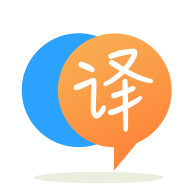
[英]Inject SignalR IHubContext into controller with Autofac
[英]Inject SignalR IHubContext into service layer with Autofac
在運行 Framework 4.72 而不是 .NET Core 的應用程序中,我試圖將 SignalR IHubContext 注入到 Web API 2.x 服務中。 我的解決方案分為三個項目,Web、服務、數據。 SignalR 集線器位於 Web 層。 我有在服務層運行的后台代碼,完成后我需要它通過集線器發送消息。 此后台任務不是由控制器啟動的。
我的 Global.asax 非常標准:
protected void Application_Start()
{
GlobalConfiguration.Configure(WebApiConfig.Register);
// Set JSON serializer to use camelCase
var json = GlobalConfiguration.Configuration.Formatters.JsonFormatter;
json.SerializerSettings.ContractResolver = new CamelCasePropertyNamesContractResolver();
DIConfig.Setup();
FilterConfig.RegisterGlobalFilters(GlobalFilters.Filters);
var logConfigFilePath = Server.MapPath("~/log4net.config");
log4net.Config.XmlConfigurator.ConfigureAndWatch(new System.IO.FileInfo(logConfigFilePath));
}
我的 DIConfig 包含:
internal static void Setup()
{
var config = System.Web.Http.GlobalConfiguration.Configuration;
var builder = new ContainerBuilder();
builder.Register(c => new ShopAPDbContext()).AsImplementedInterfaces().InstancePerBackgroundJob().InstancePerLifetimeScope();
builder.RegisterType<ShopAPRepository>().As<IShopAPRepository>().InstancePerBackgroundJob().InstancePerLifetimeScope();
builder.RegisterType<ShopAPService>().As<IShopAPService>().InstancePerBackgroundJob().InstancePerLifetimeScope();
builder.AddAutoMapper(typeof(InvoiceMappingProfile).Assembly);
builder.RegisterApiControllers(Assembly.GetExecutingAssembly());
var container = builder.Build();
DependencyResolver.SetResolver(new AutofacDependencyResolver(container));
config.DependencyResolver = new AutofacWebApiDependencyResolver(container);
Hangfire.GlobalConfiguration.Configuration.UseAutofacActivator(container);
}
還有我的 Startup.cs:
public class Startup
{
public void Configuration(IAppBuilder app)
{
var container = DependencyConfiguration.Configure(app);
SignalRConfiguration.Configure(app, container);
HangFireDashboardConfig.Configure(app);
}
}
public static class DependencyConfiguration
{
public static IContainer Configure(IAppBuilder app)
{
var builder = new ContainerBuilder();
builder.RegisterHubs(typeof(SignalRConfiguration).Assembly);
var container = builder.Build();
app.UseAutofacMiddleware(container);
return container;
}
}
public static class SignalRConfiguration
{
public static void Configure(IAppBuilder app, IContainer container)
{
HubConfiguration config = new HubConfiguration();
config.Resolver = new AutofacDependencyResolver(container);
app.Map("/messages", map =>
{
map.UseCors(CorsOptions.AllowAll);
var hubConfiguration = new HubConfiguration
{
EnableDetailedErrors = true,
EnableJavaScriptProxies = false
};
map.RunSignalR(hubConfiguration);
});
}
}
我的服務層的構造函數如下所示:
public ShopAPService()
{
_shopAPRepository = new ShopAPRepository();
_mapper = new Mapper((IConfigurationProvider)typeof(InvoiceMappingProfile).Assembly);
_hubContext = null; // what here?
}
public ShopAPService(IShopAPRepository shopAPRepository, IMapper mapper, IHubContext hubContext)
{
_shopAPRepository = shopAPRepository;
_mapper = mapper;
_hubContext = hubContext;
}
我知道我需要將 IHubContext 的一個實例傳遞到服務中,但到目前為止我還沒有成功。 據我所知,第一個構造函數是從控制器以外的任何東西調用服務時使用的構造函數?
