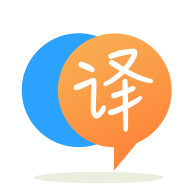
[英]CarouselView with a different template for each slide in Xamarin forms
[英]how to display different content for each view in carouselview xamarin.forms
這是我的初始代碼
<CarouselView x:Name="BGcarousel" >
<CarouselView.ItemTemplate>
<DataTemplate>
<Grid RowSpacing="0">
<Grid.RowDefinitions>
<RowDefinition Height="*"/>
</Grid.RowDefinitions>
<Image Grid.Row="0" Grid.Column="0"
Source="{Binding BackgroundImage}"
HorizontalOptions="FillAndExpand"
VerticalOptions="FillAndExpand"
Aspect="AspectFill" />
<Frame
Grid.Row="0"
Grid.Column="0"
VerticalOptions="Center"
HorizontalOptions="Center"
HeightRequest="30"
WidthRequest="120"
CornerRadius="60"
HasShadow="True"
BackgroundColor="BlueViolet">
<Label
Text="{Binding Icon}"
TextColor="Black"
VerticalOptions="Center"
HorizontalOptions="Center"
FontAttributes="Bold"
FontSize="23"
Padding="0" />
</Frame>
<Label Grid.Row="0" Grid.Column="0"
Text="{Binding Quot}"
HorizontalOptions="FillAndExpand"
VerticalOptions="FillAndExpand"
HorizontalTextAlignment="Center"
VerticalTextAlignment="End"
Padding="0,0,0,50"
TextColor="White"
FontSize="30"/>
</Grid>
</DataTemplate>
</CarouselView.ItemTemplate>
</CarouselView>
這看起來每個頁面都有相同的內容,但我只需要在最后添加一個按鈕並控制它的行為微軟剛剛添加了一個輪播視圖作為一項功能,我是新手,任何人都可以幫助我
解決方案1 :如果您想在carouselview xamarin.forms 中為每個視圖使用其他不同的布局,我建議您使用DataTemplateSelector
來實現它。
首先,您可以為CarouselView
創建兩個DataTemplate
(如果您願意,您可以創建很多DataTemplate
)。
<ContentPage.Resources>
<DataTemplate x:Key="AmericanMonkeyTemplate">
<StackLayout>
<Frame HasShadow="True"
BorderColor="DarkGray"
CornerRadius="5"
Margin="20"
HeightRequest="300"
HorizontalOptions="Center"
VerticalOptions="CenterAndExpand">
<StackLayout>
<!--Add layout that you want-->
<Label Text="{Binding Name}"
FontAttributes="Bold"
FontSize="Large"
HorizontalOptions="Center"
VerticalOptions="Center" />
<Label Text="{Binding Location}"
HorizontalOptions="Center" />
<Label Text="{Binding Details}"
FontAttributes="Italic"
HorizontalOptions="Center"
MaxLines="5"
LineBreakMode="TailTruncation" />
</StackLayout>
</Frame>
</StackLayout>
</DataTemplate>
<DataTemplate x:Key="OtherMonkeyTemplate">
<!--Add layout that you want-->
<StackLayout>
<Frame HasShadow="True"
BorderColor="DarkGray"
CornerRadius="5"
Margin="20"
HeightRequest="300"
HorizontalOptions="Center"
VerticalOptions="CenterAndExpand">
<StackLayout>
<Label Text="{Binding Name}"
FontAttributes="Bold"
FontSize="Large"
HorizontalOptions="Center"
VerticalOptions="Center" />
<Label Text="{Binding Location}"
HorizontalOptions="Center" />
<Label Text="{Binding Details}"
FontAttributes="Italic"
HorizontalOptions="Center"
MaxLines="5"
LineBreakMode="TailTruncation" />
<Button
Text="Click"
/>
<Button
Text="Click"
/>
<Button
Text="Click"
/>
</StackLayout>
</Frame>
</StackLayout>
</DataTemplate>
<controls:MonkeyDataTemplateSelector x:Key="MonkeySelector"
AmericanMonkey="{StaticResource AmericanMonkeyTemplate}"
OtherMonkey="{StaticResource OtherMonkeyTemplate}" />
</ContentPage.Resources>
<StackLayout>
<CarouselView ItemsSource="{Binding Monkeys}" ItemTemplate="{StaticResource MonkeySelector}">
</CarouselView>
</StackLayout>
</ContentPage>
然后創建一個DataTemplateSelector
,您可以依賴一個屬性來顯示哪個DataTemple
布局。 例如,如果Location
是 Eurp,我將其設置為 OtherMonkey DataTemple
,其他人設置 America DataTemple
。
public class MonkeyDataTemplateSelector : DataTemplateSelector
{
public DataTemplate AmericanMonkey { get; set; }
public DataTemplate OtherMonkey { get; set; }
protected override DataTemplate OnSelectTemplate(object item, BindableObject container)
{
return ((Monkey)item).Location.Contains("Eurp") ? OtherMonkey : AmericanMonkey;
}
}
解決方案2同意Jason,您可以將IsVisible
用於按鈕,使用ButtonIsVisable
綁定屬性,如下代碼所示。
<Button
Text="Click"
IsVisible="{Binding ButtonIsVisable}"
/>
然后在您的模型中添加ButtonIsVisable
屬性。
public class Monkey
{
public string Name { get; set; }
public string Location { get; set; }
public string Details { get; set; }
public bool ButtonIsVisable { get; set; }
}
在 ViewModel 中,您可以控制 Button 是否可見。
public class MyViewModel
{
public ObservableCollection<Monkey> Monkeys { get; set; }
public MyViewModel()
{
Monkeys =new ObservableCollection<Monkey>();
Monkeys.Add(new Monkey() { ButtonIsVisable=false, Details="Details1", Location="Usa", Name="Test1" });
Monkeys.Add(new Monkey() { ButtonIsVisable = false, Details = "Details2", Location = "Asia", Name = "Test2" });
Monkeys.Add(new Monkey() { ButtonIsVisable = true, Details = "Details3", Location = "Eurp", Name = "Test3" });
}
}
在布局中添加按鈕。
<CarouselView ItemsSource="{Binding Monkeys}">
<CarouselView.ItemTemplate>
<DataTemplate>
<StackLayout>
<Frame HasShadow="True"
BorderColor="DarkGray"
CornerRadius="5"
Margin="20"
HeightRequest="300"
HorizontalOptions="Center"
VerticalOptions="CenterAndExpand">
<StackLayout>
<Label Text="{Binding Name}"
FontAttributes="Bold"
FontSize="Large"
HorizontalOptions="Center"
VerticalOptions="Center" />
<Label Text="{Binding Location}"
HorizontalOptions="Center" />
<Label Text="{Binding Details}"
FontAttributes="Italic"
HorizontalOptions="Center"
MaxLines="5"
LineBreakMode="TailTruncation" />
<Button
Text="Click"
IsVisible="{Binding ButtonIsVisable}"
/>
</StackLayout>
</Frame>
</StackLayout>
</DataTemplate>
</CarouselView.ItemTemplate>
</CarouselView>
這是布局背景代碼。
public MainPage()
{
InitializeComponent();
this.BindingContext = new MyViewModel();
}
這里正在運行 GIF。
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.