tables entity framework
[英]Relationships between three tables with Entity Framework
我想在三個表之間建立關系。
我的數據庫如下所示: https://ibb.co/VggWGRb
這些是我的課程:
public class Equipment // model for Equipment table
{
public int EquipmentID { get; set; }
public IEnumerable<string> Item { get; set; }
}
public class Car // model for car table
{
public int CarID { get; set; }
public string Brand { get; set; }
public string Model { get; set; }
public int Price { get; set; }
public string Category { get; set; }
public string Description { get; set; }
public int Production { get; set; }
public int Mileage { get; set; }
public byte[] ImageData { get; set; }
public string ImageMimeType { get; set; }
}
public class CarEquipment // model for carequipment table
{
public int CarID { get; set; }
public int EquipmentID { get; set; }
public virtual Car Car { get; set; }
public virtual ICollection<Equipment> Equipment { get; set; }
}
public class EfDbContext : DbContext
{
public DbSet<Car> Cars { get; set; }
public DbSet<Equipment> Equipments { get; set; }
public DbSet<CarEquipment> CarEquipments { get; set; }
}
我找到了一些解決方案,有人使用context.Guild.Include("Player").ToList();
也許我應該做這樣的事情?
public class EFCarEquipmentRepository : ICarEquipmentRepository
{
private EfDbContext context = new EfDbContext();
public IEnumerable<CarEquipment> CarEquipment
{
get
{
return context.CarEquipments;
}
}
}
public interface ICarEquipmentRepository
{
IEnumerable<CarEquipment> CarEquipment { get; set; }
}
應該如何正確書寫以及如何使用 controller 中的IEnumerable<string> equipment
調用汽車 model?
根據問題圖像中顯示的 ER 圖,您可以在 EF 中定義關系,如下所示,
public class Equipment // model for Equipment table
{
public int EquipmentID { get; set; }
public IEnumerable<string> Item { get; set; }
public virtual ICollection<CarEquipment> CarEquipments { get; set; }
}
public class Car // model for car table
{
public int CarID { get; set; }
public string Brand { get; set; }
public string Model { get; set; }
public int Price { get; set; }
public string Category { get; set; }
public string Description { get; set; }
public int Production { get; set; }
public int Mileage { get; set; }
public byte[] ImageData { get; set; }
public string ImageMimeType { get; set; }
public virtual ICollection<CarEquipment> CarEquipments { get; set; }
}
public class CarEquipment // model for carequipmeHnt table
{
public int CarID { get; set; }
public int EquipmentID { get; set; }
public virtual Car Car { get; set; }
public virtual Equipment Equipment { get; set; }
}
public class EfDbContext : DbContext
{
public DbSet<Car> Cars { get; set; }
public DbSet<Equipment> Equipments { get; set; }
public DbSet<CarEquipment> CarEquipments { get; set; }
protected override void OnModelCreating(ModelBuilder modelBuilder)
{
modelBuilder.Entity<Car>().HasKey(x => x.CarID);
modelBuilder.Entity<CarEquipment>().HasKey(x => new { x.CarID, x.EquipmentID });
modelBuilder.Entity<Equipment>().HasKey(x => x.EquipmentID);
modelBuilder.Entity<CarEquipment>().HasOne(x => x.Equipment).WithMany(x => x.CarEquipments);
modelBuilder.Entity<CarEquipment>().HasOne(x => x.Car).WithMany(x => x.CarEquipments);
}
}
現在你可以像這樣訪問 CarEquipment 和 Car,
public class EFCarEquipmentRepository : ICarEquipmentRepository
{
private EfDbContext context = new EfDbContext();
public IEnumerable<CarEquipment> CarEquipment
{
get
{
return context.CarEquipments.Include(x => x.Car);
}
}
}
如果你也想訪問設備,只需像這樣鏈接方法,
context.CarEquipments.Include(x => x.Car).Include(x => x.Equipment)
注意:我已經解釋了這一點,保持 EF Core 為參考。 這是可以學習如何在 EF Core 中定義關系的最佳來源之一: MS Doc
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.