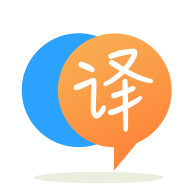
[英]How to randomly return the index of one of the maximum elements in a list in Python?
[英]python :return the maximum of the first n elements in a list
我有一個列表,需要在列表a
中找到兩個列表,分別跟蹤最大值/最小值。
在某些不需要循環的 package 或numpy
中是否存在 function? 我需要加速我的代碼,因為我的數據集很大。
a=[4,2,6,5,2,6,9,7,10,1,2,1]
b=[];c=[];
for i in range(len(a)):
if i==0:
b.append(a[i])
elif a[i]>b[-1]:
b.append(a[i])
for i in range(len(a)):
if i==0:
c.append(a[i])
elif a[i]<c[-1]:
c.append(a[i])
#The output should be a list :
b=[4,6,9,10];c=[4,2,1]
既然您說您正在處理一個非常大的數據集,並且希望避免使用循環,那么也許這是一個潛在的解決方案,它將循環保持在最低限度:
def while_loop(a):
b = [a[0]]
c = [a[0]]
a = np.array(a[1:])
while a.size:
if a[0] > b[-1]:
b.append(a[0])
elif a[0] < c[-1]:
c.append(a[0])
a = a[(a > b[-1]) | (a < c[-1])]
return b, c
編輯:
def for_loop(a):
b = [a[0]]
c = [a[0]]
for x in a[1:]:
if x > b[-1]:
b.append(x)
elif x < c[-1]:
c.append(x)
return b, c
print(
timeit(lambda: while_loop(np.random.randint(0, 10000, 10000)), number=100000)
) # 27.847886939000002
print(
timeit(lambda: for_loop(np.random.randint(0, 10000, 10000)), number=100000)
) # 112.90950811199998
好的,所以我只是檢查了常規 for 循環的時間,而 while 循環似乎快了 4-5 倍。 但不能保證,因為這似乎很大程度上取決於數據集的結構(見評論)。
首先,您可以簡單地使用 a 的第a
元素初始化b
和c
。 這簡化了循環(您只需要 1 個):
a = [...]
b = [a[0]]
c = [a[0]]
for x in a[1:]:
if x > b[-1]:
b.append(x)
elif x < c[-1]:
c.append(x)
請注意,在循環內部, x
的值不能既大於當前最大值又小於當前最小值,因此使用elif
而不是兩個單獨的if
語句。
另一個優化是兩個使用額外的變量來避免索引b
和c
重復,以及一個顯式迭代器來避免對a
進行淺拷貝。
a = [...]
a_iter = iter(a) curr_min = curr_max = next(a_iter)
b = [curr_max]
c = [curr_min]
for x in a_iter:
if x > curr_max:
b.append(x)
curr_max = x
elif x curr_min:
c.append(x)
curr_min = x
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.