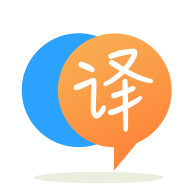
[英]How can I refresh navigation page from left menu master page if navigation page is already on top of the stack?
[英]How to refresh page from left menu
Xamarin 表單使用 MVVM .. 使用漢堡示例具有左側菜單。
//MainPage
[XamlCompilation(XamlCompilationOptions.Compile)]
public partial class MainPage : MasterDetailPage
{
int idOfNewPage;
Dictionary<int, NavigationPage> MenuPages = new Dictionary<int, NavigationPage>();
public MainPage()
{
InitializeComponent();
MasterBehavior = MasterBehavior.Popover;
MenuPages.Add((int)MenuItemType.Products, (NavigationPage)Detail);
}
public async Task NavigateFromMenu(int id)
{
if (!MenuPages.ContainsKey(id))
{
switch (id)
{
case (int)MenuItemType.Products:
MenuPages.Add(id, new NavigationPage(new ProductPage()));
break;
case (int)MenuItemType.Shopping:
MenuPages.Add(id, new NavigationPage(new ShoppingPage()));
break;
case (int)MenuItemType.Browse:
MenuPages.Add(id, new NavigationPage(new ItemsPage()));
break;
case (int)MenuItemType.About:
MenuPages.Add(id, new NavigationPage(new AboutPage()));
break;
}
idOfNewPage = id;
}
idOfNewPage = id;
var newPage = MenuPages[id];
if (newPage != null)
{
Detail = newPage;
if (Device.RuntimePlatform == Device.Android)
await Task.Delay(100);
IsPresented = false;
}
}
}
[XamlCompilation(XamlCompilationOptions.Compile)]
public partial class MenuPage : ContentPage
{
MainPage RootPage { get => Application.Current.MainPage as MainPage; }
List<HomeMenuItem> menuItems;
public MenuPage()
{
InitializeComponent();
menuItems = new List<HomeMenuItem>
{
new HomeMenuItem {Id = MenuItemType.Products, Title="Products", Icon = "back_nav.png", Name = "test" },
new HomeMenuItem {Id = MenuItemType.ShoppingCart, Title="Shopping Cart", Icon = "back_nav.png"},
new HomeMenuItem {Id = MenuItemType.Browse, Title="Browse", Icon = "back_nav.png"},
new HomeMenuItem {Id = MenuItemType.About, Title="About", Icon = "back_nav.png"}
};
ListViewMenu.ItemsSource = menuItems;
ListViewMenu.ItemSelected += async (sender, e) =>
{
if (e.SelectedItem == null)
return;
var id = (int)((HomeMenuItem)e.SelectedItem).Id;
var MenuBtnClicked = (string)((HomeMenuItem)e.SelectedItem).Title;
await RootPage.NavigateFromMenu(id);
ListViewMenu.SelectedItem = null;
};
}
}
所以這一切正常,我可以使用左側的菜單從頁面跳回和第四頁。 然而問題出在shoppingPage上。
產品列表視圖顯示在產品頁面上。(下)如果用戶更改產品的數量,則調用 WineQuantityChanged()。 更新屏幕上的數量並調用 - UpdateShoppingCartItems()。 它將產品添加到 DrinksToPurchaseList。 現在,當用戶單擊購物車圖標(作為工具欄項添加,而不是從菜單添加)時,代碼調用 ShoppingClicked(),它使用 DrinksToPurchase 列表填充 App.globalShoppingCartOC 並加載購物車頁面。
//ProductPage.cs
public ProductPage()
{
PopulateQuantityPicker();
InitializeComponent();
productPage_ViewModal = new ProductPageViewModel();
NoItemsInShoppingCart.Text = App.NoOfItemsInShoppingCartGlobalVar;
BindingContext = productPage_ViewModal;
}
private async void ShoppingClicked(object sender, EventArgs e)
{
//global list storing up to date products and quantites
App.globalShoppingCartOC = DrinksToPurchase;
await RootPage.NavigateFromMenu(1);
}
