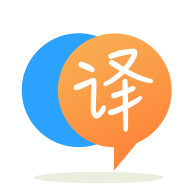
[英]WPF, C#, Ink Canvas, Image - How to save inkcanvas on existing image and save it as new image?
[英]how to save work done on canvas using InkCanvas to an image file in UWP C#?
我想在我的 UWP 應用程序中保存我在 canvas 上完成的工作。 我正在使用 InkCanvas 在 canvas 內的選定圖像上繪制線條,並且我想將 canvas 工作保存到新的圖像文件中。
嘗試保存文件后,我得到一個空白圖像。 我嘗試了兩種保存文件的方法。
完成工作:
xaml代碼
<Button Click="ShowPopup" Content="click me"/>
<Popup x:Name="IMG_G" Width="600" Height="300" HorizontalAlignment="Left" ManipulationMode="All">
<Grid x:Name="img_grid" Height="300" Background="{ThemeResource ApplicationPageBackgroundThemeBrush}" ManipulationMode="Scale">
<Image VerticalAlignment="Top" HorizontalAlignment="Left"
x:Name="img" Stretch="Fill" Height="300" ManipulationMode="All">
</Image>
<Canvas x:Name="selectionCanvas" Width="600" Background="Transparent" Height="300"/>
<InkCanvas x:Name="inker" />
<InkToolbar x:Name="img_inktoolbar" TargetInkCanvas="{x:Bind inker}"
VerticalAlignment="Top">
</InkToolbar>
</Grid>
</Popup>
<Button Content="Save"
Width="100"
Height="25"
HorizontalAlignment="Center"
VerticalAlignment="Center" Click="BtnSave_Click"/>
代碼背后
public DrawLines()
{
InitializeComponent();
inker.InkPresenter.InputDeviceTypes = CoreInputDeviceTypes.Mouse | CoreInputDeviceTypes.Touch;
inker.InkPresenter.UnprocessedInput.PointerPressed += StartLine;
inker.InkPresenter.UnprocessedInput.PointerMoved += ContinueLine;
inker.InkPresenter.UnprocessedInput.PointerReleased += CompleteLine;
inker.InkPresenter.InputProcessingConfiguration.RightDragAction = InkInputRightDragAction.LeaveUnprocessed;
}
private async void ShowPopup(object sender, RoutedEventArgs e)
{
var _filePicker = new FileOpenPicker();
_filePicker.SuggestedStartLocation = PickerLocationId.Desktop;
_filePicker.ViewMode = PickerViewMode.Thumbnail;
_filePicker.FileTypeFilter.Add(".bmp");
_filePicker.FileTypeFilter.Add(".jpg");
StorageFile _file = await _filePicker.PickSingleFileAsync();
IRandomAccessStream imageStream = await _file.OpenAsync(FileAccessMode.Read);
BitmapImage bmpimage = new BitmapImage();
await bmpimage.SetSourceAsync(imageStream);
img.Source = bmpimage;
IMG_G.IsOpen = true;
}
private void StartLine(InkUnprocessedInput sender, PointerEventArgs args)
{
line = new Line();
line.X1 = args.CurrentPoint.RawPosition.X;
line.Y1 = args.CurrentPoint.RawPosition.Y;
line.X2 = args.CurrentPoint.RawPosition.X;
line.Y2 = args.CurrentPoint.RawPosition.Y;
line.Stroke = new SolidColorBrush(Colors.Purple);
line.StrokeThickness = 4;
selectionCanvas.Children.Add(line);
}
private void ContinueLine(InkUnprocessedInput sender, PointerEventArgs args)
{
line.X2 = args.CurrentPoint.RawPosition.X;
line.Y2 = args.CurrentPoint.RawPosition.Y;
}
private void CompleteLine(InkUnprocessedInput sender, PointerEventArgs args)
{
List<InkPoint> points = new List<InkPoint>();
InkStrokeBuilder builder = new InkStrokeBuilder();
InkPoint pointOne = new InkPoint(new Point(line.X1, line.Y1), 0.5f);
points.Add(pointOne);
InkPoint pointTwo = new InkPoint(new Point(line.X2, line.Y2), 0.5f);
points.Add(pointTwo);
InkStroke stroke = builder.CreateStrokeFromInkPoints(points, System.Numerics.Matrix3x2.Identity);
InkDrawingAttributes ida = inker.InkPresenter.CopyDefaultDrawingAttributes();
stroke.DrawingAttributes = ida;
inker.InkPresenter.StrokeContainer.AddStroke(stroke);
selectionCanvas.Children.Remove(line);
}
保存文件的方法1
private async void BtnSave_Click(object sender, RoutedEventArgs e)
{
StorageFolder pictureFolder = KnownFolders.SavedPictures;
var file = await pictureFolder.CreateFileAsync("test2.bmp", CreationCollisionOption.ReplaceExisting);
CanvasDevice device = CanvasDevice.GetSharedDevice();
CanvasRenderTarget renderTarget = new CanvasRenderTarget(device, (int)img.ActualWidth, (int)img.ActualHeight, 96);
//get image's path
StorageFolder folder = ApplicationData.Current.LocalFolder;
//Get the same image file copy which i selected to draw on in ShowPopup() but I actually wanted to get the edited canvas
StorageFile Ifile = await folder.GetFileAsync("Datalog_2020_09_22_10_44_59_2_3_5_RSF.bmp");
var inputFile = Ifile.Path;
