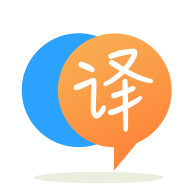
[英]How to add an ActionListener while adding an Instantiation of a JComponent to a JContainer?
[英]How do I add an ActionListener to a specific JComponent?
我正在嘗試為特定的JComponent
創建一個ActionListener
,但在我的代碼中,有兩個JComponent
具有ActionListener
。
這是我的代碼:
/**
* This is a Java Swing program that lets you play Minesweeper! Make sure to play it in fullscreen or else it's not going to work.
* @author Joshua Diocares
* @version JDK14.0
* @since Still in development!
*/
import java.awt.Color;
import java.awt.Cursor;
import java.awt.Font;
import java.awt.Graphics;
import java.awt.event.ActionListener;
import java.awt.event.MouseEvent;
import java.awt.event.MouseMotionAdapter;
import javax.swing.JButton;
import javax.swing.JComboBox;
import javax.swing.JComponent;
import javax.swing.JFrame;
import javax.swing.JLabel;
import javax.swing.JLayeredPane;
import javax.swing.JPanel;
/**
* A class that shows the xy coordinates near the mouse cursor.
*/
class AlsXYMouseLabelComponent extends JComponent {
public int x;
public int y;
/**
* Uses the xy coordinates to update the mouse cursor label.
*/
@Override
public void paintComponent(Graphics g) {
super.paintComponent(g);
String coordinates = x + ", " + y; // Get the cordinates of the mouse
g.setColor(Color.red);
g.drawString(coordinates, x, y); // Display the coordinates of the mouse
}
}
public class Minesweeper implements ActionListener {
private static JComboBox < String > gameModeDropdown;
private static JComboBox < String > difficultyDropdown;
public static void main(String[] args) {
JPanel panel = new JPanel();
JFrame frame = new JFrame();
frame = new JFrame();
frame.setSize(1365, 767);
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.setTitle("Tic Tac Toe");
frame.setCursor(new Cursor(Cursor.CROSSHAIR_CURSOR));
frame.setVisible(true);
frame.add(panel);
panel.setLayout(null);
AlsXYMouseLabelComponent alsXYMouseLabel = new AlsXYMouseLabelComponent();
/**
* Add the component to the DRAG_LAYER of the layered pane (JLayeredPane)
*/
JLayeredPane layeredPane = frame.getRootPane().getLayeredPane();
layeredPane.add(alsXYMouseLabel, JLayeredPane.DRAG_LAYER);
alsXYMouseLabel.setBounds(0, 0, frame.getWidth(), frame.getHeight());
/**
* Add a mouse motion listener, and update the crosshair mouse cursor with the xy coordinates as the user moves the mouse
*/
frame.addMouseMotionListener(new MouseMotionAdapter() {
/**
* Detects when the mouse moved and what the mouse's coordinates are.
* @param event the event that happens when the mouse is moving.
*/
@Override
public void mouseMoved(MouseEvent event) {
alsXYMouseLabel.x = event.getX();
alsXYMouseLabel.y = event.getY();
alsXYMouseLabel.repaint();
}
});
JLabel title = new JLabel("Minesweeper");
title.setBounds(500, 100, 550, 60);
title.setFont(new Font("Verdana", Font.PLAIN, 50));
panel.add(title);
JLabel gameModePrompt = new JLabel("Game Mode: ");
gameModePrompt.setBounds(280, 335, 300, 25);
gameModePrompt.setFont(new Font("Verdana", Font.PLAIN, 20));
panel.add(gameModePrompt);
String[] gameModes = {
"Normal"
};
JComboBox < String > gameModeDropdown = new JComboBox < String > (gameModes);
gameModeDropdown.setBounds(415, 335, 290, 25);
gameModeDropdown.setFont(new Font("Verdana", Font.PLAIN, 20));
gameModeDropdown.addActionListener(new Minesweeper());
panel.add(gameModeDropdown);
JLabel difficultyPrompt = new JLabel("Difficulty: ");
difficultyPrompt.setBounds(800, 335, 240, 25);
difficultyPrompt.setFont(new Font("Verdana", Font.PLAIN, 20));
panel.add(difficultyPrompt);
String[] difficulties = {
"Medium"
};
JComboBox < String > difficultyDropdown = new JComboBox < String > (difficulties);
difficultyDropdown.setBounds(910, 335, 120, 25);
difficultyDropdown.setFont(new Font("Verdana", Font.PLAIN, 20));
panel.add(difficultyDropdown);
JButton playButton = new JButton("Play");
playButton.setBounds(530, 480, 220, 25);
playButton.setFont(new Font("Verdana", Font.PLAIN, 20));
playButton.addActionListener(new Minesweeper());
panel.add(playButton);
}
@Override
public void actionPerformed(ActionEvent e) {
}
}
任何建議都會非常有幫助
我清理了你的代碼。 我添加了一個PlayListener
class 作為播放按鈕的ActionListener
。 對於任一下拉菜單,您都不需要ActionListener
。 您可以在需要時在PlayListener