void WineQuantityChanged(object sender, EventArgs e)
{
var picker = (Picker)sender;
int newQuantity = picker.SelectedIndex;
string winePickerId = picker.Id.ToString();
int oldQuantity = -1;
int tempDiffOfValues = 0;
var product = (ProductModel)picker.BindingContext;
//code to update quantity
//update shopping cart items
UpdateShoppingCartItems(product, newQuantity);
NoItemsInShoppingCart.Text = quantityOfProducts.ToString();
App.NoOfItemsInShoppingCartGlobalVar = quantityOfProducts.ToString();
}
}
//update item in shopping cart with new quantity
void UpdateShoppingCartItems(ProductModel product, int quantity)
{
if (!DrinksToPurchaseList.Contains(product))
{
product.Quantity = quantity;
DrinksToPurchaseList.Add(product);
}
else
{
ProductModel result = DrinksToPurchaseList.Where(x => x.ProductId == product.ProductId).FirstOrDefault();
if (result != null)
{
//remove from list
if (quantity < 1)
{
DrinksToPurchaseList.Remove(result);
}
else // else update new quantity
{
result.Quantity = quantity;
}
}
}
}
//ProductPage.xaml
<ToolbarItem x:Name="NumberOfItemsInShoppingCart" Priority="0" Order="Primary" Activated="ShoppingClicked"/>
<StackLayout Grid.Row="2">
<Button Text="Wine" Clicked="WineClicked" WidthRequest="100" HorizontalOptions="Start"/>
<ListView ItemsSource="{Binding WineList}" x:Name="WineListView" IsVisible="False" HasUnevenRows="True" SeparatorVisibility="None" VerticalOptions="FillAndExpand" VerticalScrollBarVisibility="Always" HeightRequest="1500">
<ListView.ItemTemplate>
<DataTemplate>
<ViewCell>
<Grid x:Name="Wine" RowSpacing="25">
<Grid.RowDefinitions>
<RowDefinition Height="Auto"/>
<RowDefinition Height="*"/>
</Grid.RowDefinitions>
<Grid.ColumnDefinitions>
<ColumnDefinition Width="Auto"/>
<ColumnDefinition Width="*"/>
</Grid.ColumnDefinitions>
<Label Grid.Column="0" Grid.Row="0" Text="{Binding ProductId}" VerticalOptions="End" IsVisible="False"/>
<controls:CircleImage Grid.Column="1" Grid.Row="0" HeightRequest="60" HorizontalOptions="CenterAndExpand" VerticalOptions="Center" Aspect="AspectFill" WidthRequest="66" Grid.RowSpan="2" Source="{Binding Image}"/>
<Label Grid.Column="2" Grid.Row="0" Text="{Binding ProductName}" VerticalOptions="Start"/>
<Label Grid.Column="2" Grid.Row="0" Text="{Binding Description}" VerticalOptions="End"/>
<Label Grid.Column="3" Grid.Row="0" VerticalOptions="Start" Text="{Binding Price, StringFormat='£{0:0.00}'}"/>
<Picker Grid.Column="4" Grid.Row="0" SelectedIndexChanged="WineQuantityChanged" SelectedIndex="{Binding Quantity}">
<Picker.Items>
<x:String>0</x:String>
<x:String>1</x:String>
<x:String>2</x:String>
<x:String>3</x:String>
<x:String>4</x:String>
<x:String>5</x:String>
<x:String>6</x:String>
</Picker.Items>
</Picker>
</Grid>
</ViewCell>
</DataTemplate>
</ListView.ItemTemplate>
</ListView>
從購物車頁面,用戶還可以更改商品的數量,調用 QuantityChanged()-> 調用 UpdateShoppingCartItems() 並使用 ShoppingCartViewModel.ShoppingCartList(保存新數量)重新填充 App.globalShoppingCartOC 說例如他們更改數量,然后返回產品頁面(從左側菜單)。
(應該在 ProductPage 上調用 OnAppearing())並重新加載 VM?