using (var ds = renderTarget.CreateDrawingSession())
{
ds.Clear(Colors.White);
CanvasBitmap image = await CanvasBitmap.LoadAsync(device, inputFile);
//var image = img2.Source;
// I want to use this too, but I have no idea about this
ds.DrawImage(image);
ds.DrawInk(inker.InkPresenter.StrokeContainer.GetStrokes());
}
// save results
using (var fileStream = await file.OpenAsync(FileAccessMode.ReadWrite))
{
await renderTarget.SaveAsync(fileStream, CanvasBitmapFileFormat.Jpeg, 1f);
}
}
結果
期望的結果
結果我用這種方法得到
test2.bmp(圖像由於某種原因被放大)
保存文件的方法2
private async void BtnSave_Click(object sender, RoutedEventArgs e)
{
RenderTargetBitmap bitmap = new RenderTargetBitmap();
await bitmap.RenderAsync(selectionCanvas);
Debug.WriteLine($"Capacity = {(uint)bitmap.PixelWidth}, Length={(uint)bitmap.PixelHeight}");
var pixelBuffer = await bitmap.GetPixelsAsync();
Debug.WriteLine($"Capacity = {pixelBuffer.Capacity}, Length={pixelBuffer.Length}");
byte[] pixels = pixelBuffer.ToArray();
var displayInformation = DisplayInformation.GetForCurrentView();
StorageFolder pictureFolder = KnownFolders.SavedPictures;
var file = await pictureFolder.CreateFileAsync("test2.bmp", CreationCollisionOption.ReplaceExisting);
using (var stream = await file.OpenAsync(FileAccessMode.ReadWrite))
{
var encoder = await BitmapEncoder.CreateAsync(BitmapEncoder.BmpEncoderId, stream);
encoder.SetPixelData(BitmapPixelFormat.Bgra8,
BitmapAlphaMode.Ignore,
(uint)bitmap.PixelWidth,
(uint)bitmap.PixelHeight,
displayInformation.RawDpiX,
displayInformation.RawDpiY,
pixels);
await encoder.FlushAsync();
}
}
這種方法的結果
出於某種原因,我得到全黑圖像
測試2.bmp
任何幫助將不勝感激。 方法2的任何幫助都會更好。
如何使用 InkCanvas 將 canvas 上完成的工作保存到 UWP C# 中的圖像文件中?
您無需使用RenderTargetBitmap
將 InkCanvas 保存到圖像。 UWP InkCanvas
具有 SaveAsync 方法,可以將StrokeContainer
stream 直接保存到圖像文件中。 例如。
async void OnSaveAsync(object sender, RoutedEventArgs e)
{
// We don't want to save an empty file
if (inkCanvas.InkPresenter.StrokeContainer.GetStrokes().Count > 0)
{
var savePicker = new FileSavePicker();
savePicker.SuggestedStartLocation = PickerLocationId.PicturesLibrary;
savePicker.FileTypeChoices.Add("png with embedded ISF", new[] { ".png" });
StorageFile file = await savePicker.PickSaveFileAsync();
if (null != file)
{
try
{
using (IRandomAccessStream stream = await file.OpenAsync(FileAccessMode.ReadWrite))
{
// Truncate any existing stream in case the new file
// is smaller than the old file.
stream.Size = 0;
await inkCanvas.InkPresenter.StrokeContainer.SaveAsync(stream);
}
}
catch (Exception ex)
{
}
}
}
else
{
}
}
更多詳情請參考 UWP 簡單墨水代碼示例場景3
更新
嘗試保存文件后,我得到一個空白圖像。
上面的代碼只能保存InkCanvas
筆畫,我檢查了你的代碼,我發現你沒有在selectionCanvas
中放置任何元素。 所以selectionCanvas
的RenderTargetBitmap
將變黑為空。 請嘗試使用img_grid
替換。
嘿抱歉,但現在 InkToolbar 也與我所做的墨水更改一起被復制到圖像上:(
這是設計使然, RenderTargetBitmap
將渲染所有查看過的元素,對於您的場景,我們建議您制作矩形覆蓋InkToolbar
或將 img_inktoolbar Visibility 設置為 Collapsed,然后再捕獲屏幕並在完成后重置它。
我對方法 2 進行了一些更改並得到了預期的結果
保存文件的方法2
private async void BtnSave_Click(object sender, RoutedEventArgs e)
{
// In order to hide the InkToolbar before the saving the image
img_inktoolbar.Visibility = Visibility.Collapsed;
RenderTargetBitmap bitmap = new RenderTargetBitmap();
await bitmap.RenderAsync(img_grid);
Debug.WriteLine($"Capacity = {(uint)bitmap.PixelWidth}, Length={(uint)bitmap.PixelHeight}");
var pixelBuffer = await bitmap.GetPixelsAsync();
Debug.WriteLine($"Capacity = {pixelBuffer.Capacity}, Length={pixelBuffer.Length}");
byte[] pixels = pixelBuffer.ToArray();
var displayInformation = DisplayInformation.GetForCurrentView();
StorageFolder pictureFolder = KnownFolders.SavedPictures;
var file = await pictureFolder.CreateFileAsync("test2.bmp", CreationCollisionOption.ReplaceExisting);
using (var stream = await file.OpenAsync(FileAccessMode.ReadWrite))
{
var encoder = await BitmapEncoder.CreateAsync(BitmapEncoder.BmpEncoderId, stream);
encoder.SetPixelData(BitmapPixelFormat.Bgra8,
BitmapAlphaMode.Ignore,
(uint)bitmap.PixelWidth,
(uint)bitmap.PixelHeight,
displayInformation.RawDpiX,
displayInformation.RawDpiY,
pixels);
await encoder.FlushAsync();
}
}
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.