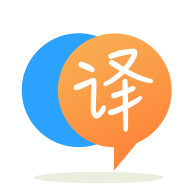
[英]Upload Files to Google Drive using Google Drive API in ASP.Net with C#
[英]Problems with files downloading from Google Drive Api using asp.net mvc
我可以在本地服務器上成功下載文件,但部署后我不能繼續加載而不下載
<a href="~/Home/DownloadFile/@item.Id">Download</a>
家庭控制器.cs
public void DownloadFile(string id)
{
string FilePath = DownloadGoogleFile(id);
Response.AddHeader("Content-Disposition", "attachment; filename=" + Path.GetFileName(FilePath));
Response.WriteFile(System.Web.Hosting.HostingEnvironment.MapPath("/GoogleDriveFiles/" + Path.GetFileName(FilePath)));
Response.End();
Response.Flush();
}
static string DownloadGoogleFile(string fileId)
{
Google.Apis.Drive.v3.DriveService service = GetService();
string FolderPath = System.Web.Hosting.HostingEnvironment.MapPath("/GoogleDriveFiles/");
Google.Apis.Drive.v3.FilesResource.GetRequest request = service.Files.Get(fileId);
string FileName = request.Execute().Name;
string FilePath = System.IO.Path.Combine(FolderPath, FileName);
MemoryStream stream1 = new MemoryStream();
request.MediaDownloader.ProgressChanged += (Google.Apis.Download.IDownloadProgress progress) =>
{
switch (progress.Status)
{
case DownloadStatus.Downloading:
{
Console.WriteLine(progress.BytesDownloaded);
break;
}
case DownloadStatus.Completed:
{
Console.WriteLine("Download complete.");
SaveStream(stream1, FilePath);
break;
}
}
};
request.Download(stream1);
return FilePath;
}
public static Google.Apis.Drive.v3.DriveService GetService()
{
var CSPath = System.Web.Hosting.HostingEnvironment.MapPath("~/");
UserCredential credential;
using (var stream = new FileStream(Path.Combine(CSPath, "client_secret.json"), FileMode.Open, FileAccess.Read))
{
String FilePath = Path.Combine(CSPath, "DriveServiceCredentials.json");
credential = GoogleWebAuthorizationBroker.AuthorizeAsync(
GoogleClientSecrets.Load(stream).Secrets,
Scopes,
"user",
CancellationToken.None,
new FileDataStore(FilePath, true)).Result;
}
Google.Apis.Drive.v3.DriveService service = new Google.Apis.Drive.v3.DriveService(new BaseClientService.Initializer()
{
HttpClientInitializer = credential,
ApplicationName = "GoogleDriveRestAPI-v3",
});
return service;
}
我應該改變什么? 我正在嘗試從谷歌獲取文件,它自己而不是從瀏覽器獲取文件是 SSL 證書並確保 web 與此有關,因為我的 web 現在不安全?
向 Google 進行身份驗證時,安裝的應用程序和 web 應用程序之間存在差異。 安裝好的應用可以在本機瀏覽器打開window授權,Web應用需要在用戶機器打開web瀏覽器授權。 GoogleWebAuthorizationBroker.AuthorizeAsync
設計用於已安裝的應用程序。 它將在服務器上打開 web 瀏覽器進行身份驗證和授權,但無法正常工作。
對於 web 應用程序,您應該使用GoogleAuthorizationCodeFlow
using System;
using System.Web.Mvc;
using Google.Apis.Auth.OAuth2;
using Google.Apis.Auth.OAuth2.Flows;
using Google.Apis.Auth.OAuth2.Mvc;
using Google.Apis.Drive.v2;
using Google.Apis.Util.Store;
namespace Google.Apis.Sample.MVC4
{
public class AppFlowMetadata : FlowMetadata
{
private static readonly IAuthorizationCodeFlow flow =
new GoogleAuthorizationCodeFlow(new GoogleAuthorizationCodeFlow.Initializer
{
ClientSecrets = new ClientSecrets
{
ClientId = "PUT_CLIENT_ID_HERE",
ClientSecret = "PUT_CLIENT_SECRET_HERE"
},
Scopes = new[] { DriveService.Scope.Drive },
DataStore = new FileDataStore("Drive.Api.Auth.Store")
});
public override string GetUserId(Controller controller)
{
// In this sample we use the session to store the user identifiers.
// That's not the best practice, because you should have a logic to identify
// a user. You might want to use "OpenID Connect".
// You can read more about the protocol in the following link:
// https://developers.google.com/accounts/docs/OAuth2Login.
var user = controller.Session["user"];
if (user == null)
{
user = Guid.NewGuid();
controller.Session["user"] = user;
}
return user.ToString();
}
public override IAuthorizationCodeFlow Flow
{
get { return flow; }
}
}
}
在此處查看完整示例
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.