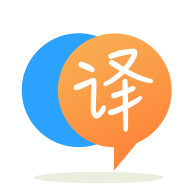
[英]Angular2,How to broadcast event from parent component to child directive?
[英]Angular 9: How to get data from parent component in child directive
我有父組件,里面有子指令。 需要從指令中的組件獲取輸入數據。
<my-parent [data]="'Hello from parent'">
<input myChild>
</my-parent>
父組件
@Component({
selector: 'my-parent',
template: `
<ng-content select="[myChild]"></ng-content>
`
})
export class ParentComponent {
@Input() data: string;
}
兒童指令
@Directive({
selector: '[myChild]'
})
export class ChildDirective implements OnInit {
value: string;
ngOnInit() {
// needs to get data from parent component here
}
}
我可以將父組件注入指令,但我不想這樣做,因為它會增加循環依賴的風險。
我可以在 ParentComponent 中使用@ContentChild
並通過公共屬性在AfterContentInit
中傳遞數據,但我不確定此屬性何時可以在 ChildDirective 中使用,我應該在這種情況下使用AfterContentChecked
嗎?
父組件
@ContentChild(ChildDirective, {static: true}) child: ChildDirective;
ngAfterContentInit() {
this.child.value = this.data;
}
兒童指令
value: string;
ngAfterContentChecked() {
// will be available data from parent here?
}
也許我應該使用服務在組件和指令之間共享數據?
https://stackblitz.com/edit/angular-pass-data-to-child-directive
是否有特殊原因需要使用 ng-content 而不是 ng-template?
我建議您使用 ng-templates 的方法如下
父組件
@Component({
selector: 'my-parent',
template: `
<ng-template
[ngTemplateOutlet]="child"
[ngTemplateOutletContext]="{ $implicit: data }">
</ng-template>
`
})
export class ParentComponent implements AfterContentInit {
@Input() data: string;
@ContentChild(TemplateRef, {static: true}) child: TemplateRef<any>;
}
兒童指令
@Directive({
selector: '[myChild]'
})
export class ChildDirective {
@Input()
value: string;
}
用法
<my-parent [data]="'Hello from parent'">
<ng-template let-dataThroughParent>
<input myChild [value]="dataThroughParent" class="form-control">
</ng-template>
</my-parent>
重要的
在這種特定情況下(示例),實際上沒有必要將數據綁定到您的父級,然后通過模板變量獲取它,然后再次將其綁定到您的“myChild”指令,因為如果您的用例與此類似,我會將數據直接傳遞給“myChild”指令,如下所示:
<my-parent [data]="'Hello from parent'">
<ng-template>
<input myChild [value]="'Hello from parent'" class="form-control">
</ng-template>
</my-parent>
請注意,它也適用於您的初始方法:
<my-parent [data]="'Hello from parent'">
<input myChild [value]="'Hello from parent'">
</my-parent>
但我假設您確實需要從使用父級的 scope 中無法訪問的父級傳遞數據。 這是一個工作示例
https://stackblitz.com/edit/angular-pass-data-to-child-directive-ebcbg6
您可以使用@Input()
綁定將數據傳遞給指令。
app.component.html
<my-parent [data]="'Hello from parent'"></my-parent>
我的父級組件.ts
@Component({
selector: 'my-parent',
templateUrl: './my-parent.component.html',
styleUrls: ['./my-parent.component.scss'],
})
export class MyParentComponent implements OnInit {
@Input() data: string;
}
我的父組件.html
<input [myChild]="data"/>
my-child.directive.ts
@Directive({
selector: '[myChild]'
})
export class ChildDirective implements OnInit {
value: string;
@Input('myChild') data: string;
ngOnInit() {
console.log(this.data) // data from parent
}
}
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.