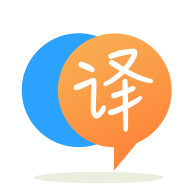
[英]How to set text of multiple buttons using a for loop and the contents of an ArrayList - Java - Android
[英]Set multiple buttons text in android
我在android中寫了一個問答游戲。 我希望每次游戲進入下一個問題時,所有按鈕文本也會隨着 string.xml 中存在的每個字符串而更新。 我的 string.xml 文件包含每個問題的<string>
值,如下所示:
<!-- Second question-->
<string name="secondQ_A1">Oracle</string>
<string name="secondQ_A2">Apple</string>
<string name="secondQ_A3">Sun Microsystems</string> <!-- Right answer -->
<string name="secondQ_A4">IBM</string>
這是代碼:
private Question[] questions = new Question[] {
new Question(R.drawable.first, R.string.first_question_text, R.string.firstQ_A2),
new Question(R.drawable.second, R.string.second_question_text, R.string.secondQ_A3),
new Question(R.drawable.third, R.string.third_question_text, R.string.thirdQ_A2),
};
private ImageView questionImage;
private TextView questionText;
private Button[] answerButtons;
private int currentQuestion = 0;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_game_play);
//Initializing
initializeFields();
int textQ = questions[currentQuestion].getResQuestionText();
questionText.setText(textQ);
int imageQ = questions[currentQuestion].getResQuestionImg();
questionImage.setImageResource(imageQ);
for (Button button : answerButtons) {
button.setOnClickListener(v -> {
if (currentQuestion + 1 < questions.length) {
currentQuestion += 1;
updateQuestion();
}
});
}
}
public void updateQuestion() {
int textQ = questions[currentQuestion].getResQuestionText();
questionText.setText(textQ);
int imageQ = questions[currentQuestion].getResQuestionImg();
questionImage.setImageResource(imageQ);
List<Integer> firstQ_answers = new LinkedList<>();
firstQ_answers.add(R.string.firstQ_A1);
firstQ_answers.add(R.string.firstQ_A2);
firstQ_answers.add(R.string.firstQ_A3);
firstQ_answers.add(R.string.firstQ_A4);
for (int i = 0; i< answerButtons.length; i++) {
questions[currentQuestion].setOptions(firstQ_answers);
answerButtons[i].setText(questions[currentQuestion].getOptions().get(i));
}
}
還有Question
class:
public class Question {
private int resQuestionText;
private int resQuestionImg;
private int resAnswer;
public Question(int resQuestionImg, int resQuestionText, int resAnswer) {
this.resQuestionImg = resQuestionImg;
this.resQuestionText = resQuestionText;
this.resAnswer = resAnswer;
}
public int getResQuestionText() {
return resQuestionText;
}
public int getResQuestionImg() {
return resQuestionImg;
}
public int getResAnswer() {
return resAnswer;
}
}
updateQuestion()
方法僅適用於問題的圖像和文本,但按鈕沒有文本。 我想用上面的 string.xml 文件值更新按鈕文本。 其實我不知道,誰能指導我?
從你的代碼中,你甚至沒有更新它,所以你必須在你的updateQueation()
方法中做這樣的事情:
answerButtons[0].setText(getString(R.string.secondQ_A1));
answerButtons[1].setText(getString(R.string.secondQ_A2));
answerButtons[2].setText(getString(R.string.secondQ_A3));
answerButtons[3].setText(getString(R.string.secondQ_A4));
作為最佳實踐,您應該定義一個全局二維數組來保存所有問題索引及其選項,例如:
int[][] myOptions ={{allFirstQuestionAnswersResHere},{second},{third},...};
然后在 updateQuestion() 中使用問題編號從全局數組中獲取選項,例如:
for(int i=0;i<answerButtons.length();i++){
answerButtons[i].setText(myOptions[questionIndex][i]);
}
享受 !
您在問題中使用 int 作為文本,這就是為什么不能這樣設置
questionText.setText(" "+textQ);
或將 int questionText 更改為 String questionText。
你可以通過改變你的問題 class 來達到同樣的目的
public class Question {
private int resQuestionText;
private int resQuestionImg;
private int resAnswer[];
public Question(int resQuestionImg, int resQuestionText, int resAnswer[]) {
this.resQuestionImg = resQuestionImg;
this.resQuestionText = resQuestionText;
this.resAnswer = resAnswer;
}
public int getResQuestionText() {
return resQuestionText;
}
public int getResQuestionImg() {
return resQuestionImg;
}
public int [] getResAnswer() {
return resAnswer;
}
}
然后在你的 updateQuestion 方法中
public void updateQuestion() {
// you can get array of button text for each question like below and can update your buttons text by iterating through this array
int buttonTexts[] = questions[currentQuestion].getResAnswer();
int textQ = questions[currentQuestion].getResQuestionText();
questionText.setText(textQ);
int imageQ = questions[currentQuestion].getResQuestionImg();
questionImage.setImageResource(imageQ);
}
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.