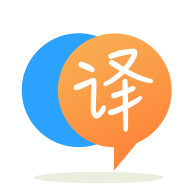
[英]How to get inner for-loop to run all the way through before moving onto next increment of outer loop?
[英]How to get for loop to move onto the next inner list?
我正在嘗試計算嵌套列表中的零數。 我的代碼輸出 2,因為它只通過第一個內部列表。
這是我目前所擁有的:
def e(xlst):
cnt = 0
for numbers in xlst:
for numbers2 in numbers:
if numbers2 == 0:
cnt += 1
return cnt
xlst = [[1,0,0],[1,1,1],[1,1,0]]
e(xlst)
您放錯了return
,它應該與外部循環匹配
return語句在第一個for 內,因此在第一行之后它將返回並退出函數
此外, list 有一個內置函數count ,可用於使代碼更簡單和更快:
from time import time
def e(xlst):
cnt = 0
for numbers in xlst:
for numbers2 in numbers:
if numbers2 == 0:
cnt += 1
return cnt
def with_count(xlst):
cnt = 0
for numbers in xlst:
cnt += numbers.count(0)
return cnt
# Creates a 2D list with 300 rows of [1,0,0]
xlst = [[1,0,0]]*300
t0=time()
print(f'\nfirst function found: {e(xlst)}')
print(f'time: {time() - t0}\n')
t0=time()
print(f'second function found: {with_count(xlst)}')
print(f'time: {time() - t0}\n')
找到的第一個函數:600
時間:0.0003502368927001953
找到的第二個函數:600
時間:0.000194549560546875
更正return
縮進
代碼應該是這樣的
def e(xlst):
cnt = 0
for numbers in xlst:
for numbers2 in numbers:
if numbers2 == 0:
cnt += 1
return cnt
xlst = [[1,0,0],[1,1,1],[1,1,0]]
print(e(xlst))
它打印 3
使用全局變量是不好的做法,但我太累了,不知道如何在不使用兩個函數的情況下做到這一點
def e(xlst):
cnt = 0
if (type(xlst) == list):
for x in xlst:
e(x)
elif (xlst == 0):
cnt +=1
這里我們使用遞歸,在每個嵌套列表上調用函數。 這適用於無限嵌套的列表。
你可以把它作為一個班輪來做。
from itertools import chain
def e(xlst):
return len([i for i in chain.from_iterable(xlst) if i == 0])
xlst = [[1,0,0],[1,1,1],[1,1,0]]
print(e(xlst))
或沒有臨時列表。
def e(xlst):
return sum(1 for i in chain.from_iterable(xlst) if i == 0)
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.