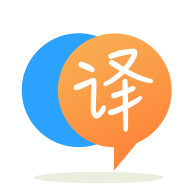
[英]I've done a program to display student name and height using queue linked list in C++ now i want to display it in Desceding order i dont know how
[英]Program to input Name and Height and Display it. I can't figure out how to display the name and Height of the tallest person in the list
void insertname()
{
while (true)
{
char dec;
nodename* temp;
temp = new nodename;
std::cout << "ENTER YOUR NAME : ";
std::cin >> temp->name;
std::cout << "ENTER YOUR HEIGHT : ";
std::cin >> temp->height;
std::cout << '\n';
temp->next = NULL;
if (rear == NULL)
{
rear = temp;
front = temp;
}
else
{
rear->next = temp;
rear = temp;
}
std::cout << "ADD ANOTHER DATA? (Y/N) : ";
std::cin >> dec;
std::cout << '\n';
if (dec == 'n' || dec == 'N')
{
break;
}
}
}
void display()
{
nodename* temp = front;
while (temp != NULL)
{
std::cout << "--------------------------" << '\n';
std::cout << "NAME : " << temp->name << endl;
std::cout << showpoint << fixed << setprecision(0);
std::cout << "HEIGHT : " << temp->height << endl;
std::cout << showpoint << fixed << setprecision(2);
temp = temp->next;
std::cout << "--------------------------" << '\n';
}
}
這使用帶隊列的鏈接列表來顯示我已經完成的姓名和身高,但我想不出一種方法來顯示 output 以便它顯示列表中最高人的姓名和身高。 對於程序中的任何錯誤,我深表歉意,我對編程還比較陌生,而且我還在學習。 我真的很感激幫助
據我了解,您正在尋找的是 STL 庫排序 function,您可以簡單地使用:
std::sort(arr, sizeof(arr)/sizeof(arr[0]), greater<int>());
按降序對任何數組進行排序。
void insertname()
{
while (true)
{
char dec;
nodename* temp;
temp = new nodename;
std::cout << "ENTER YOUR NAME : ";
std::cin >> temp->name;
std::cout << "ENTER YOUR HEIGHT : ";
std::cin >> temp->height;
std::cout << '\n';
temp->next = NULL;
if (rear == NULL)
{
rear = temp;
front = temp;
}
else
{
rear->next = temp;
rear = temp;
}
std::cout << "ADD ANOTHER DATA? (Y/N) : ";
std::cin >> dec;
std::cout << '\n';
if (dec == 'n' || dec == 'N')
{
break;
}
}
}
void display()
{
nodename* temp = front;
while (temp != NULL)
{
std::cout << "--------------------------" << '\n';
std::cout << "NAME : " << temp->name << endl;
std::cout << showpoint << fixed << setprecision(0);
std::cout << "HEIGHT : " << temp->height << endl;
std::cout << showpoint << fixed << setprecision(2);
temp = temp->next;
std::cout << "--------------------------" << '\n';
}
}
這使用帶有隊列的鏈接列表來顯示我已經完成的名稱和高度,但我無法找到顯示 output 的方法,以便它顯示列表中最高人的名稱和高度。 對於程序中的任何錯誤,我很抱歉,我對編程比較陌生,我還在學習。 我真的很感激幫助
您不需要鏈接列表,您可以使用滾動最大值:
std::string name;
std::string name_tallest;
int height = 0;
int tallest_height = 0;
static const char name_prompt[] = "Enter your name:\n";
static const char height_prompt[] = "Enter your height: ";
std::cout.write(name_prompt, sizeof(name_prompt) - 1);
while (std::cin >> name)
{
std::cout.write(height_prompt, sizeof(height_prompt) - 1);
if (std::cin >> height)
{
if (height > tallest_height)
{
tallest_height = height;
name_tallest = name;
}
}
}
std::cout << "Name of tallest person: " << name_tallest << "\n";
std::cout << "Height: " << tallest_height << "\n";
如果要使用隊列,請使用std::queue
。
對於列表,請使用std::list
。
學習鏈表,把你的鏈表代碼放到一個單獨的文件中。 最好不要將鏈表代碼與隊列代碼或main
代碼混合使用。
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.