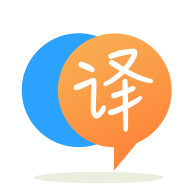
[英]boost graph library, depth_first_search not calling finish_edge in msvc
[英]Have trouble supplying Color Map to depth first search in boost graph library
我正在嘗試從特定頂點開始使用深度優先搜索。 為此,我需要提供一個訪客和一個顏色 map。
如果我不提供起始頂點,那么我也不需要提供顏色 map 並且一切正常。 但是,我沒有找到可以接受起始頂點而不需要顏色 map 的簽名。
我使用 C++ 17(Embarcadero C++ Builder)這是代碼:
#include <stdio.h>
#include <boost/graph/graph_traits.hpp>
#include <boost/graph/adjacency_list.hpp>
#include <boost/graph/breadth_first_search.hpp>
#include <boost/graph/depth_first_search.hpp>
#include <boost/graph/topological_sort.hpp>
struct EdgeProps
{
int value;
};
struct VertexProps
{
int value;
};
struct cycle_detector : public boost::default_dfs_visitor
{
cycle_detector( bool& has_cycle)
: _has_cycle(has_cycle) { }
template <class Edge, class Graph>
void back_edge(Edge, Graph&) {
_has_cycle = true;
}
protected:
bool& _has_cycle;
};
int _tmain(int argc, _TCHAR* argv[])
{
typedef boost::adjacency_list<boost::setS, boost::vecS, boost::bidirectionalS, VertexProps, EdgeProps> Graph;
Graph g;
auto v0 = boost::add_vertex(g);
auto v1 = boost::add_vertex(g);
auto v2 = boost::add_vertex(g);
auto v3 = boost::add_vertex(g);
auto v4 = boost::add_vertex(g);
boost::add_edge(v0, v1, g);
boost::add_edge(v0, v2, g);
boost::add_edge(v0, v3, g);
boost::add_edge(v2, v4, g);
boost::add_edge(v3, v4, g);
bool has_cycle = false;
std::vector<boost::default_color_type> colors(boost::num_vertices(g));
boost::iterator_property_map color_map(colors.begin(), boost::get(boost::vertex_index, g));
boost::depth_first_search(g, boost::visitor(cycle_detector(has_cycle)), color_map, v0);
return 0;
}
我收到一連串抱怨訪客的錯誤消息。 然而,如果我不提供 map 和一個起始頂點,那么一切都很好。
以下是消息:
bcc32c command line for "DFS.cpp"
c:\program files (x86)\embarcadero\studio\21.0\bin\bcc32c.exe -cc1 -D _DEBUG -output-dir .\Win32\Debug -I D:\Study\Trees -I D:\Study\Trees -isystem
"c:\program files (x86)\embarcadero\studio\21.0\include" -isystem "c:\program files (x86)\embarcadero\studio\21.0\include\dinkumware64" -isystem
"c:\program files (x86)\embarcadero\studio\21.0\include\windows\crtl" -isystem "c:\program files (x86)\embarcadero\studio\21.0\include\windows\sdk"
-isystem "c:\program files (x86)\embarcadero\studio\21.0\include\windows\rtl" -isystem "c:\program files
(x86)\embarcadero\studio\21.0\include\windows\vcl" -isystem "c:\program files (x86)\embarcadero\studio\21.0\include\windows\fmx" -isystem
C:\Users\Public\Documents\Embarcadero\Studio\21.0\hpp\Win32 -isystem "c:\program files (x86)\embarcadero\studio\21.0\include\boost_1_70" -isystem
"D:\Icrfs\Components\TeeChart 2020.30\Compiled\Delphi27.win32\Include" -isystem "D:\ICRFS\Components\Orpheus Sydney\Win32\hpp" -isystem "c:\program
files (x86)\embarcadero\studio\21.0\include\boost_1_70\\BOOST" -isystem D:\ICRFS\Components\SimpleGraph\hpp -isystem
C:\Users\Public\Documents\Embarcadero\Studio\21.0\hpp\Win32 -debug-info-kind=standalone -fborland-extensions -nobuiltininc -nostdsysteminc -triple
i686-pc-windows-omf -emit-obj -mrelocation-model static -masm-verbose -ffunction-sections -fexceptions -fcxx-exceptions -fseh -mstack-alignment=16
-fno-spell-checking -fno-use-cxa-atexit -fno-threadsafe-statics -main-file-name DFS.cpp -x c++ -std=c++17 -O0 -fmath-errno -tC -tM -o
.\Win32\Debug\DFS.obj --auto-dependency-output -MT .\Win32\Debug\DFS.obj -include-pch .\Win32\Debug\TreesPCH1.pch DFS.cpp
[bcc32c Error] depth_first_search.hpp(234): no member named 'initialize_vertex' in 'boost::bgl_named_params<cycle_detector, boost::graph_visitor_t, boost::no_property>'
