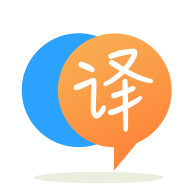
[英]Find the most common sentences/phrases among millions of documents using Python
[英]Finding most similar sentences among all in python
建議/參考鏈接/代碼表示贊賞。
我有一個超過 1500 行的數據。 每一行都有一個句子。 我試圖找出在所有句子中找到最相似句子的最佳方法。
我試過的
我嘗試過 K-mean 算法,它將相似的句子分組在一個集群中。 但是我發現了一個缺點,我必須通過K來創建一個集群。 很難猜測K 。 我嘗試了 elbo 方法來猜測集群,但將所有組合在一起是不夠的。 在這種方法中,我將所有數據分組。 我正在尋找與 0.90% 以上的數據類似的數據,應返回 ID。
我嘗試了余弦相似度,其中我使用TfidfVectorizer
創建矩陣,然后傳入余弦相似度。 即使這種方法也不能正常工作。
我在尋找什么
我想要一種方法,我可以在其中傳遞閾值示例 0.90 的所有行中的數據,這些數據應該作為結果返回。
Data Sample
ID | DESCRIPTION
-----------------------------
10 | Cancel ASN WMS Cancel ASN
11 | MAXPREDO Validation is corect
12 | Move to QC
13 | Cancel ASN WMS Cancel ASN
14 | MAXPREDO Validation is right
15 | Verify files are sent every hours for this interface from Optima
16 | MAXPREDO Validation are correct
17 | Move to QC
18 | Verify files are not sent
預期結果
上面的數據相似度高達 0.90% 應該得到帶有ID的結果
ID | DESCRIPTION
-----------------------------
10 | Cancel ASN WMS Cancel ASN
13 | Cancel ASN WMS Cancel ASN
11 | MAXPREDO Validation is corect # even spelling is not correct
14 | MAXPREDO Validation is right
16 | MAXPREDO Validation are correct
12 | Move to QC
17 | Move to QC
為什么它對余弦相似度和 TFIDF 向量化器不起作用?
我試過了,它適用於以下代碼:
import pandas as pd
import numpy as np
from sklearn.feature_extraction.text import TfidfVectorizer
from sklearn.metrics.pairwise import cosine_similarity
df = pd.DataFrame(columns=["ID","DESCRIPTION"], data=np.matrix([[10,"Cancel ASN WMS Cancel ASN"],
[11,"MAXPREDO Validation is corect"],
[12,"Move to QC"],
[13,"Cancel ASN WMS Cancel ASN"],
[14,"MAXPREDO Validation is right"],
[15,"Verify files are sent every hours for this interface from Optima"],
[16,"MAXPREDO Validation are correct"],
[17,"Move to QC"],
[18,"Verify files are not sent"]
]))
corpus = list(df["DESCRIPTION"].values)
vectorizer = TfidfVectorizer()
X = vectorizer.fit_transform(corpus)
threshold = 0.4
for x in range(0,X.shape[0]):
for y in range(x,X.shape[0]):
if(x!=y):
if(cosine_similarity(X[x],X[y])>threshold):
print(df["ID"][x],":",corpus[x])
print(df["ID"][y],":",corpus[y])
print("Cosine similarity:",cosine_similarity(X[x],X[y]))
print()
閾值也可以調整,但閾值為 0.9 時不會產生您想要的結果。
閾值為 0.4 的輸出為:
10 : Cancel ASN WMS Cancel ASN
13 : Cancel ASN WMS Cancel ASN
Cosine similarity: [[1.]]
11 : MAXPREDO Validation is corect
14 : MAXPREDO Validation is right
Cosine similarity: [[0.64183024]]
12 : Move to QC
17 : Move to QC
Cosine similarity: [[1.]]
15 : Verify files are sent every hours for this interface from Optima
18 : Verify files are not sent
Cosine similarity: [[0.44897995]]
閾值為 0.39 時,所有預期的句子都是輸出中的特征,但也可以找到帶有索引 [15,18] 的附加對:
10 : Cancel ASN WMS Cancel ASN
13 : Cancel ASN WMS Cancel ASN
Cosine similarity: [[1.]]
11 : MAXPREDO Validation is corect
14 : MAXPREDO Validation is right
Cosine similarity: [[0.64183024]]
11 : MAXPREDO Validation is corect
16 : MAXPREDO Validation are correct
Cosine similarity: [[0.39895808]]
12 : Move to QC
17 : Move to QC
Cosine similarity: [[1.]]
14 : MAXPREDO Validation is right
16 : MAXPREDO Validation are correct
Cosine similarity: [[0.39895808]]
15 : Verify files are sent every hours for this interface from Optima
18 : Verify files are not sent
Cosine similarity: [[0.44897995]]
可以使用這個 Python 3 庫來計算句子相似度: https : //github.com/UKPLab/sentence-transformers
來自https://www.sbert.net/docs/usage/semantic_textual_similarity.html 的代碼示例:
from sentence_transformers import SentenceTransformer, util
model = SentenceTransformer('paraphrase-MiniLM-L12-v2')
# Two lists of sentences
sentences1 = ['The cat sits outside',
'A man is playing guitar',
'The new movie is awesome']
sentences2 = ['The dog plays in the garden',
'A woman watches TV',
'The new movie is so great']
#Compute embedding for both lists
embeddings1 = model.encode(sentences1, convert_to_tensor=True)
embeddings2 = model.encode(sentences2, convert_to_tensor=True)
#Compute cosine-similarits
cosine_scores = util.pytorch_cos_sim(embeddings1, embeddings2)
#Output the pairs with their score
for i in range(len(sentences1)):
print("{} \t\t {} \t\t Score: {:.4f}".format(sentences1[i], sentences2[i], cosine_scores[i][i]))
該庫包含最先進的句子嵌入模型。
請參閱https://stackoverflow.com/a/68728666/395857以執行句子聚類。
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.