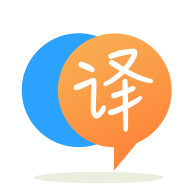
[英]3 textboxes, 1 button, on button click put text in the textbox where the cursor is
[英]How can I insert text via button click at the cursor in the most recent textbox used?
我有一個帶有多個文本框和一組按鈕的表單。 使用下面的 Javascript,我可以單擊一個按鈕並將文本插入其中一個命名框。
單擊按鈕時是否可以將文本插入最新/活動的文本框?
目前我有這個,但它使用的是文本框的特定 ID,而不是最近使用/活動的 ID;
$( 'input[type=button]' ).on('click', function(){
var cursorPos = $('#my_text_box').prop('selectionStart');
var v = $('#my_text_box').val();
var textBefore = v.substring(0, cursorPos );
var textAfter = v.substring( cursorPos, v.length );
$('#my_text_box').val( textBefore+ $(this).val() +textAfter );
});
JSFiddle 上的示例更清晰;
http://jsfiddle.net/bd1xf0sj/1/
這個想法取決於單擊按鈕時您所在的文本框,而不是使用某個文本框的 ID。 這個想法是可以單擊按鈕並且可以使用任何文本框。
您可以創建一個變量來存儲最新的文本框,並在文本框像這樣聚焦時更新它:
let currentInput = $('#text1');
$(document).on('focus', 'textarea', function() {
currentInput = $(this);
})
然后使用此變量來更新文本,就像您對#my_text_box
所做的那樣:
$( 'input[type=button]' ).on('click', function(){
let cursorPos = currentInput.prop('selectionStart');
let v = currentInput.val();
let textBefore = v.substring(0, cursorPos );
let textAfter = v.substring( cursorPos, v.length );
currentInput.val( textBefore + $(this).val() + textAfter );
});
更新:插入文本后保留 cursor position 的一些修改:
let currentInput = document.getElementById('text1'); $(document).on('focus', 'textarea', function() { currentInput = this; }) $( 'input[type=button]' ).on('click', function(){ let cursorPos = currentInput.selectionStart; let v = currentInput.value; let textBefore = v.substring(0, cursorPos ); let textAfter = v.substring( cursorPos, v.length ); currentInput.value = textBefore + this.value + textAfter; cursorPos += this.value.length; currentInput.focus(); currentInput.setSelectionRange(cursorPos, cursorPos); });
<script src="https://cdnjs.cloudflare.com/ajax/libs/jquery/3.3.1/jquery.min.js"></script> <form> <textarea id="text1" cols="40" rows="3"></textarea> <textarea id="text2" cols="40" rows="3"></textarea> <textarea id="text3" cols="40" rows="3"></textarea> <textarea id="text4" cols="40" rows="3"></textarea> <br> <input type="button" value="one" /> <input type="button" value="two" /> <input type="button" value="three" /> </form>
您可以使用在輸入元素 HTML 中設置為 boolean 值true
或false
的data-attribute
來跟蹤輸入焦點的當前 state。
首先將第一個data-current
屬性定義為true ,每隔一個屬性定義為false 。 這樣,默認設置為true 。 然后我們運行一個事件來檢查對textarea元素的關注。 如果textarea被關注,我們刪除選擇器data-current
值的所有實例並將它們全部設置為false ,然后我們將當前事件目標data-current
值設置為true 。
然后在按鈕的單擊事件中,我們檢查迭代它們的textarea元素並運行檢查它們的data-current
值的條件,我們找到設置為true的那個,然后將其值分配給按鈕值。
$('textarea').focus(e => { let $this = e.target; $('textarea').each((i, el) => { if(el.dataset.current === 'true'){ el.dataset.current = false; } $this.dataset.current = true; }) console.log(`Selected TextArea's id: ${$this.id}`) }) $('input[type=button]').click(function(){ const $this = $(this); const $ta = $('textarea'); $ta.each((i, el) => { // do not retain previously set values when a new button // is selected on a different focused textarea el.dataset.current === 'true'? el.value = $this.val(): el.value = ''; /* // retain previously set values in TA's on button click // when new textarea is focused el.dataset.current === 'true'? el.value = $this.val(): null; */ }) })
<script src="https://cdnjs.cloudflare.com/ajax/libs/jquery/3.3.1/jquery.min.js"></script> <form> <textarea id="text1" data-current="true" cols="40" rows="3"></textarea> <textarea id="text2" data-current="false" cols="40" rows="3"></textarea> <textarea id="text3" data-current="false" cols="40" rows="3"></textarea> <br> <input type="button" value="one" /> <input type="button" data-current="false" value="two" /> <input type="button" data-current="false" value="three" /> </form>
這實際上是一個簡單而有趣的練習來解決,有很多方法可以解決這個特定問題,盡管我會建議我的。
這是解決的jsFiddle示例的鏈接。
解釋:
第 1 步:在所有textarea
上添加focus
事件偵聽器。
第 2 步:在textarea
集中,從現有last-focused.textarea
元素中刪除 class last-focused
元素。
第 3 步:將last-focused
class 添加到當前聚焦的textarea
元素。
第 4 步:在button
上添加click
監聽器
第 5 步:在單擊button
時,select last-focused.textarea
focused.textarea 元素,並將其內容替換為按鈕的值。
注意:我們可以為 class 使用任何名稱,只需將名稱命名為last-focused
,因為它與當前上下文相關。
HTML:
<form>
<textarea id="text1" cols="40" rows="3"></textarea>
<textarea id="text2" cols="40" rows="3"></textarea>
<br>
<input type="button" value="one" />
<input type="button" value="two" />
<input type="button" value="three" />
</form>
JS + JQuery:
// Step 1
$('textarea').on('focus', function() {
// Step 2
$('textarea.last-focused').removeClass('last-focused');
// Step 3
$(this).addClass('last-focused');
});
// Step 4
$('input[type=button]').on('click', function() {
// Step 5
$('textarea.last-focused').html($(this).val());
});
您可以使用 textarea 元素的焦點事件和 append 單擊按鈕時最后一個活動元素的值來跟蹤最后一個焦點輸入元素:
var mostrecent=$('textarea')[0];
$('textarea').each(function(i,item){
$(item).focus(function(){
mostrecent=this;
});
});
var mostrecent=$('textarea')[0]; $('textarea').each(function(i,item){ $(item).focus(function(){ mostrecent=this; }); }); $( 'input[type=button]' ).on('click', function(){ var cursorPos = $('#text1').prop('selectionStart'); var v = $('#text1').val(); var textBefore = v.substring(0, cursorPos ); var textAfter = v.substring( cursorPos, v.length ); $(mostrecent).val( textBefore+ $(this).val() +textAfter ); });
<script src="https://cdnjs.cloudflare.com/ajax/libs/jquery/3.3.1/jquery.min.js"></script> <form> <textarea id="text1" cols="40" rows="3"></textarea> <textarea id="text2" cols="40" rows="3"></textarea> <br> <input type="button" value="one" /> <input type="button" value="two" /> <input type="button" value="three" /> </form>
掛鈎表單事件以將最近的輸入標記為“最近的”(使用某個類)並取消標記所有其他輸入。 單擊修改“最近”輸入。
您很可能需要onfocus
,但可能會根據您的特定用例進行onchange
。
當前選擇的字段是 document.activeElement。
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.