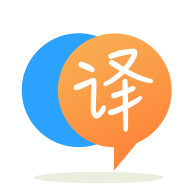
[英]What's tight time complexity of this algorithm for Binary Tree Zigzag Level Order Traversal?
[英]What is the time complexity of this segment tree algorithm?
class SegTreeNode {
public:
int start;
int end;
int min;
SegTreeNode *left;
SegTreeNode *right;
SegTreeNode(int start, int end) {
this->start = start;
this->end = end;
left = right = NULL;
}
};
class Solution {
public:
int largestRectangleArea(vector<int>& heights) {
if (heights.size() == 0) return 0;
// first build a segment tree
SegTreeNode *root = buildSegmentTree(heights, 0, heights.size() - 1);
// next calculate the maximum area recursively
return calculateMax(heights, root, 0, heights.size() - 1);
}
int calculateMax(vector<int>& heights, SegTreeNode* root, int start, int end) {
if (start > end) {
return -1;
}
if (start == end) {
return heights[start];
}
int minIndex = query(root, heights, start, end);
int leftMax = calculateMax(heights, root, start, minIndex - 1);
int rightMax = calculateMax(heights, root, minIndex + 1, end);
int minMax = heights[minIndex] * (end - start + 1);
return max( max(leftMax, rightMax), minMax );
}
SegTreeNode *buildSegmentTree(vector<int>& heights, int start, int end) {
if (start > end) return NULL;
SegTreeNode *root = new SegTreeNode(start, end);
if (start == end) {
root->min = start;
return root;
} else {
int middle = (start + end) / 2;
root->left = buildSegmentTree(heights, start, middle);
root->right = buildSegmentTree(heights, middle + 1, end);
root->min = heights[root->left->min] < heights[root->right->min] ? root->left->min : root->right->min;
return root;
}
}
int query(SegTreeNode *root, vector<int>& heights, int start, int end) {
if (root == NULL || end < root->start || start > root->end) return -1;
if (start <= root->start && end >= root->end) {
return root->min;
}
int leftMin = query(root->left, heights, start, end);
int rightMin = query(root->right, heights, start, end);
if (leftMin == -1) return rightMin;
if (rightMin == -1) return leftMin;
return heights[leftMin] < heights[rightMin] ? leftMin : rightMin;
}
};
這是這個leet代碼問題的解決方案https://leetcode.com/problems/largest-rectangle-in-histogram/
我相信以下代碼應該具有 logN * logN 的平均時間復雜度和 NlogN 的最壞情況時間復雜度
我的推理是,calculateMax 的遞歸平均將采用 logN,並且只會在最壞的情況下惡化到 N,其中數組中矩形的高度按升序或降序排序。
我只想知道我的邏輯是否正確,並希望得到某種確認。
謝謝 :)
你的推理是對的。 但是為什么您使用 Segment 樹使代碼如此復雜。 也可以使用堆棧來完成。
參考這個: 最大化矩形區域下直方圖
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.