第一次修訂
好的,我知道所有東西都應該放在一個容器中。 根據反饋和查看這些鏈接,我創建了一個容器並將其傳遞。 這是我修改后的啟動:
public class Startup
{
public void Configuration(IAppBuilder app)
{
//var config = System.Web.Http.GlobalConfiguration.Configuration;
var container = GetDependencyContainer();
RegisterWebApi(app, container);
RegisterSignalR(app, container);
GlobalHost.DependencyResolver = new AutofacDependencyResolver(container);
HubConfiguration config = new HubConfiguration();
config.Resolver = new AutofacDependencyResolver(container);
//config.DependencyResolver = new AutofacWebApiDependencyResolver(container);
Hangfire.GlobalConfiguration.Configuration.UseAutofacActivator(container);
HangFireDashboardConfig.Configure(app);
}
private IContainer GetDependencyContainer()
{
return AutofacConfig.RegisterModules();
}
private void RegisterWebApi(IAppBuilder app, IContainer container)
{
var configuration = new HttpConfiguration
{
DependencyResolver = new AutofacWebApiDependencyResolver(container)
};
WebApiConfig.Register(configuration);
app.UseAutofacMiddleware(container);
app.UseAutofacWebApi(configuration);
app.UseWebApi(configuration);
}
private void RegisterSignalR(IAppBuilder app, IContainer container)
{
var configuration = new HubConfiguration
{
Resolver = new AutofacDependencyResolver(container)
};
app.MapSignalR(configuration);
}
}
我的 AutofacConfig 構建容器並完成大部分注冊:
internal class AutofacConfig
{
public static IContainer RegisterModules()
{
var builder = new ContainerBuilder();
builder.Register(c => new ShopAPDbContext()).AsImplementedInterfaces().InstancePerBackgroundJob().InstancePerLifetimeScope();
builder.RegisterType<ShopAPRepository>().As<IShopAPRepository>().InstancePerBackgroundJob().InstancePerLifetimeScope();
builder.RegisterType<ShopAPService>().As<IShopAPService>().InstancePerBackgroundJob().InstancePerLifetimeScope();
builder.RegisterAutoMapper(typeof(InvoiceMappingProfile).Assembly);
builder.RegisterApiControllers(Assembly.GetExecutingAssembly());
// Register Autofac resolver into container to be set into HubConfiguration later
builder.RegisterType<AutofacDependencyResolver>().As<IDependencyResolver>().SingleInstance();
// Register ConnectionManager as IConnectionManager so that you can get hub context via IConnectionManager injected to your service
builder.RegisterType<ConnectionManager>().As<IConnectionManager>().SingleInstance();
builder.RegisterHubs(Assembly.GetExecutingAssembly());
var container = builder.Build();
return container;
}
}
但是,我仍然無法在我的服務中獲得對集線器的引用(它位於與 API 不同的項目中,但在相同的解決方案中。
我的 Service 方法是從 HangFire 調用的,沒有對集線器的引用。 如何在我的無參數構造函數中引用它?
public partial class ShopAPService : IShopAPService
{
private static readonly ILog _log = LogManager.GetLogger(System.Reflection.MethodBase.GetCurrentMethod().DeclaringType);
readonly IShopAPRepository _shopAPRepository;
readonly IMapper _mapper;
private IHubContext _hubContext;
public ShopAPService()
{
_shopAPRepository = new ShopAPRepository();
_mapper = new Mapper((IConfigurationProvider)typeof(InvoiceMappingProfile).Assembly);
_hubContext = connectionManager.GetHubContext<MessageHub>();
}
public ShopAPService(IShopAPRepository shopAPRepository, IMapper mapper, IHubContext hubContext)
{
_shopAPRepository = shopAPRepository;
_mapper = mapper;
_hubContext = hubContext;
}
}
您無法直接解析IHubContext
。 但是您可以解析此接口的通用實現。 此處和此處描述的更多詳細信息。
我只是創建了非常簡單的OWIN
(Stratup.cs) 實現。 我已經安裝了Autofac.SignalR塊包並使用方法RegisterHubs進行注冊和方法MapSignalR進行映射。 這是標准方法,解決類型化集線器實現很重要。
但是,如果您希望解析上下文更正確,那么您需要再添加兩個注冊: AutofacDependencyResolver和ConnectionManager ( 此處提供更多信息)。
請查看完整樣本:
using Autofac;
using Autofac.Integration.SignalR;
using Autofac.Integration.WebApi;
using Microsoft.AspNet.SignalR;
using Microsoft.Owin;
using Owin;
using System.Reflection;
using System.Web.Http;
[assembly: OwinStartup(typeof(Startup))]
namespace Sample
{
public class Startup
{
public void Configuration(IAppBuilder app)
{
var container = GetDependencyContainer();
RegisterWebApi(app, container);
RegisterSignalR(app, container);
}
private IContainer GetDependencyContainer()
{
var builder = new ContainerBuilder();
AutofacConfig.RegisterModules(builder);
builder.RegisterApiControllers(Assembly.GetExecutingAssembly());
builder.RegisterHubs(Assembly.GetExecutingAssembly());
// Register Autofac resolver into container to be set into HubConfiguration later
builder.RegisterType<AutofacDependencyResolver>().As<IDependencyResolver>().SingleInstance();
// Register ConnectionManager as IConnectionManager so that you can get hub context via IConnectionManager injected to your service
builder.RegisterType<ConnectionManager>().As<IConnectionManager>().SingleInstance();
var container = builder.Build();
return container;
}
private void RegisterWebApi(IAppBuilder app, IContainer container)
{
var configuration = new HttpConfiguration
{
DependencyResolver = new AutofacWebApiDependencyResolver(container)
};
WebApiConfig.Register(configuration);
app.UseAutofacMiddleware(container);
app.UseAutofacWebApi(configuration);
app.UseWebApi(configuration);
}
private void RegisterSignalR(IAppBuilder app, IContainer container)
{
var configuration = new HubConfiguration
{
Resolver = new AutofacDependencyResolver(container)
};
app.MapSignalR(configuration);
}
}
}
我的集線器是標准且簡單的:
public class MaintenanceHub : Hub
{
public MaintenanceHub(IMaintenanceLogProvider maintenanceLogProvider)
{
maintenanceLogProvider.TaskProgressStatusEvent += (s, e) => GetTaskLogStatus(e);
}
public void GetTaskLogStatus(LongTaskProgressStatus taskProgressStatus)
{
Clients.All.getTaskLogStatus(taskProgressStatus);
}
}
注冊后,您可以使用集線器注入上下文。 類似的東西(可用 2 個選項,您只能使用您想要的一個):
public AccountController(IConnectionManager connectionManager, MaintenanceHub maintenanceHub)
{
var context = connectionManager.GetHubContext<MaintenanceHub>();
var hub = maintenanceHub;
}
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.