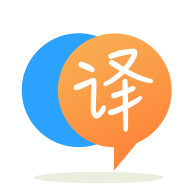
[英]How to get return values and output values from a stored procedure with EF Core?
[英]How to get a list of objects from a stored procedure in EF Core?
我有一個存儲過程,它獲取多個參數,並基於它們調用另一個存儲過程。 返回的列可能因實際調用的存儲過程而異。
因此,我構建了一個包含所有共享列的基類,並使用不同的類對其進行了擴展,每個類都有自己的附加列作為要從存儲過程返回的數據的模型。
以下是類:
public class SP_BaseModel
{
public decimal? Sum { get; set; }
public string Name { get; set; }
public DateTime? BirthDate { get; set; }
}
public class SP_Extended1 : SP_BaseModel
{
public int? Age { get; set; }
public string Hobby { get; set; }
}
我正在像這樣在 DbContext 中注冊上述模型:
protected override void OnModelCreating(ModelBuilder modelBuilder)
{
modelBuilder.Entity<SP_BaseModel>().HasNoKey();
modelBuilder.Entity<SP_Extended1>();
...
}
我已經嘗試了以下兩種方法,但都不起作用:
public IQueryable<T> GetData<T>(string param1, int? patam2 = null, DateTime? param3 = null) where T : SP_BaseModel
{
return Context.Set<T>()
.FromSqlInterpolated($"EXEC SP_GetData {param1},{param2 ?? null},{param3 ?? null}");
}
public async Task<List<T>> GetData<T>(string param1, int? patam2 = null, DateTime? param3 = null) where T : SP_BaseModel
{
return Context.Set<T>()
.FromSqlInterpolated($"EXEC SP_GetData {param1},{param2 ?? null},{param3 ?? null}").ToListAsync();
}
我正在從中創建一個 Nuget,並在客戶端中使用它:
public List<SP_Extended1> GetData(DateTime? today = null)
{
today ??= DateTime.Today;
//This didn't work
var list1 = unitOfWork.MyRepository.GetData<SP_Extended1>(...);
//This didn't work as well
var list2 = unitOfWork.GetData<SP_Extended1>(...).AsEnumerable();
return ...
}
但我收到此錯誤消息:
'FromSqlRaw or FromSqlInterpolated was called with non-composable SQL and with a query composing over it.
Consider calling `AsEnumerable` after the FromSqlRaw or FromSqlInterpolated method to perform the composition on the client-side.'
盡量不要在這里使用繼承。 將SP_Extended1
與基類合並。 EF 不喜歡繼承類。 通常需要大量的血液才能使其發揮作用。 更容易使用獨立的類。 這是一個示例:
我寫了一個特殊的類來從存儲過程中獲取數據
public class AccDef
{
public string AccDefName { get; set; }
public string InvoiceNumber { get; set; }
public DateTime InvoiceDate { get; set; }
public DateTime DeliveryDate { get; set; }
public string ZoneName { get; set; }
public double? Quantity { get; set; }
public double HaulawayAmount { get; set; }
public double FuelAmount { get; set; }
public double SumAmount { get; set; }
[NotMapped]
public string StrQuantity
{
get { return Quantity == null || Quantity == 0 ? "-" : string.Format("{0:F0}", Quantity); }
}
[NotMapped]
public string StrInvoiceDate
{
get { return InvoiceDate.ToString("dd/MM/yyyy"); }
}
[NotMapped]
public string StrDeliveryDate
{
get { return DeliveryDate.ToString("dd/MM/yyyy"); }
}
}
EF上下文
modelBuilder.Entity<AccDef>(e =>
{
e.HasNoKey();
});
這是我使用 EF 從存儲過程中獲取數據的代碼:
public async Task<IEnumerable<AccDef>> GetAccDefReportDetailsData(DateTime endDate)
{
var pEndDate = new SqlParameter("@EndDate", endDate);
return await _context.Set<AccDef>()
.FromSqlRaw("Execute sp_GetAccDefReportDetails @EndDate", parameters: new[] { pEndDate })
.ToArrayAsync();
}
在此之后,我將此數組轉換為我的 EF 模型實體列表。
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.