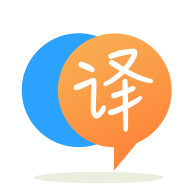
[英]java.lang.NoSuchMethodError: org.apache.commons.codec.binary.Base64.encodeBase64String
[英]No static method encodeBase64String([B)Ljava/lang/String; in class Lorg/apache/commons/codec/binary/Base64; or its super classes
實際上,我正在嘗試在我的 android 應用程序中添加 Aes 加密和解密功能。 在加密過程中,它會使我的 motoG5 S plus 設備崩潰,但它在我的 One Plus 設備中正常工作。
這是我的代碼:AesUtil.Java
public class AesUtil {
private int keySize = 256;
private int iterationCount = 1000 ;
private Cipher cipher;
private final String salt = "36e8fc9a6adf090665f459a7ad1b864d";
private final String iv = "ab00b7ea4e88500f2f0a17a7b5c7bcb1";
private final String passphrase = "ArknRQxD1YgaSFRHrjYazX7JMrlRxTERdkQx0dhENVlz";
public AesUtil() {
try {
cipher = Cipher.getInstance("AES/CBC/PKCS5Padding");
}
catch (NoSuchAlgorithmException | NoSuchPaddingException e) {
throw fail(e);
}
}
public String encrypt(String plaintext) {
try {
SecretKey key = generateKey(salt, passphrase);
byte[] encrypted = doFinal(Cipher.ENCRYPT_MODE, key, iv, plaintext.getBytes("UTF-8"));
return Base64.encodeBase64String(encrypted);
}
catch (UnsupportedEncodingException e) {
throw fail(e);
}
}
public String decrypt(String salt, String iv, String passphrase, String ciphertext) {
try {
SecretKey key = generateKey(salt, passphrase);
byte[] decrypted = doFinal(Cipher.DECRYPT_MODE, key, iv, base64(ciphertext));
return new String(decrypted, "UTF-8");
} catch (Exception e) {
throw fail(e);
}
}
private byte[] doFinal(int encryptMode, SecretKey key, String iv, byte[] bytes) {
try {
cipher.init(encryptMode, key, new IvParameterSpec(hex(iv)));
return cipher.doFinal(bytes);
} catch (InvalidKeyException
| InvalidAlgorithmParameterException
| IllegalBlockSizeException
| BadPaddingException e) {
throw fail(e);
}
}
public SecretKey generateKey(String salt, String passphrase) {
try {
SecretKeyFactory factory = SecretKeyFactory.getInstance("PBKDF2WithHmacSHA1");
KeySpec spec = new PBEKeySpec(passphrase.toCharArray(), hex(salt), iterationCount, keySize);
SecretKey key = new SecretKeySpec(factory.generateSecret(spec).getEncoded(), "AES");
return key;
}
catch (NoSuchAlgorithmException | InvalidKeySpecException e) {
return null;
}
}
public static byte[] base64(String str) {
return Base64.decodeBase64(str);
}
public static byte[] hex(String str) {
try {
return Hex.decodeHex(str.toCharArray());
}
catch (DecoderException e) {
throw new IllegalStateException(e);
}
}
private IllegalStateException fail(Exception e) {
e.printStackTrace();
return null;
} }
在我的主要活動中撥打 Function:
String encryptedText=AesUtil().encrypt("codeinsidecoffee")
Moto G5s Plus 內部的錯誤日志:
java.lang.NoSuchMethodError: 沒有 static 方法 encodeBase64String([B)Ljava/lang/String; 在 class Lorg/apache/commons/codec/binary/Base64 中; 或其超類('org.apache.commons.codec.binary.Base64' 的聲明出現在 /system/framework/org.apache.http.legacy.boot.jar 中)在 com.justcodenow.bynfor.utils.AesUtil.encrypt( AesUtil.java:40)
Method1:此方法自 Apache Commons Codec 版本 1.4 起可用。 如果手機操作系統只有舊版本的Codec package,則該方法不可用。 或者,我們可以使用舊版本中存在的方法。
代替:
String encodedString = Base64.encodeBase64String(bytes);
利用:
String encodedString = new String(Base64.encodeBase64(bytes));
而對於解碼,而不是:
byte[] bytes = Base64.decodeBase64(encodedString);
利用:
byte[] bytes = Base64.decodeBase64(encodedString.getBytes());
方法2:您也可以使用以下完整代碼進行加密和解密。
import android.util.Base64;