DFS.cpp(62): in instantiation of function template specialization 'boost::depth_first_search<boost::adjacency_list<boost::setS, boost::vecS, boost::bidirectionalS, VertexProps, EdgeProps, boost::no_property, boost::listS>, boost::bgl_named_params<cycle_detector, boost::graph_visitor_t, boost::no_property>, boost::iterator_property_map<std::_Vector_iterator<std::_Vector_val<std::_Simple_types<boost::default_color_type> > >, boost::vec_adj_list_vertex_id_map<VertexProps, unsigned int>, boost::default_color_type, boost::default_color_type &> >' requested here
[bcc32c Error] depth_first_search.hpp(237): no member named 'start_vertex' in 'boost::bgl_named_params<cycle_detector, boost::graph_visitor_t, boost::no_property>'
[bcc32c Error] depth_first_search.hpp(245): no member named 'start_vertex' in 'boost::bgl_named_params<cycle_detector, boost::graph_visitor_t, boost::no_property>'
[bcc32c Error] depth_first_search.hpp(39): no member named 'initialize_vertex' in 'boost::bgl_named_params<cycle_detector, boost::graph_visitor_t, boost::no_property>'
general.hpp(47): in instantiation of member function 'boost::DFSVisitorConcept<boost::bgl_named_params<cycle_detector, boost::graph_visitor_t, boost::no_property>, boost::adjacency_list<boost::setS, boost::vecS, boost::bidirectionalS, VertexProps, EdgeProps, boost::no_property, boost::listS> >::constraints' requested here
depth_first_search.hpp(227): in instantiation of member function 'boost::concepts::constraint<boost::DFSVisitorConcept<boost::bgl_named_params<cycle_detector, boost::graph_visitor_t, boost::no_property>, boost::adjacency_list<boost::setS, boost::vecS, boost::bidirectionalS, VertexProps, EdgeProps, boost::no_property, boost::listS> > >::failed' requested here
DFS.cpp(62): in instantiation of function template specialization 'boost::depth_first_search<boost::adjacency_list<boost::setS, boost::vecS, boost::bidirectionalS, VertexProps, EdgeProps, boost::no_property, boost::listS>, boost::bgl_named_params<cycle_detector, boost::graph_visitor_t, boost::no_property>, boost::iterator_property_map<std::_Vector_iterator<std::_Vector_val<std::_Simple_types<boost::default_color_type> > >, boost::vec_adj_list_vertex_id_map<VertexProps, unsigned int>, boost::default_color_type, boost::default_color_type &> >' requested here
[bcc32c Error] depth_first_search.hpp(40): no member named 'start_vertex' in 'boost::bgl_named_params<cycle_detector, boost::graph_visitor_t, boost::no_property>'
[bcc32c Error] depth_first_search.hpp(41): no member named 'discover_vertex' in 'boost::bgl_named_params<cycle_detector, boost::graph_visitor_t, boost::no_property>'
[bcc32c Error] depth_first_search.hpp(42): no member named 'examine_edge' in 'boost::bgl_named_params<cycle_detector, boost::graph_visitor_t, boost::no_property>'
[bcc32c Error] depth_first_search.hpp(43): no member named 'tree_edge' in 'boost::bgl_named_params<cycle_detector, boost::graph_visitor_t, boost::no_property>'
[bcc32c Error] depth_first_search.hpp(44): no member named 'back_edge' in 'boost::bgl_named_params<cycle_detector, boost::graph_visitor_t, boost::no_property>'
[bcc32c Error] depth_first_search.hpp(45): no member named 'forward_or_cross_edge' in 'boost::bgl_named_params<cycle_detector, boost::graph_visitor_t, boost::no_property>'
[bcc32c Error] depth_first_search.hpp(47): no member named 'finish_vertex' in 'boost::bgl_named_params<cycle_detector, boost::graph_visitor_t, boost::no_property>'
[bcc32c Error] depth_first_search.hpp(134): no member named 'discover_vertex' in 'boost::bgl_named_params<cycle_detector, boost::graph_visitor_t, boost::no_property>'