public ShoppingCartPage ()
{
InitializeComponent();
if (App.globalShoppingCartOC != null)
{
foreach (ProductModel Model in App.globalShoppingCartOC)
{
if (Model.Quantity > 0)
{
//populate LV with global shopping OC
Model.SubTotalForItem = Model.Quantity * Model.Price;
ShoppingCartViewModel.ShoppingCartList.Add(Model);//ShoppingCartList is the ListView on xaml
TotalForAllItems += Model.SubTotalForItem;
}
}
}
this.BindingContext = this;
BindingContext = ShoppingCartViewModel;
}
void QuantityChanged(object sender, EventArgs e)
{
//updates correct quantity on screen and calls
//UpdateShoppingCartItems()
}
//update item in shopping cart with new quantity
void UpdateShoppingCartItems(ProductModel product, int quantity)
{
ProductModel result = ShoppingCartViewModel.ShoppingCartList.ToList().Find(x => x.ProductId == product.ProductId);
if (result != null)
{
//remove from list
if (quantity < 1)
{
ShoppingCartViewModel.ShoppingCartList.Remove(result);
}
else // else update new quantity
{
result.Quantity = quantity;
}
}
App.globalShoppingCartOC = ShoppingCartViewModel.ShoppingCartList;
}
//xaml
<StackLayout Grid.Row="0">
<ListView ItemsSource="{Binding ShoppingCartList}" HasUnevenRows="True" SeparatorVisibility="None">
<ListView.Header>
<Button Text="Place Order" Clicked="OrderPlaced_BtnClicked"/>
</ListView.Header>
<ListView.Footer>
<Label x:Name="TotalForItems" HorizontalTextAlignment="End" VerticalTextAlignment="Start" Margin="20,20" FontAttributes="Bold"/>
</ListView.Footer>
<ListView.ItemTemplate>
<DataTemplate>
<ViewCell>
<Grid x:Name="ShoppingCartGrid" RowSpacing="25" ColumnSpacing="10">
<Grid.RowDefinitions>
<RowDefinition Height="Auto"/>
<RowDefinition Height="*"/>
</Grid.RowDefinitions>
<Grid.ColumnDefinitions>
<ColumnDefinition Width="Auto"/>
<ColumnDefinition Width="*"/>
</Grid.ColumnDefinitions>
<Label Grid.Column="0" Text="{Binding Id}" VerticalOptions="End" IsVisible="False"/>
<controls:CircleImage Grid.Column="1" Grid.Row="1" HeightRequest="60" HorizontalOptions="CenterAndExpand" VerticalOptions="CenterAndExpand" Aspect="AspectFill" WidthRequest="66" Grid.RowSpan="2" Source="{Binding Image}"/>
<Label Grid.Column="2" Grid.Row="1" Text="{Binding ProductName}" VerticalOptions="End"/>
<Label Grid.Column="2" Grid.Row="2" VerticalOptions="Start" Text="{Binding Description}"/>
<Label Grid.Column="3" Grid.Row="2" VerticalOptions="Start" Text="{Binding Price, StringFormat='£{0:0.00}'}"/>
<Picker Grid.Column="4" Grid.Row="2" VerticalOptions="Start" SelectedIndexChanged="QuantityChanged" SelectedIndex="{Binding Quantity}">
<Picker.Items>
<x:String>0</x:String>
<x:String>1</x:String>
<x:String>2</x:String>
<x:String>3</x:String>
<x:String>4</x:String>
<x:String>5</x:String>
<x:String>6</x:String>
</Picker.Items>
</Picker>
</Grid>
</ViewCell>
現在,從這里我需要從 productPage_ViewModal.WineList 重新加載來自“WineList”的所有產品,並通過 App.globalShoppingCartOC 進行迭代,更新任何新的數量。
問題是我可以單步執行並且 OnAppearing 執行此操作,但是當頁面重新加載時,它會顯示原始數量,從用戶第一次從產品頁面移動到購物車頁面。 (因此存儲在 DrinksToOrder 中的數量)
修正
更新代碼以在 ProductPage 中包含 OnAppearing
protected override void OnAppearing()
{
base.OnAppearing();
if (App.globalShoppingCartOC != null)
{
if (App.globalShoppingCartOC.Count > 0)
{
foreach(var item in App.globalShoppingCartOC)
{
if(item.Genre == "Wine")
{
productPage_ViewModal.WineList.Where(x => x.ProductId == item.ProductId).FirstOrDefault().Quantity = item.Quantity;
}
}
WineListView.ItemsSource = productPage_ViewModal.WineList;
this.BindingContext = this.productPage_ViewModal;
}
}
}
所以逐步完成這個我可以看到我已經更新了 productPage_ViewModal.WineList 和 WineListView.ItemsSource 中項目的正確數量
然后甚至厭倦了再次綁定上下文...但從購物車頁面返回后,頁面仍然加載原始的 DrinksToPurchaseList
為什么是這樣? 為什么我不能刷新大眾來更新?
Create an event on master page, invoke event from master page in your case blog and consume that event on another page where you want to refresh data..
on master page what you've to do is..
declare event..
public delegate void refreshDelegate();
public static event refreshDelegate refreshEvent;
__
now invoke this event,
refreshEvent?.inovke();
now,
on the page where you want to refresh data, at constructor access the declared event,
masterpage.refreshEvent+=masterpage_refreshEvent;
private void masterpage_refreshEvent()
{
BindData();
}
now, bind the data in invoked event, it'll refresh your screen as expected.
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.