import org.apache.commons.codec.DecoderException;
import org.apache.commons.codec.binary.Hex;
import javax.crypto.*;
import javax.crypto.spec.IvParameterSpec;
import javax.crypto.spec.PBEKeySpec;
import javax.crypto.spec.SecretKeySpec;
import java.io.UnsupportedEncodingException;
import java.security.InvalidAlgorithmParameterException;
import java.security.InvalidKeyException;
import java.security.NoSuchAlgorithmException;
import java.security.spec.InvalidKeySpecException;
import java.security.spec.KeySpec;
public class AesUtil {
private int keySize = 256;
private int iterationCount = 1000 ;
private Cipher cipher;
private final String salt = "36e8fc9a6adf090665f459a7ad1b864d";
private final String iv = "ab00b7ea4e88500f2f0a17a7b5c7bcb1";
private final String passphrase = "ArknRQxD1YgaSFRHrjYazX7JMrlRxTERdkQx0dhENVlz";
public AesUtil() {
try {
cipher = Cipher.getInstance("AES/CBC/PKCS5Padding");
}
catch (NoSuchAlgorithmException | NoSuchPaddingException e) {
throw fail(e);
}
}
public String encrypt(String plaintext) {
try {
SecretKey key = generateKey(salt, passphrase);
byte[] encrypted = doFinal(Cipher.ENCRYPT_MODE, key, iv, plaintext.getBytes("UTF-8"));
return Base64.encodeToString(encrypted, Base64.DEFAULT);
}
catch (UnsupportedEncodingException e) {
throw fail(e);
}
}
public String decrypt(String salt, String iv, String passphrase, String ciphertext) {
try {
SecretKey key = generateKey(salt, passphrase);
byte[] decrypted = doFinal(Cipher.DECRYPT_MODE, key, iv, base64(ciphertext));
return new String(decrypted, "UTF-8");
} catch (Exception e) {
throw fail(e);
}
}
private byte[] doFinal(int encryptMode, SecretKey key, String iv, byte[] bytes) {
try {
cipher.init(encryptMode, key, new IvParameterSpec(hex(iv)));
return cipher.doFinal(bytes);
} catch (InvalidKeyException
| InvalidAlgorithmParameterException
| IllegalBlockSizeException
| BadPaddingException e) {
throw fail(e);
}
}
public SecretKey generateKey(String salt, String passphrase) {
try {
SecretKeyFactory factory = SecretKeyFactory.getInstance("PBKDF2WithHmacSHA1");
KeySpec spec = new PBEKeySpec(passphrase.toCharArray(), hex(salt), iterationCount, keySize);
SecretKey key = new SecretKeySpec(factory.generateSecret(spec).getEncoded(), "AES");
return key;
}
catch (NoSuchAlgorithmException | InvalidKeySpecException e) {
return null;
}
}
public static byte[] base64(String str) {
return Base64.decode(str, Base64.DEFAULT);
}
public static byte[] hex(String str) {
try {
return Hex.decodeHex(str.toCharArray());
}
catch (DecoderException e) {
throw fail(e);
}
}
private IllegalStateException fail(Exception e) {
e.printStackTrace();
return null;
}
private static IllegalStateException fail(DecoderException e) {
e.printStackTrace();
return null;
} }
如果有人正在研究 JWT,我建議閱讀此https://github.com/auth0/java-jwt/issues/259
有一個庫實際上支持 android https://github.com/auth0/JWTDecode.Android
經過這么多次重試。 我想將 sdk 中的所有邏輯復制粘貼到我的項目中。 但似乎只有一個 static function 在某些 android apache/commons 框架中不可用。 這是Base64.encodeBase64String()
所以我所做的是,我在我的項目中從庫中復制了一個 function,並且來自庫的遞歸 function 調用存在於舊框架中。
因此,不要使用Base64.encodeBase64String()在您的文件中復制此 function 並使用它。 這將同樣有效。
fun encodeBase64String(binaryData: ByteArray?): String? {
return StringUtils.newStringUsAscii(
org.apache.commons.codec.binary.Base64.encodeBase64(
binaryData,
false
)
)
}
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.