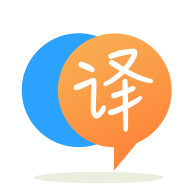
[英]Calling REST service via HttpClient from ASP.net 4 Web API 2 project results in exception
[英]HTTPClient error when calling ASP.NET Web API REST service from ASP.NET MVC app
I have an ASP.NET MVC application which is invoking a ASP.NET Web Api REST service:
public class MyClass
{
private static HttpClient client = new HttpClient();
public async Task DumpWarehouseDataIntoFile(Warehouse myData, string path, string filename) // See Warehouse class later in this post
{
try
{
//Hosted web API REST Service base url
string Baseurl = "http://XXX.XXX.XX.X:YYYY/";
//using (var client = new HttpClient()) --> I have declared client as an static variable
//{
//Passing service base url
client.BaseAddress = new Uri(Baseurl);
client.DefaultRequestHeaders.Clear();
//Define request data format
client.DefaultRequestHeaders.Accept.Add(new MediaTypeWithQualityHeaderValue("application/json"));
// Serialize parameter to pass to the asp web api rest service
string jsonParam = Newtonsoft.JsonConvert.SerializeObject(myData);
//Sending request to find web api REST service resource using HttpClient
var httpContent = new StringContent(jsonParam, Encoding.UTF8, "application/json");
HttpResponseMessage Res = await client.PostAsync("api/Warehouse/DumpIntoFile", httpContent);
//Checking the response is successful or not which is sent using HttpClient
if (Res.IsSuccessStatusCode)
{
// Some other sftuff here
}
//}
}
catch (Exception ex)
{
// Do some stuff here
} // End Try
} // End DumpWarehouseDataIntoFile method
} // End class
The ASP.NET Web API REST Controller:
public class WarehouseController : ApiController
{
public bool DumpIntoFile(string data)
{
// Stuff here
}
}
我使用了 HttpClient static 變量,我沒有將它包含在“使用”塊中,因為不推薦使用此鏈接中的說明: 您正在使用 HTTPCLIENT 錯誤,它正在破壞您的軟件
執行此代碼時,它會失敗並出現錯誤:
System.Net.Http.HttpRequestException:發送請求時出錯。 System.Net.WebException:無法解析遠程名稱:'xxx'
我想我不需要處理 HttpClient 客戶端 object,對吧?
我該如何解決這個問題?
我正在使用 Visual Studio 2013 和 NET 4.5(非核心)
UPDATE 2020/12/10 : Previous error was occurring because of ASP.NET Web API REST Url was not configured correctly in the internal DNS.
現在解決先前的錯誤后,我得到以下錯誤:
404 未找到
下面是client.PostAsync返回的一些錯誤消息:
Ping 到 ASP.NET Web API Z55276C10D84E1DF7713B441E76E141 正在工作。
也許我的 web api 路由配置在下面的 App_Start\WebApiConfig.cs 下缺少一些東西?
public static class WebApiConfig
{
public static void Register(HttpConfiguration config)
{
// Configuración y servicios de API web
// Rutas de API web
config.MapHttpAttributeRoutes();
config.Routes.MapHttpRoute(
name: "DefaultApi",
routeTemplate: "api/{controller}/{id}",
defaults: new { id = RouteParameter.Optional }
);
}
}
可能的看點:
檢查您的 WebApi 文檔。 web API 中的 404 表示主機無法訪問或您調用的端點錯誤。 首先通過在瀏覽器中粘貼 URL 來檢查。 如果它是一個 POST 操作並且您的機器可以訪問,那么您將收到一個method not supported
的錯誤。
如果在瀏覽器中,如果它再次出現 404 但您知道它是正確的,那么您應該查看機器的 ACL(用戶授權和身份驗證)。 該機器是否可訪問/可訪問您的網絡。
我在您的代碼中看不到任何授權標頭。 請也檢查一下。
很少可能 - 如果它需要一些預配置的應用程序,如 Facebook、Google 等。
我假設您正在使用基於您提供的WebApiConfig
的 Web API 2。 關於您收到的 404 響應,這完全源於您的 controller 操作中路由的不正確使用或缺少路由。
使用您的示例 WebApiConfig,這將啟用屬性路由以及使用 Web API 的默認路由模板的基於約定的路由。 默認模板不會根據動作名稱匹配,它僅根據請求的 controller 名稱和 HTTP 方法(GET、POST 等)匹配。 示例GET http://localhost:5000/api/books/2
將路由到BooksController
並調用與命名約定匹配的操作方法,即GetBookById(int id)
。
config.MapHttpAttributeRoutes();
config.Routes.MapHttpRoute(
name: "DefaultApi",
routeTemplate: "api/{controller}/{id}",
defaults: new { id = RouteParameter.Optional }
);
在您的情況下,您有一個名為DumpIntoFile(string data)
的操作方法,它不遵循上述標准命名約定,因此路由系統不知道要調用哪個操作。 您可以使用在配置中啟用的屬性路由來解決此問題。 您需要向您的操作方法添加一個屬性,該屬性指定 HTTP 動詞以及應該調用該方法的路由。 請注意應添加到 controller class 的附加屬性,該屬性指定路由前綴。
[RoutePrefix("api/warehouse")]
public class WarehouseController : ApiController
{
// Routes POST http://localhost:5000/api/warehouse/dump
[Route("dump")]
[HttpPost]
public bool DumpIntoFile(string data)
{
//...
}
}
這會將 POST 請求路由到http://localhost:5000/api/warehouse/dump
。 顯然localhost:5000
是您的基本 uri 的占位符。 我不知道請求中的data
有多復雜,但您可以參考有關如何處理請求正文中的數據的文檔。 該示例主要關注路由部分。
這只是一個簡單的示例,您可以使用各種不同的屬性來指定路由、接受的方法等。 文檔很好地概述了它並提供了大量示例。 下面提供了鏈接。 另外,我建議返回IHttpActionResult
或HttpResponseMessage
類型之一,而不是簡單的 bool/string/int/etc。 它只是為您返回的 HTTP 響應類型提供了更多選項,這些也在文檔中。
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.