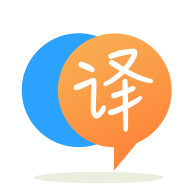
[英]Azure Mobile App with ASP.Net backend posting data to a SQL Server table with a foreign key
[英]SQL query to insert data into a table containing foreign key in asp.net
這是一個包含了被點擊時應該從文本框的數據添加到賬單表在這里添加按鈕的代碼crid
, crname
, cid
, cname
和truckid
外鍵。
單擊添加按鈕時,數據未添加到表中,並且沒有顯示錯誤,因此我不確定是什么原因造成的。
using System;
using System.Configuration;
using System.Data;
using System.Data.SqlClient;
using System.Web.UI;
namespace project
{
public partial class bill : System.Web.UI.Page
{
string strcon = ConfigurationManager.ConnectionStrings["con"].ConnectionString;
protected void Page_Load(object sender, EventArgs e)
{
GridView2.DataBind();
}
//add
protected void Button1_Click(object sender, EventArgs e)
{
if (BillCheck())
{
ScriptManager.RegisterClientScriptBlock(this, this.GetType(), "alertMessage",
"alert('This bill already exists. Please generate a new bill.')", true);
}
else
{
addNewBill();
}
}
//user defined function
void addNewBill()
{
if (TextBox1.Text.Trim().Equals(""))
{
ScriptManager.RegisterClientScriptBlock(this, this.GetType(), "alertMessage",
"alert(GR no cannot be blank')", true);
}
else
{
try
{
SqlConnection con = new SqlConnection(strcon);
if (con.State == ConnectionState.Closed)
{
con.Open();
}
SqlCommand cmd = new SqlCommand(
"insert into bill (GRNo,Date,from,to,crid,crname,cid,cname,package," +
"description,HSNcode,privatemark,invoiceno,value,truckid,paymentmode,actual,charged,amount)" +
" (@GRNo,@Date,@from,@to,(select crid from Consignor where crid='" + TextBox4.Text.Trim() +
"';)," +
"(select crname from Consignor where crname='" + TextBox5.Text.Trim() + "';)," +
"(select cid from Consignee where cid='" + TextBox8.Text.Trim() + "';)," +
"(select cname from Consignee where cname='" + TextBox9.Text.Trim() +
"';),@package,@description,@HSNcode,@privatemark," +
"@invoiceno,@value,@paymentmode,@actual,@charged,@amount);", con);
cmd.Parameters.AddWithValue("@GRNo", TextBox1.Text.Trim());
cmd.Parameters.AddWithValue("@Date", TextBox2.Text.Trim());
cmd.Parameters.AddWithValue("@from", TextBox7.Text.Trim());
cmd.Parameters.AddWithValue("@to", TextBox3.Text.Trim());
cmd.Parameters.AddWithValue("@crid", TextBox4.Text.Trim());
cmd.Parameters.AddWithValue("@crname", TextBox5.Text.Trim());
cmd.Parameters.AddWithValue("@cid", TextBox8.Text.Trim());
cmd.Parameters.AddWithValue("@cname", TextBox9.Text.Trim());
cmd.Parameters.AddWithValue("@package", TextBox6.Text.Trim());
cmd.Parameters.AddWithValue("@description", TextBox10.Text.Trim());
cmd.Parameters.AddWithValue("@HSNcode", TextBox11.Text.Trim());
cmd.Parameters.AddWithValue("@privatemark", TextBox12.Text.Trim());
cmd.Parameters.AddWithValue("@invoiceno", TextBox13.Text.Trim());
cmd.Parameters.AddWithValue("@value", TextBox14.Text.Trim());
cmd.Parameters.AddWithValue("@truckid", DropDownList1.SelectedItem.Value);
cmd.Parameters.AddWithValue("@paymentmode", DropDownList2.SelectedItem.Value);
cmd.Parameters.AddWithValue("@actual", TextBox21.Text.Trim());
cmd.Parameters.AddWithValue("@charged", TextBox22.Text.Trim());
cmd.Parameters.AddWithValue("@amount", TextBox23.Text.Trim());
cmd.ExecuteNonQuery();
con.Close();
ScriptManager.RegisterClientScriptBlock(this, this.GetType(), "alertMessage",
"alert('New Bill added Successfully!')", true);
clearForm();
GridView2.DataBind();
}
catch (Exception ex)
{
ScriptManager.RegisterClientScriptBlock(this, this.GetType(), "alertMessage",
"alert('" + ex.Message + "')", true);
}
}
}
public bool BillCheck()
{
try
{
SqlConnection con = new SqlConnection(strcon);
if (con.State == ConnectionState.Closed)
{
con.Open();
}
SqlCommand cmd = new SqlCommand("Select * from bill where GRNo='" + TextBox1.Text.Trim() + "';", con);
SqlDataAdapter da = new SqlDataAdapter(cmd);
DataTable dt = new DataTable();
da.Fill(dt);
if (dt.Rows.Count >= 1)
{
return true;
}
else
{
return false;
}
}
catch (Exception ex)
{
ScriptManager.RegisterClientScriptBlock(this, this.GetType(), "alertMessage",
"alert('" + ex.Message + "')", true);
return false;
}
}
void clearForm()
{
TextBox1.Text = "";
TextBox2.Text = "";
TextBox3.Text = "";
TextBox4.Text = "";
TextBox5.Text = "";
TextBox6.Text = "";
TextBox7.Text = "";
TextBox8.Text = "";
TextBox9.Text = "";
TextBox10.Text = "";
TextBox11.Text = "";
TextBox12.Text = "";
TextBox13.Text = "";
TextBox14.Text = "";
TextBox21.Text = "";
TextBox22.Text = "";
TextBox23.Text = "";
DropDownList1.SelectedValue = "";
DropDownList2.SelectedValue = "";
}
}
}
我試過用值編寫查詢,沒有它仍然無法正常工作。 我試過檢查我的數據庫以查看是否添加了行我試過從 select 語句中刪除分號,但它仍然不起作用。 這是我的帳單表列的圖像。
我認為你的 sql st 有問題,你不能像你一樣使用 param,試試這個
SqlCommand cmd = new SqlCommand("insert into bill (GRNo,Date,from,to,crid,crname,cid,cname,package," +"description,HSNcode,privatemark,invoiceno,value,truckid,paymentmode,actual,charged,amount)" + " (@GRNo,@Date,@from,@to,(select crid from Consignor where crid=@crid;)," +"(select crname from Consignor where crname=@crname;)," +"(select cid from Consignee where cid=@cid;)," +"(select cname from Consignee where cname=@cname;),@package,@description,@HSNcode,@privatemark," + "@invoiceno,@value,@paymentmode,@actual,@charged,@amount);", con);
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.