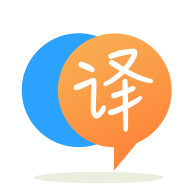
[英]calculate the max length of an array such that average is less than given value
[英]How to add, calculate and filter those elements that are less than average without using repetitive cycles
我正在使用( List
)java 結構:
var nums = List.of( 3, 9, 7, 12, 20, 4, 11, 9, 21, 6, 8, 10 );
通過這種使用,我正在執行以下程序,而無需使用重復循環:
package averagesumwithoutcycles;
import java.util.*;
import java.util.List;
public class SumaSinCiclos {
private static int suma = 0;
public static void main(String[] args) {
int[] nums = {3, 9, 7, 12, 20, 4, 11, 9, 21, 6, 8, 10};
System.out.println(average(nums, 0));
}
public static float average(int n[], int pos){
float resultado = (float)suma /(float)n.length;
if(pos < n.length) {
suma = suma + n[pos];
average(n, pos + 1);
System.out.println(resultado);
}
return resultado;
}
}
到目前為止,我已經實現了以下目標:
我的問題如下,根據我的代碼,我如何對元素( array
)求和,計算平均值,並過濾那些小於平均值的元素。
注意:在不使用重復循環的情況下獲取此數據很重要
這是我認為最好的答案。 它確實在內部使用循環,但任何可能的獲取數組總和的解決方案都需要循環(迭代或遞歸)。
import java.util.Arrays;
import java.util.stream.IntStream;
class Main {
private static int suma = 0;
public static void main(String[] args) {
int[] nums = {3, 9, 7, 12, 20, 4, 11, 9, 21, 6, 8, 10};
for (int num : nums) {
System.out.println(num);
}
nums = filter(nums, average(nums));
System.out.println("____________\n");
for (int num : nums) {
System.out.println(num);
}
}
public static float average(int[] n){
float suma = IntStream.of(n).sum();
return suma / n.length;
}
public static int[] filter(int[] nums, float average) {
return Arrays.stream(nums).filter(x -> x >= average).toArray();
}
}
你總是可以做這樣的事情(但這很麻煩)。
int[] vals = {10,2,8};
int sum = 0;
sum += vals[0];
sum += vals[1];
sum += vals[2];
double avg = (double)sum/vals.length;
List<Integer> lessThan = new ArrayList<>();
if (vals[0] < avg) {
lessThan.add(vals[0]);
}
if (vals[1] < avg) {
lessThan.add(vals[1]);
}
if (vals[2] < avg) {
lessThan.add(vals[2]);
}
System.out.println(lessThan); // prints 2
var nums = List.of( 3, 9, 7, 12, 20, 4, 11, 9, 21, 6, 8, 10 );
int sum = 0;
for (int v : nums) {
sum+=v;
}
double avg = (double)sum/v.size();
一旦你有了平均值,你就可以找到更小的平均值,如下所示。
vars lessThan = new ArrayList<>();
for (int v : nums) {
// store the numbers that are less than avg in the list.
}
Then print them here.
也許我遺漏了一些東西,但看起來你解決了這個問題。 在您的代碼中,您有元素的總和和平均值,並且您沒有使用顯式循環。
唯一的問題是那些小於平均值的元素。 一個可能的解決方案可能是從您完成第一次遞歸的那一刻起使用專用的遞歸 function(傳遞所有元素,並在遞歸時逐個檢查它們與平均值 [你已經擁有])。
也許是這樣的:
public static void main(String[] args) {
int[] nums = {3, 9, 7, 12, 20, 4, 11, 9, 21, 6, 8, 10};
System.out.println(average(nums, 0, nums)); // Added "nums" again
}
public static float average(int n[], int pos, int originalElements[]){
float resultado = (float)suma /(float)n.length;
if(pos < n.length) {
suma = suma + n[pos];
average(n, pos + 1, originalElements);
System.out.println(resultado);
} else {
// you will get hete only ONCE, in case pos = n.length
// note that in this state, "resultado" contains the original array's average
checkSmallThanAverage(resultado, 0, originalElements);
}
return resultado;
}
public static void checkSmallThanAverage(float resultado, int pos, int originalElements[]) {
if(pos < originalElements.length) {
if (originalElements[pos] < resultado) {
System.out.println("element " + originalElements[pos] + " is smaller than average");
}
checkSmallThanAverage(resultado, pos+1, originalElements);
}
}
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.