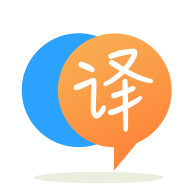
[英]Adding Children to StackPanel in Parent Window from UserControl in WPF/C#
[英]In WPF, adding UserControl C# object from ViewModel to View
我在 ViewModel 中有一個 UserControl,它是 ViewModel 中的一個屬性。 我將從一些 dll 中獲取此 UserControl。
private GraphicControl _graphicCtrl;
public GraphicControl GraphicCtrl
{
get { return _graphicCtrl; }
set
{
_graphicCtrl = value;
NotifyPropertyChanged();
}
}
在上面的屬性中, GraphicCtrl只不過是一個UserControl 。 我想在我的視圖 (xaml) 中顯示它。 那么我應該如何實現呢?
這是一個非常簡單的示例,如何將其分開
public class MainViewModel : ReactiveObject
{
public MainViewModel()
{
Stuff = new ObservableCollection<object>
{
new Person { FirstName = "Jon", LastName="Doe", },
new Car { Brand = "Ford", Model = "Model T" },
};
}
public IEnumerable Stuff { get; }
[Reactive] public object SelectedStuff { get; set; }
}
public class Person
{
public string FirstName { get; set; }
public string LastName { get; set; }
}
public class Car
{
public string Brand { get; set; }
public string Model { get; set; }
}
如您所見,不依賴於任何控件或其他 UI 相關的東西。
現在是我決定如何呈現來自 ViewModel 的數據的視圖
<Grid>
<Grid.ColumnDefinitions>
<ColumnDefinition Width="200" />
<ColumnDefinition />
</Grid.ColumnDefinitions>
<ListBox
ItemsSource="{Binding Stuff}"
SelectedItem="{Binding SelectedStuff}">
<ListBox.Resources>
<DataTemplate DataType="{x:Type local:Car}">
<TextBlock>
<Run Text="{Binding Brand}" /><Run Text=" - " /><Run Text="{Binding Model}" />
</TextBlock>
</DataTemplate>
<DataTemplate DataType="{x:Type local:Person}">
<TextBlock>
<Run Text="{Binding FirstName}" /> <Run Text="{Binding LastName}" />
</TextBlock>
</DataTemplate>
</ListBox.Resources>
<ListBox.ItemTemplate>
<DataTemplate>
<ContentPresenter Content="{Binding}" />
</DataTemplate>
</ListBox.ItemTemplate>
</ListBox>
<ContentControl
Grid.Column="1"
Content="{Binding SelectedStuff}">
<ContentControl.Resources>
<DataTemplate DataType="{x:Type local:Car}">
<local:CarControl/>
</DataTemplate>
<DataTemplate DataType="{x:Type local:Person}">
<local:PersonControl/>
</DataTemplate>
</ContentControl.Resources>
</ContentControl>
</Grid>
汽車控制
<StackPanel>
<Label Content="Car" />
<Label Content="Brand" />
<TextBlock Text="{Binding Brand}" />
<Label Content="Model" />
<TextBlock Text="{Binding Model}" />
</StackPanel>
PersonControl
<StackPanel>
<Label Content="Person" />
<Label Content="FirstName" />
<TextBlock Text="{Binding FirstName}" />
<Label Content="LastName" />
<TextBlock Text="{Binding LastName}" />
</StackPanel>
最后是截圖
我想在我的視圖 (xaml) 中顯示它。 那么我應該如何實現呢?
簡短回答:使用ContentControl
並將其Content
屬性綁定到UserControl
:
<ContentControl Content="{Binding GraphicCtrl}" />
正如其他人已經提到的,從視圖 model 公開UserControl
不是 MVVM。
您應該公開一個名為Graphic
之類的 POCO 數據 object,然后將DataTemplate
用於 map 這種類型的視圖中的UserControl
:
<ContentControl Content="{Binding Graphic}">
<ContentControl.ContentTemplate>
<DataTemplate>
<local:GraphicControl />
</DataTemplate>
</ContentControl.ContentTemplate>
</ContentControl>
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.