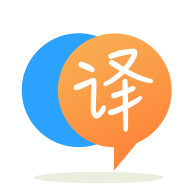
[英]Upgraded to .NET 6, having issues with EF - SqlNullValueException: 'Data is Null. This method or property cannot be called on Null values.'
[英]Data is Null. This method or property cannot be called on Null values. Entity Framework core
我試圖獲取數據列表,但從GetAllList()
方法獲取空值。 在這個項目中,我使用 .Net core 和 ORM Entity Framework core code first method with repository pattern
實體類
public class SIDGenerator
{
public int Id { get; set; }
public string TrackId { get; set; }
public string Track { get; set; }
public string SId { get; set; }
public int InstanceNumber { get; set; }
public string Comment { get; set; }
public bool InUse { get; set; }
public bool IsOtherSysType { get; set; }
public bool IsDeployed { get; set; }
public string Exception { get; set; }
public DateTime ReservationDate { get; set; }
public DateTime LastUpdated { get; set; }
public bool IsDeleted { get; set; }
public bool IsManuallyDefined { get; set; }
public int ReservatedBy { get; set; }
}
界面
public interface ISidGeneratorRepository<T> where T :SIDGenerator
{
T GetById(int id);
/// <returns>List of the entity</returns>
List<T> GetAllList();
}
執行
public class SidGeneratorRepository<T> : ISidGeneratorRepository<T> where T : SIDGenerator
{
private readonly SHIELDDbContext _dbContext;
private readonly ILoggerManager _logger;
private readonly IDateTime _date;
public SidGeneratorRepository(SHIELDDbContext dbContext, ILoggerManager logger, IDateTime date)
{
_dbContext = dbContext;
_logger = logger;
_date = date;
}
public void Delete(SIDGenerator entity)
{
throw new NotImplementedException();
}
public void DeleteById(int id)
{
throw new NotImplementedException();
}
public List<T> GetAllList()
{
var result= _dbContext.Set<T>()//This statement causes exception data is null
.ToList();
return result;
}
public T GetById(int id)
{
var sid = _dbContext.Set<T>()
.FirstOrDefault(i => i.Id == id);
if (sid == null)
{
throw new NotFoundException("Sid could not be found with id " + id.ToString(), id);
}
return sid;
}
}
這是我的 dbcontext 類
public class SHIELDDbContext : DbContext
{
public SHIELDDbContext(DbContextOptions<SHIELDDbContext> options)
: base(options)
{
}
public DbSet<SIDGenerator> SIDGenerator { get; set; }
protected override void OnModelCreating(ModelBuilder modelBuilder)
{
base.OnModelCreating(modelBuilder);
modelBuilder.ApplyConfigurationsFromAssembly(typeof(UserRoleSeedConfig).Assembly);
}
}
下面是我的處理程序類
public Task<GetAllSidViewModel> Handle(GetAllSidQuery request, CancellationToken cancellationToken)
{
try
{
var sidList = _sidRepository.GetAllList();
if (sidList?.Count > 0)
{
var viewModel = new GetAllSidViewModel
{
sIDlist = sidList
};
return Task.FromResult(viewModel);
}
return null;
}
catch (Exception ex)
{
_logger.LogError("Could not retrieve SID LIST from DB" + ex);
return null;
}
}
下面是上述實體的表腳本
CREATE TABLE [dbo].[SIDGenerator](
[Id] [int] IDENTITY(1,1) NOT NULL,
[Track] [nvarchar](max) NULL,
[TrackId] [nvarchar](max) NULL,
[SId] [nvarchar](max) NULL,
[InstanceNumber] [int] NULL,
[Comment] [nvarchar](max) NULL,
[InUse] [bit] NULL,
[IsOtherSysType] [bit] NULL,
[IsDeployed] [bit] NULL,
[Exception] [nvarchar](max) NULL,
[ReservationDate] [datetime2](7) NULL,
[LastUpdated] [datetime2](7) NULL,
[ReservatedBy] [int] NULL,
[IsDeleted] [bit] NULL,
[IsManuallyDefined] [bit] NULL,
CONSTRAINT [PK_SIDGenerators] PRIMARY KEY CLUSTERED
(
[Id] ASC
) WITH (PAD_INDEX = OFF, STATISTICS_NORECOMPUTE = OFF, IGNORE_DUP_KEY = OFF, ALLOW_ROW_LOCKS = ON, ALLOW_PAGE_LOCKS = ON, OPTIMIZE_FOR_SEQUENTIAL_KEY = OFF) ON [PRIMARY]
) ON [PRIMARY] TEXTIMAGE_ON [PRIMARY]
GO
像這樣更改您的模型,或根據您的意願配置可為空的屬性。
public class SIDGenerator
{
public int Id { get; set; }
public string TrackId { get; set; }
public string Track { get; set; }
public string SId { get; set; }
public int? InstanceNumber { get; set; }
public string Comment { get; set; }
public bool? InUse { get; set; }
public bool? IsOtherSysType { get; set; }
public bool? IsDeployed { get; set; }
public string Exception { get; set; }
public DateTime? ReservationDate { get; set; }
public DateTime? LastUpdated { get; set; }
public bool? IsDeleted { get; set; }
public bool? IsManuallyDefined { get; set; }
public int? ReservatedBy { get; set; }
}
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.