depth_first_search.hpp(238): in instantiation of function template specialization 'boost::detail::depth_first_visit_impl<boost::adjacency_list<boost::setS, boost::vecS, boost::bidirectionalS, VertexProps, EdgeProps, boost::no_property, boost::listS>, boost::bgl_named_params<cycle_detector, boost::graph_visitor_t, boost::no_property>, boost::iterator_property_map<std::_Vector_iterator<std::_Vector_val<std::_Simple_types<boost::default_color_type> > >, boost::vec_adj_list_vertex_id_map<VertexProps, unsigned int>, boost::default_color_type, boost::default_color_type &>, boost::detail::nontruth2>' requested here
DFS.cpp(62): in instantiation of function template specialization 'boost::depth_first_search<boost::adjacency_list<boost::setS, boost::vecS, boost::bidirectionalS, VertexProps, EdgeProps, boost::no_property, boost::listS>, boost::bgl_named_params<cycle_detector, boost::graph_visitor_t, boost::no_property>, boost::iterator_property_map<std::_Vector_iterator<std::_Vector_val<std::_Simple_types<boost::default_color_type> > >, boost::vec_adj_list_vertex_id_map<VertexProps, unsigned int>, boost::default_color_type, boost::default_color_type &> >' requested here
[bcc32c Error] depth_first_search.hpp(155): no member named 'examine_edge' in 'boost::bgl_named_params<cycle_detector, boost::graph_visitor_t, boost::no_property>'
[bcc32c Error] depth_first_search.hpp(158): no member named 'tree_edge' in 'boost::bgl_named_params<cycle_detector, boost::graph_visitor_t, boost::no_property>'
[bcc32c Error] depth_first_search.hpp(163): no member named 'discover_vertex' in 'boost::bgl_named_params<cycle_detector, boost::graph_visitor_t, boost::no_property>'
[bcc32c Error] depth_first_search.hpp(179): no member named 'finish_vertex' in 'boost::bgl_named_params<cycle_detector, boost::graph_visitor_t, boost::no_property>'
Failed
Elapsed time: 00:00:02.8
任何幫助將非常感激
通過將訪問者包裝在boost::visitor
中,您會混淆命名參數重載。 只是通過訪客:
boost::depth_first_search(g, cycle_detector{has_cycle}, color_map, v0);
或者將所有 arguments 作為命名參數傳遞:
boost::depth_first_search(g,
boost::visitor(cycle_detector{has_cycle})
.color_map(color_map)
.root_vertex(v0));
#include <boost/graph/adjacency_list.hpp>
#include <boost/graph/breadth_first_search.hpp>
#include <boost/graph/depth_first_search.hpp>
#include <boost/graph/graph_traits.hpp>
#include <boost/graph/topological_sort.hpp>
#include <stdio.h>
#ifndef IHAVEASANECOMPILER_PLATFORM
#define _tmain main
#define _TCHAR char
#endif
struct EdgeProps {
int value;
};
struct VertexProps {
int value;
};
struct cycle_detector : boost::default_dfs_visitor {
cycle_detector(bool& has_cycle) : _has_cycle(has_cycle) {}
template <class Edge, class Graph> void back_edge(Edge, Graph&) {
_has_cycle = true;
}
bool& _has_cycle;
};
int _tmain() {
typedef boost::adjacency_list<boost::setS, boost::vecS,
boost::bidirectionalS, VertexProps, EdgeProps>
Graph;
Graph g;
auto v0 = boost::add_vertex(g);
auto v1 = boost::add_vertex(g);
auto v2 = boost::add_vertex(g);
auto v3 = boost::add_vertex(g);
auto v4 = boost::add_vertex(g);
boost::add_edge(v0, v1, g);
boost::add_edge(v0, v2, g);
boost::add_edge(v0, v3, g);
boost::add_edge(v2, v4, g);
boost::add_edge(v3, v4, g);
bool has_cycle = false;
std::vector<boost::default_color_type> colors(boost::num_vertices(g));
boost::iterator_property_map color_map(colors.begin(), boost::get(boost::vertex_index, g));
boost::depth_first_search(g, cycle_detector{has_cycle}, color_map, v0);
}
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.