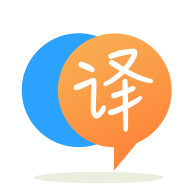
[英]How do I remove the border from multiple JButtons attached to one ActionListener (Created with for loop)?
[英]How do I add ActionListener to multiple buttons created in a for loop?
這是一個困難的問題,但是如何將ActionListener
添加到我在 for 循環中創建的多個按鈕中?
到目前為止,這是我的代碼:
import java.awt.*;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import javax.swing.*;
public class Loop extends JFrame {
public Loop() {
this.setSize(700, 300);
this.setLocation(400, 300);
this.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
JPanel jpanel = new JPanel();
jpanel.setBackground(Color.CYAN);
jpanel.setLayout(new GridLayout(0,6));
JButton[] jButton = new JButton[6];
String string[] = {"One","Two","Three","Four","Five","Six"};
this.add(jpanel);
int j=0;
for(int i =0; i<jButton.length;i++) {
while(j < 6) {
jButton[i]= new JButton();
jButton[i].setPreferredSize(new Dimension(50, 50));
jButton[i].setText(string[j++]);
jpanel.add(jButton[i]);
jButton[i].addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
System.out.println("Works");
}
});
}
}
}
public static void main(String[] args) {
Loop myLoop = new Loop();
myLoop.setVisible(true);
}
}
(從代碼看來,在for
循環中添加ActionListener
會給所有按鈕提供相同的操作。問題是我希望每個按鈕的操作都不同)。
任何回應將不勝感激。
您可以創建ActionListener
的列表或數組並將其傳遞給構造函數,例如
import java.awt.*;
import java.awt.event.ActionListener;
import java.util.List;
import javax.swing.*;
public class Loop extends JFrame {
public Loop(java.util.List<ActionListener> listeners) {
this.setSize(700, 300);
this.setLocation(400, 300);
this.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
JPanel jpanel = new JPanel();
jpanel.setBackground(Color.CYAN);
jpanel.setLayout(new GridLayout(0, 6));
JButton[] jButton = new JButton[6];
String[] string = {"One", "Two", "Three", "Four", "Five", "Six"};
this.add(jpanel);
int j = 0;
for (int i = 0; i < jButton.length; i++) {
while (j < 6) {
jButton[i] = new JButton();
jButton[i].setPreferredSize(new Dimension(50, 50));
jButton[i].setText(string[j]);
jpanel.add(jButton[i]);
jButton[i].addActionListener(listeners.get(j));
j++;
}
}
}
public static void main(String[] args) {
java.util.List<ActionListener> listeners = List.of(
e -> System.out.println("actionListener 1"),
e -> System.out.println("actionListener 2"),
e -> System.out.println("actionListener 3"),
e -> System.out.println("actionListener 4"),
e -> System.out.println("actionListener 5"),
e -> System.out.println("actionListener 6")
);
Loop myLoop = new Loop(listeners);
myLoop.setVisible(true);
}
}
從代碼中可以看出,在 for 循環中添加 ActionListener 會給所有按鈕提供相同的操作。
你是對的。 您正在創建一個實現ActionListener
接口的匿名 class 。 但是,每個JButton
都分配有該 class 的單獨實例。
將相同的單個實例分配給不同的JButton
沒有任何問題。 在actionPerformed
方法的代碼中,您可以通過檢查ActionEvent
參數來識別激活了哪個JButton
。
ActionEvent
包含一個動作命令字符串以及source ,在您的情況下,它是被激活的實際JButton
。
就個人而言,我會讓您的Loop
class 實現ActionListener
並在actionPerformed
方法中確定從action command激活了哪個JButton
。 下面的代碼演示。
import java.awt.*;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import javax.swing.*;
public class Loop extends JFrame implements ActionListener {
public Loop() {
this.setSize(700, 300);
this.setLocation(400, 300);
this.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
JPanel jpanel = new JPanel();
jpanel.setBackground(Color.CYAN);
jpanel.setLayout(new GridLayout(0, 6));
JButton[] jButton = new JButton[6];
String string[] = {"One", "Two", "Three", "Four", "Five", "Six"};
this.add(jpanel);
for (int i = 0; i < jButton.length; i++) {
jButton[i] = new JButton(string[i]);
jButton[i].setPreferredSize(new Dimension(50, 50));
jpanel.add(jButton[i]);
jButton[i].addActionListener(this);
}
}
public void actionPerformed(ActionEvent event) {
String actionCommand = event.getActionCommand();
switch (actionCommand) {
case "One":
break;
case "Two":
break;
case "Three":
break;
case "Four":
break;
case "Five":
break;
case "Six":
break;
default:
}
}
public static void main(String[] args) {
Loop myLoop = new Loop();
myLoop.setVisible(true);
}
}
默認情況下,操作命令被分配了JButton
的文本,但您可以通過setActionCommand方法分配不同的值。
你可以這樣做:
import java.awt.*;
import java.util.stream.Stream;
import javax.swing.*;
@SuppressWarnings("serial")
public class Loop extends JFrame {
public Loop() {
final JPanel jpanel = new JPanel();
/**/ jpanel.setBackground(Color.CYAN);
/**/ jpanel.setLayout(new GridLayout(0, 6));
this.add (jpanel);
Stream.of("One", "Two", "Three", "Four", "Five", "Six")
.forEach(text -> {
final JButton button = new JButton(text);
/**/ button.setPreferredSize(new Dimension(50, 50));
/**/ button.addActionListener((e) -> {System.out.println("Button " + text + " pressed");});
jpanel.add (button);
});
this.setPreferredSize(new Dimension(700, 300));
this.setLocation(400, 300);
this.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
this.pack();
this.setResizable(false);
this.setVisible(true);
}
public static void main(final String[] args) {
SwingUtilities.invokeLater(() -> new Loop());
}
}
您需要某種方式來識別哪個 Button,但您可以使用 1 個共享偵聽器,如下所示。
請注意使用SwingUtilities.invokeLater(...)
在正確的線程上執行。
import java.awt.*;
import java.awt.event.ActionListener;
import java.util.stream.Stream;
import javax.swing.*;
@SuppressWarnings("serial")
public class Loop extends JFrame {
public Loop() {
final JPanel jpanel = new JPanel();
/**/ jpanel.setBackground(Color.CYAN);
/**/ jpanel.setLayout(new GridLayout(0, 6));
this.add (jpanel);
final ActionListener oneSharedListener = (e) -> System.out.println("Button " + e.getActionCommand() + " pressed");
Stream.of("One", "Two", "Three", "Four", "Five", "Six")
.forEach(text -> {
final JButton button = new JButton(text);
/**/ button.setPreferredSize(new Dimension(50, 50));
/**/ button.addActionListener(oneSharedListener);
/**/ button.setActionCommand(text);
jpanel.add (button);
});
this.setPreferredSize(new Dimension(700, 300));
this.setLocation(400, 300);
this.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
this.pack();
this.setResizable(false);
this.setVisible(true);
}
public static void main(final String[] args) {
SwingUtilities.invokeLater(() -> new Loop());
}
}
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.