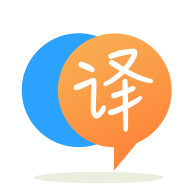
[英]How to call an Object's Method from another class without creating a sub-class and/or inheriting class?
[英]How to implement an abstarct class's method in sub-class by getting variables of it's parents class using a constructor in c++
首先,有一個名為 Shape 的父類,它有兩個構造函數,一個帶有一個參數,另一個帶有兩個參數。有兩個類繼承了類“Shape”的屬性。 它們是 Rectangle 和 Circle 。
我用java試過了,我得到了我想要的。
這是java實現..
package javaapplication6;
import java.io.*;
abstract class Shape{
protected int radius,length,width;
public Shape(int n){
radius=n;
}
public Shape(int x,int y){
length=x;
width=y;
}
abstract public void getArea();
}
class Rectangle extends Shape{
public Rectangle(int x,int y){
super(x,y);
}
public void getData(){
System.out.println(length+" "+width);
}
public void getArea(){
System.out.println("Area of Reactangle is : "+width*length);
}
}
class Circle extends Shape{
public Circle(int x){
super(x);
}
public void getData(){
System.out.println(radius);
}
public void getArea(){
System.out.println("Area of Reactangle is : "+2*radius*3.14);
}
}
public class JavaApplication6 {
public static void main(String[] args) {
Rectangle r=new Rectangle(3,4);
r.getData();
r.getArea();
System.out.println();
Circle c=new Circle(3);
c.getData();
c.getArea();
}
}
我想要在 C++ 中實現的確切內容..
我試過如下...
#include<bits/stdc++.h>
using namespace std;
class Shape{
public:
int r,x,y;
Shape(int rad){
r=rad;
}
Shape(int height,int width){
x=height;
y=width;
}
void getClass(){
cout<<"Ur in class shape"<<endl;
}
virtual void getArea();
};
class Rectangle : public Shape{
public:
Rectangle(int x,int y):Shape(x,y){}
void getArea(){
cout<< "Area of rectangle : "<<x * y<<endl;
}
void getClass(){
cout<<"Ur in class Rectangle"<<endl;
}
};
class Circle : public Shape{
public:
Circle(int r):Shape(r){}
vooid getArea(){
cout<< "Area of Circle : "<<2* 3.14 * r<<endl;
}
void getClass(){
cout<<"Ur in class Circle"<<endl;
}
};
int main(){
Circle c(5);
c.getClass();
c.getArea();
Rectangle r(3,4);
r.getClass();
r.getArea();
}
但我收到一個錯誤..
abstract.cpp:(.rdata$.refptr._ZTV5Shape[.refptr._ZTV5Shape]+0x0): undefined reference to `vtable for Shape'
您收到錯誤是因為Shape::getArea
沒有定義,也沒有聲明為純虛擬:
virtual void getArea();
要使其純虛擬,您需要:
virtual void getArea() = 0;
您還應該提供一個虛擬析構函數。 何時需要,何時不需要超出了本問題的范圍。 最簡單的就是提供它:
virtual ~Shape(){};
“未定義對 vtable 的引用”錯誤來自未為所有未定義為純虛擬的virtual
函數提供定義。
在您的示例中,您聲明Shape::getArea
,但您沒有將其聲明為純virtual
或給它一個定義,這是您問題的根源:
class Shape{
...
virtual void getArea(); // declared, but not pure virtual or defined
};
要使其純虛擬,最后需要= 0
。
class Shape{
...
virtual void getArea() = 0;
};
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.