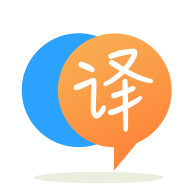
[英]asp.net mvc 5 How to get id from url and save it in another table
[英]How to get an Id from one table and save it to another table in ASP.NET Core, repository pattern
這是安排考試服務:
public int AddSchedule(ScheduleExamViewModel schedule)
{
var newSchedule = new ScheduleExam()
{
ExamDate = schedule.ExamDate,
SubjectId = schedule.SubjectId,
StudentId = schedule.StudentId,
Status = schedule.Status
};
_context.ScheduleExams.Add(newSchedule);
_context.SaveChanges();
return newSchedule.Id;
}
這是安排考試控制器:
// GET: ScheduleExamController/Create
public IActionResult Create()
{
var model = new ScheduleExamViewModel();
ViewBag.Subject = new SelectList(_isubject.GetSubject(), "Id", "Name");
ViewBag.Student = new SelectList(_istudent.GetStudent(), "Id", "Name");
return View(model);
}
// POST: ScheduleExamController/Create
[HttpPost]
[ValidateAntiForgeryToken]
public IActionResult Create(ScheduleExamViewModel schedule)
{
if (ModelState.IsValid)
{
int id = _ischeduleExam.AddSchedule(schedule);
if (id > 0)
{
return RedirectToAction(nameof(Create));
}
}
ViewBag.Subject = new SelectList(_isubject.GetSubject(), "Id", "Name");
ViewBag.Student = new SelectList(_istudent.GetStudent(), "Id", "Name");
return View(schedule);
}
我想通過單擊“安排新考試”來獲取學生的 ID 並將其保存到安排考試表中
這是 Student 表的index.cshtml
:
<table class="table table-hover table-bordered table-condensed">
<thead class="table-color text-white">
<tr>
<th>
@Html.DisplayNameFor(model => model.Id)
</th>
<th>
@Html.DisplayNameFor(model => model.Name)
</th>
<th>
@Html.DisplayNameFor(model => model.LastName)
</th>
<th>
@Html.DisplayNameFor(model => model.Email)
</th>
<th>Schedule New Exam</th>
<th>Properties</th>
</tr>
</thead>
<tbody>
@foreach (var item in Model)
{
<tr>
<td>
@Html.DisplayFor(modelItem => item.Id)
</td>
<td>
@Html.DisplayFor(modelItem => item.Name)
</td>
<td>
@Html.DisplayFor(modelItem => item.LastName)
</td>
<td>
@Html.DisplayFor(modelItem => item.Email)
</td>
<td>
<a asp-action="Create" asp-controller="ScheduleExam", asp-route-id="@item.Id">Schedule New Exam</a>
</td>
<td>
@Html.ActionLink(" ", "Edit", new { id = item.Id }, new { @class = "fa fa-edit", title = "Edit" }) |
@Html.ActionLink(" ", "Details", new { id = item.Id }, new { @class = "fa fa-info-circle", title = "More details" }) |
@Html.ActionLink(" ", "Delete", new { id = item.Id }, new { @class = "fa fa-trash", title = "Delete" })
</td>
</tr>
}
</tbody>
</table>
這是日程安排考試的 create.csthml 頁面:
<form asp-action="Create">
<div asp-validation-summary="ModelOnly" class="text-danger"></div>
<div class="form-group">
<label asp-for="ExamDate" class="control-label"></label>
<input asp-for="ExamDate" class="form-control" />
<span asp-validation-for="ExamDate" class="text-danger"></span>
</div>
<div class="form-group">
<label asp-for="StudentId" class="control-label"></label>
<input asp-for="StudentId" class="form-control" value="@ViewBag.model.Id" />
<span asp-validation-for="StudentId" class="text-danger"></span>
</div>
<div class="input-group">
<div class="input-group-prepend">
<span class="input-group-text"><strong>Subject:</strong></span>
</div><br />
<select asp-for="SubjectId" class="form-control input-hover" asp-items="ViewBag.Subject">
<option value="">Please choose a subject...</option>
</select>
<span asp-validation-for="SubjectId " class="text-danger"></span>
</div><br />
<div class="form-group">
<input type="submit" value="Create" class="btn btn-primary" />
</div>
</form>
但是當我單擊 Student 表的 index.cshtml 中的 Schedule New 考試時,我收到此錯誤:
RuntimeBinderException:無法對空引用執行運行時綁定 CallSite.Target(Closure , CallSite , object )
CallSite.Target(Closure, CallSite, object) System.Dynamic.UpdateDelegates.UpdateAndExecute1<T0, TRet>(CallSite site, T0 arg0)
Create.cshtml 中的 AspNetCore.Views_ScheduleExam_Create.b__22_0() +
<input asp-for="StudentId" class="form-control" value="@ViewBag.model.Id" />
請詳細解決它,我正在使用使用存儲庫模式的 ASP.NET Core 3.1 MVC。
謝謝
首先,您應該在 Create-Action 中獲得一個 Id 並為學生設置:
public IActionResult Create(int Id)
{
var model = new ScheduleExamViewModel();
ViewBag.Subject = new SelectList(_isubject.GetSubject(), "Id", "Name");
ViewBag.Student = new SelectList(_istudent.GetStudent(), "Id", "Name" , Id);
return View(model);
}
其次,我在您的視圖中找不到學生的下拉列表。 我認為您應該在 ViewModel 的控制器中設置 Id。
var model = new ScheduleExamViewModel();
mode.Id = Id;
第三,您將模型傳遞給視圖,您可以從模型中獲取信息。
@model ScheduleExamViewModel
<input asp-for="StudentId" class="form-control" value="@Model.Id" />
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.