class 中獲取選定的下拉值。
我添加了對SwingUtilities
invokeLater
方法的調用。 此方法可確保您的 Swing 組件在Event Dispatch Thread上創建和執行。
我重新安排了您的JFrame
方法調用。 我不知道您是否注意到,但您在創建組件之前使JFrame
可見。 在顯示JFrame
之前,您必須創建所有組件。
不鼓勵使用 null 布局和絕對定位。 Swing GUI 必須在具有不同屏幕監視器像素大小的不同操作系統上運行。 你的 GUI 幾乎不適合我的顯示器。 我留下了你的絕對定位代碼,但在未來,你應該學會使用Swing 布局管理器。
我將AlsXYMouseLabelComponent
class 設為內聯 class。 您可以擁有任意數量的內聯類。 不過,一般來說,每個 class 都應該在一個單獨的文件中。 我制作了內聯類,以向您展示如何定義內聯類,並且還可以粘貼一個可運行的示例文件。
import java.awt.Color;
import java.awt.Cursor;
import java.awt.Font;
import java.awt.Graphics;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.awt.event.MouseEvent;
import java.awt.event.MouseMotionAdapter;
import javax.swing.JButton;
import javax.swing.JComboBox;
import javax.swing.JComponent;
import javax.swing.JFrame;
import javax.swing.JLabel;
import javax.swing.JLayeredPane;
import javax.swing.JPanel;
import javax.swing.SwingUtilities;
public class Minesweeper implements Runnable {
public static void main(String[] args) {
SwingUtilities.invokeLater(new Minesweeper());
}
private JComboBox<String> gameModeDropdown;
private JComboBox<String> difficultyDropdown;
@Override
public void run() {
JPanel panel = new JPanel();
JFrame frame = new JFrame("Minesweeper");
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.setCursor(new Cursor(Cursor.CROSSHAIR_CURSOR));
panel.setLayout(null);
AlsXYMouseLabelComponent alsXYMouseLabel =
new AlsXYMouseLabelComponent();
/**
* Add the component to the DRAG_LAYER of the layered pane (JLayeredPane)
*/
JLayeredPane layeredPane = frame.getRootPane().getLayeredPane();
layeredPane.add(alsXYMouseLabel, JLayeredPane.DRAG_LAYER);
alsXYMouseLabel.setBounds(0, 0, frame.getWidth(), frame.getHeight());
/**
* Add a mouse motion listener, and update the crosshair mouse cursor with the
* xy coordinates as the user moves the mouse
*/
frame.addMouseMotionListener(new MouseMotionAdapter() {
/**
* Detects when the mouse moved and what the mouse's coordinates are.
*
* @param event the event that happens when the mouse is moving.
*/
@Override
public void mouseMoved(MouseEvent event) {
alsXYMouseLabel.x = event.getX();
alsXYMouseLabel.y = event.getY();
alsXYMouseLabel.repaint();
}
});
JLabel title = new JLabel("Minesweeper");
title.setBounds(500, 100, 550, 60);
title.setFont(new Font("Verdana", Font.PLAIN, 50));
panel.add(title);
JLabel gameModePrompt = new JLabel("Game Mode: ");
gameModePrompt.setBounds(280, 335, 300, 25);
gameModePrompt.setFont(new Font("Verdana", Font.PLAIN, 20));
panel.add(gameModePrompt);
String[] gameModes = { "Normal" };
gameModeDropdown = new JComboBox<String>(gameModes);
gameModeDropdown.setBounds(415, 335, 290, 25);
gameModeDropdown.setFont(new Font("Verdana", Font.PLAIN, 20));
panel.add(gameModeDropdown);
JLabel difficultyPrompt = new JLabel("Difficulty: ");
difficultyPrompt.setBounds(800, 335, 240, 25);
difficultyPrompt.setFont(new Font("Verdana", Font.PLAIN, 20));
panel.add(difficultyPrompt);
String[] difficulties = { "Easy", "Medium", "Hard" };
difficultyDropdown = new JComboBox<String>(difficulties);
difficultyDropdown.setSelectedIndex(1);
difficultyDropdown.setBounds(910, 335, 120, 25);
difficultyDropdown.setFont(new Font("Verdana", Font.PLAIN, 20));
panel.add(difficultyDropdown);
JButton playButton = new JButton("Play");
playButton.setBounds(530, 480, 220, 25);
playButton.setFont(new Font("Verdana", Font.PLAIN, 20));
playButton.addActionListener(new PlayListener());
panel.add(playButton);
frame.add(panel);
frame.setSize(1365, 767);
frame.setVisible(true);
}
public class PlayListener implements ActionListener {
@Override
public void actionPerformed(ActionEvent event) {
// TODO Auto-generated method stub
}
}
/**
* A class that shows the xy coordinates near the mouse cursor.
*/
public class AlsXYMouseLabelComponent extends JComponent {
private static final long serialVersionUID = 1L;
public int x;
public int y;
/**
* Uses the xy coordinates to update the mouse cursor label.
*/
@Override
public void paintComponent(Graphics g) {
super.paintComponent(g);
String coordinates = x + ", " + y; // Get the cordinates of the mouse
g.setColor(Color.red);
g.drawString(coordinates, x, y); // Display the coordinates of the mouse
}
}
}
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.